Java Reference
In-Depth Information
Notice that the anonymous inner class has access to the fi eld
x
and also the
final
local
variable
s
. Study the code and try to determine its output.
1. public class AnonInner {
2. public int x = 10;
3.
4. public void printX() {
5. final String s = “x = “;
6. Thread t = new Thread() {
7. public void run() {
8. while(true) {
9. System.out.println(s + x);
10. }
11. }
12. };
13. t.start();
14. }
15.
16. public static void main(String [] args) {
17. new AnonInner().printX();
18. }
19.}
You might not be familiar with threads in Java, but invoking
start
on a
Thread
object
causes its
run
method to execute in a new thread of the process. Here is the sequence of
events that occurs within
main
:
1.
A new outer object is instantiated on line 17 and its
printX
method on line 4 is
invoked.
2.
An anonymous inner class that extends
Thread
is declared and instantiated on
lines 6 to 12.
3.
The
start
method is invoked on this
Thread
object on line 13, which causes the
run
method on line 7 to execute.
4.
The
run
method contains an infinite loop and prints out
“x=10”
until the JVM is
terminated manually (press Ctrl+C in Windows).
Because the anonymous inner class does not have a name, the compiler assigns it a
number. When
AnonInner
is compiled, two bytecode fi les are generated:
AnonInner.class
and
AnonInner$1.class
.
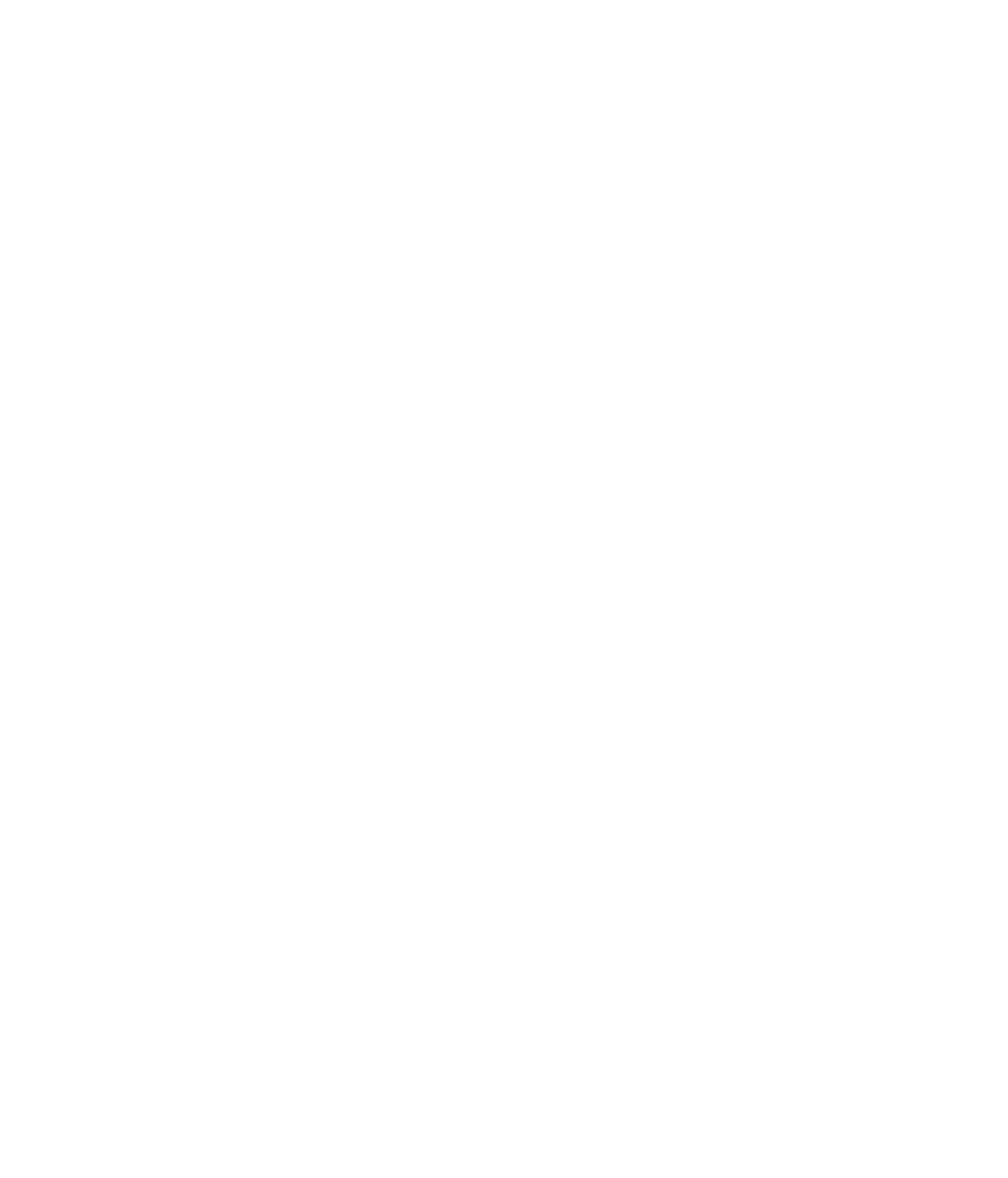




Search WWH ::

Custom Search