Java Reference
In-Depth Information
Why does the no-argument constructor get invoked on
Book
within the
NonFictionBook
constructor declared on line 10? The instantiation process requires that the parent class
constructor execute before any child class constructor executes. There are two important
rules of using
super
in a constructor that enforce this behavior:
Any call to
super
must be the first line of code in a constructor or the code will not
compile.
If a constructor does not explicitly have a call to
super
or
this
as its first line of code,
the compiler inserts the statement
super();
as the first line of code in the constructor.
In other words, if you write a constructor and do not call
super
, the compiler does it for
you. In the
NonFictionBook
constructor on line 10, the constructor actually looks like the
following:
public NonFictionBook(String subject) {
super(); //Compiler adds this statement
this.subject = subject;
}
If you want, you can explicitly add the call to
super
to make your code more readable.
The behavior of your code does not change by adding
super();
because the compiler
adds it for you anyway. With the call to
super
explicitly declared, it becomes clear which
constructor in the parent is being invoked.
Default Constructors and
super
Watch out on the exam for a question that tests your knowledge of the default call to
super
. It is an important concept in Java and this default line of code (that you don't
even write) generates a compiler error if the parent class does not have a no-argument
constructor. For example, suppose we have the following
Book
class:
public class Book {
public String ISBN;
public Book(String ISBN) {
this.ISBN = ISBN;
}
}
This version of
Book
only has one constructor. A
String
must be passed into any new
Book
being instantiated. (Recall that you do not get a default constructor in a class that
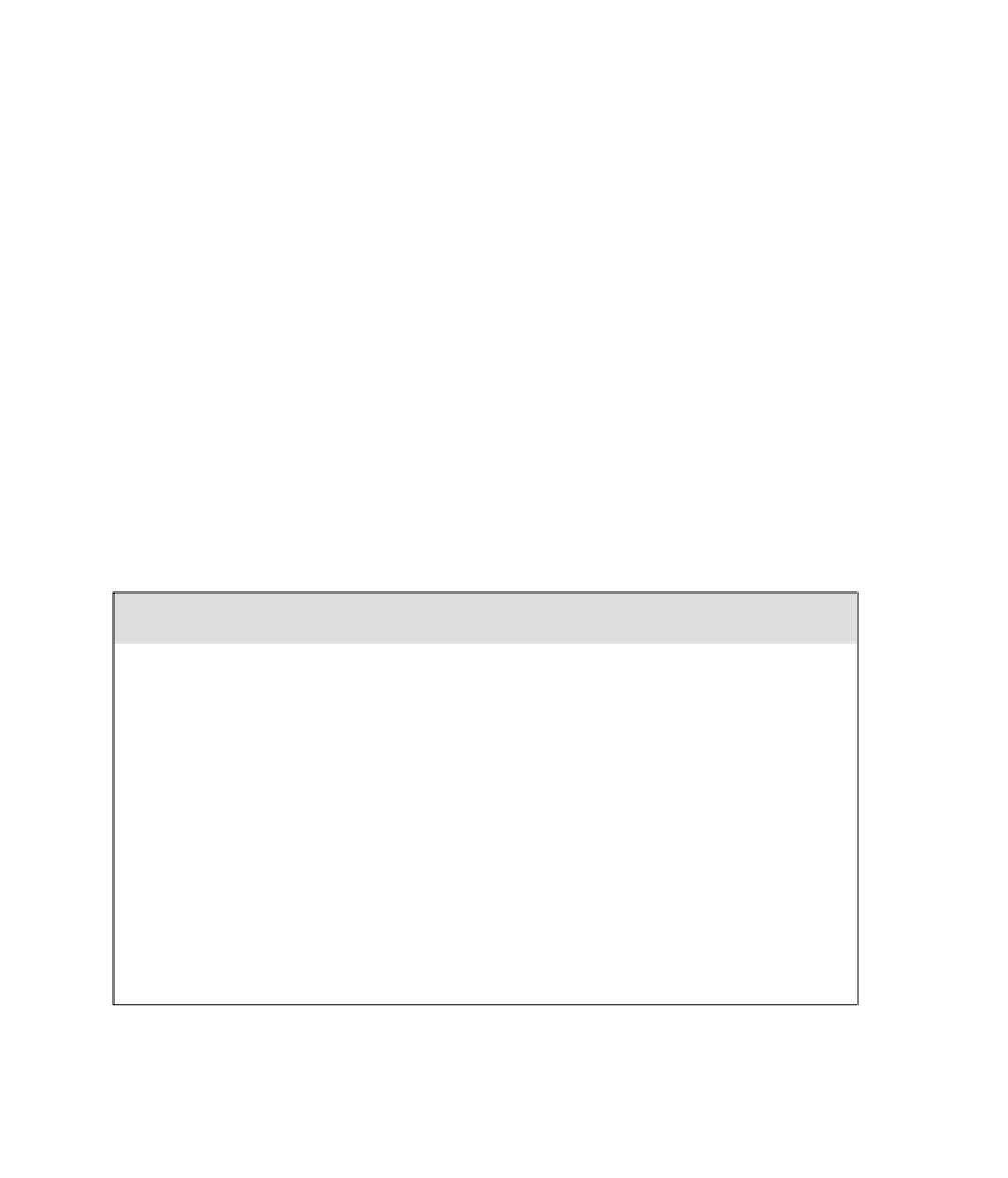




Search WWH ::

Custom Search