Java Reference
In-Depth Information
Methods Behind the Scenes
From an object-oriented point of view, each instance of a class gets each fi eld and
method of the class in memory when the object is instantiated. For fi elds, this is exactly
what happens on the heap. Every object must have its own memory for each nonstatic
fi eld of the class because the values of the fi elds are unique for each object.
However, from a practical point of view, each object does not need its own copy of
the methods because methods do not have any state and the implementation of each
method is the exact same for every instance. To save memory, the JVM instead stores the
method implementations in the
Class
object of the class, and each object accesses these
implementations by storing a corresponding function pointer for each method in the
class. In other words, instance methods are actually shared among all instances.
However, it is important to understand that from a theoretical point of view every object
has its own copy of each fi eld and each method in memory. If no
Customer
objects exist
in memory, then neither do any fi elds or methods of the
Customer
class. If there are
100
Customer
objects in memory, then there are 100
name
references and 100
ints
named
id
. In theory, there are also 100
setName
methods, 100
getName
methods, 100
processOrder
methods, and so on.
These behind-the-scenes details of how Java stores instance methods in the
Class
object
to save memory are not a topic on the SCJP exam.
Static Methods
A
static method
, also referred to as a
class method
, is declared using the
static
keyword. A static method is just like a static fi eld in that it belongs to the class, not the
instances. A static method is invoked without any instances of the class. Instead, use
the name of the class to invoke one of its static methods.
For example, the
java.lang.Math
class has a static method named
sqrt
that computes
the square root of a
double
:
public static double sqrt(double a)
To invoke
sqrt
, you prefi x it with the class name
Math
:
double x = 49.0;
double response = Math.sqrt(x);
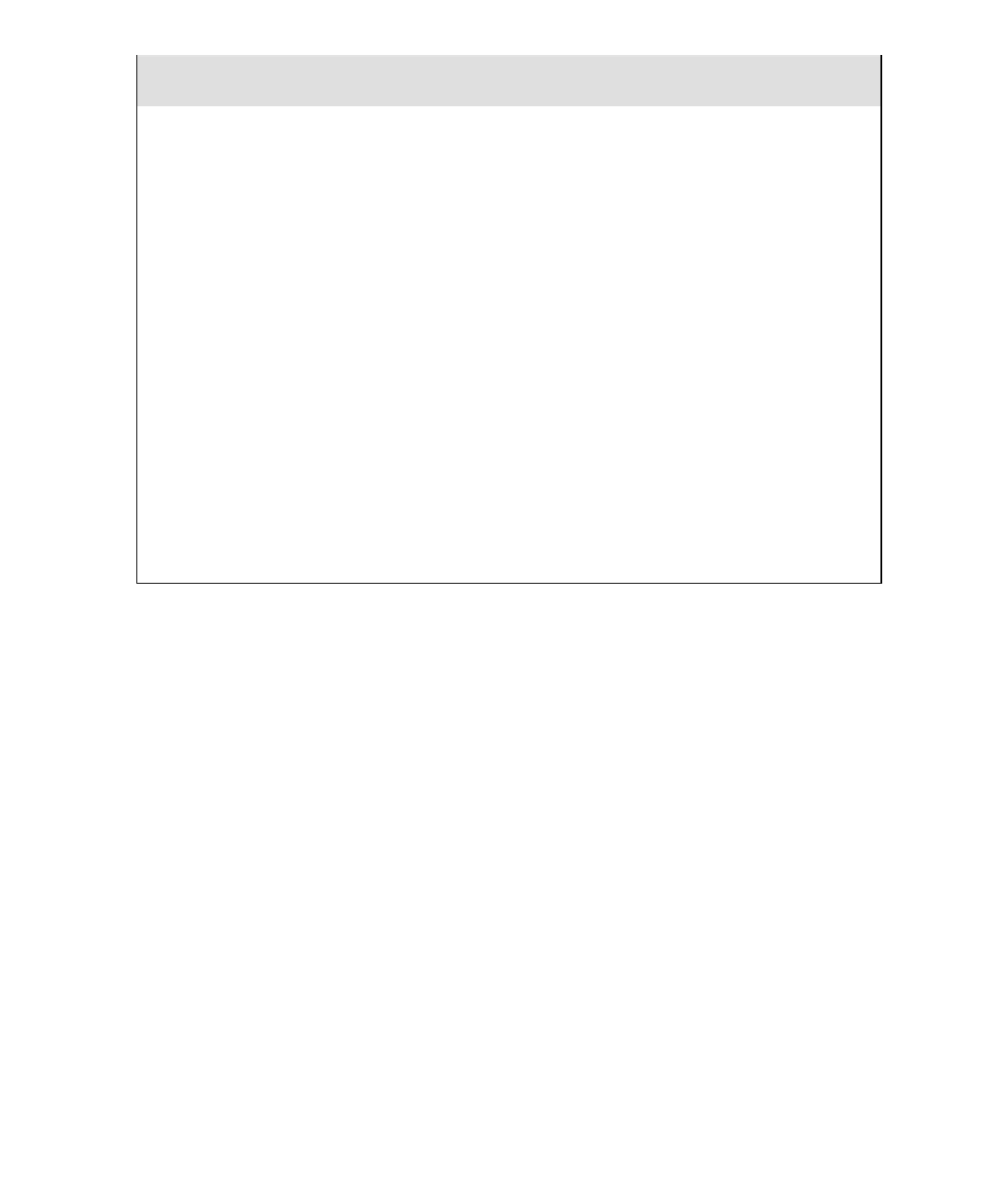




Search WWH ::

Custom Search