Java Reference
In-Depth Information
The child method cannot throw a greater exception than the parent. In other words,
any exception thrown by the child method must be a subclass of one of the exceptions
thrown by the parent method.
The return type of the method in the child class has to be the same or a subclass of the
parent method's return type.
Private Methods and Overriding
Method overriding refers to a child class overriding an instance method that it inherits
from its parent. A
private
method is not accessible outside of the class it is defi ned
in, and
private
methods are not inherited by child classes. Therefore, any discussion
on method overriding implies we are talking about the nonprivate instance methods of
a class. (We discuss overriding nonprivate static methods in the upcoming section on
method hiding.)
The following example demonstrates a child class
Lion
overriding the
eat
method in its
parent class
Mammal
:
//Mammal.java
public class Mammal {
protected int eat(String something) {
System.out.println(“Inside Mammal”);
return -1;
}
}
//Lion.java
public class Lion extends Mammal {
public int eat(String something) {
. System.out.println(“Inside Lion”);
. return something.length();
. }
}
Notice the
eat
method in
Lion
has the same name and parameter type as
eat
in
Mammal
.
The
eat
method is
protected
in
Mammal
and
public
in
Lion
, which is valid because
public
is more accessible than
protected
, so
Lion
successfully overrides the
eat
method in
Mammal
.
What is the output of the following statements?
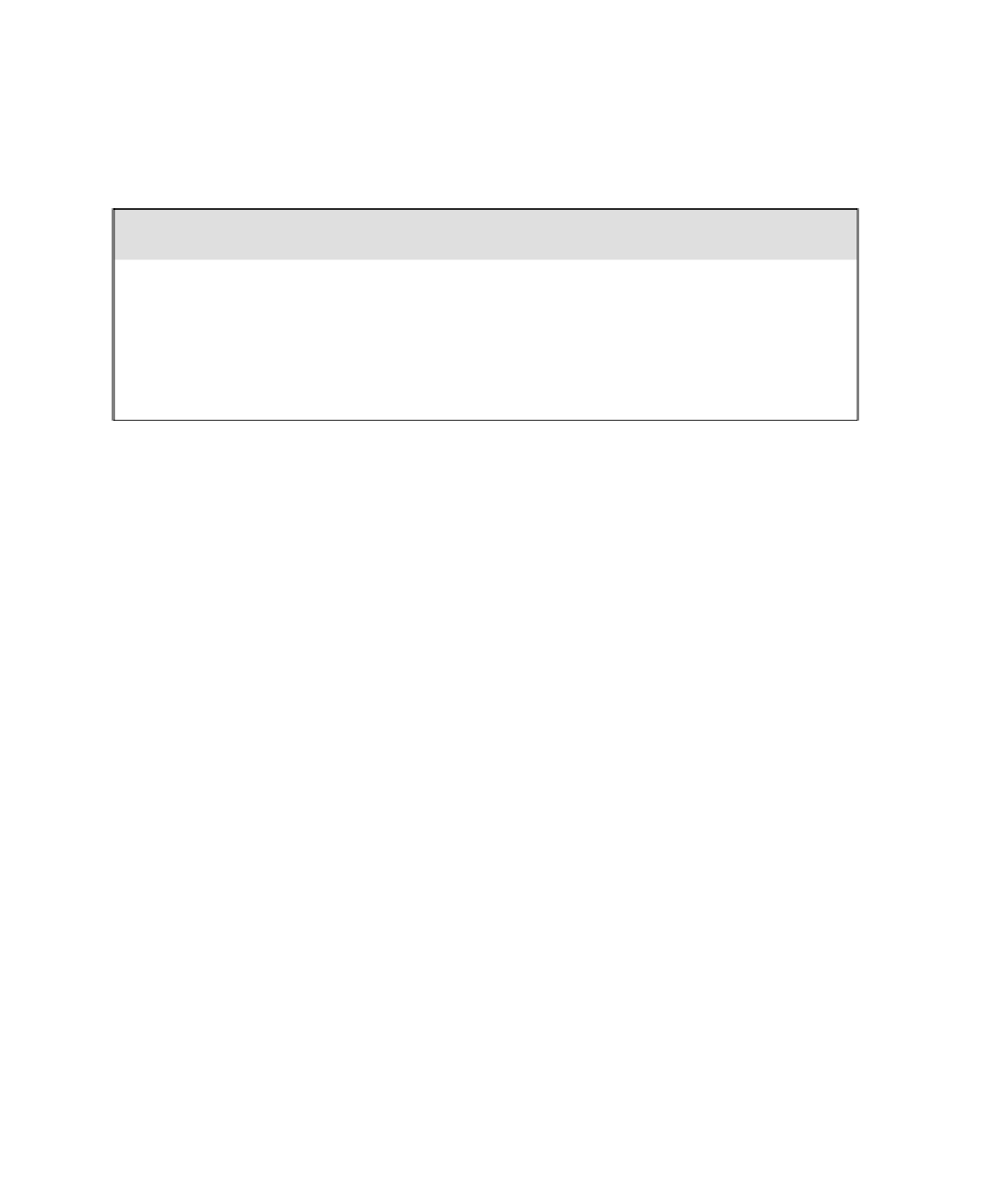




Search WWH ::

Custom Search