Java Reference
In-Depth Information
18. System.out.println(“The variety is “ + variety);
19. }
20.
21. public static void main(String [] args) {
22. Apple apple = new Apple(“Granny Smith”);
23. System.out.println(“Variety is “ + apple.variety);
24. }
25.}
The
main
method instantiates a new
Apple
object, passing in
“Granny Smith”
. The JVM
allocates memory for an
Apple
(which includes the memory for the
Fruit
parent object)
and zeroes the memory. Then explicit initialization occurs, which in this example assigns
variety
to
“McIntosh”
on line 9. Then the
Apple
constructor on line 11 is invoked, but
before it executes the
Fruit
constructor on line 4 is invoked and executes. After the
Fruit
constructor completes, the instance initializer on lines 16 to 19 is invoked, then the body of
the
Apple
constructor on line 11 executes. The output of running
main
looks like this:
Constructing a Fruit
Inside the instance initializer
The variety is McIntosh
Constructing an Apple
Variety is Granny Smith
Now that you have seen the order of events that occur when a new object is instantiated,
we will next look at the details of declaring and using constructors in Java.
Constructors
The exam objectives state that “given a set of classes and superclasses,” you should be
able to “develop constructors for one or more of the classes. Given a class declaration,
determine if a default constructor will be created, and if so, determine the behavior of that
constructor.” This section discusses these topics in detail.
A
constructor
is a special method within a class that gets invoked during the
instantiation process. The purpose of a constructor is to allow you to “construct” your
object, ensuring that all of the fi elds are properly initialized. Constructors also can take in
arguments, allowing you to initialize the state of the object.
A constructor has the following properties:
The name of a constructor must match the name of the class.
A constructor does not declare a return value.
A constructor is only invoked one time during the instantiation process.
A constructor can have any of four levels of access:
public
,
private
,
protected
, or the
default.
A constructor can throw any number of exceptions.
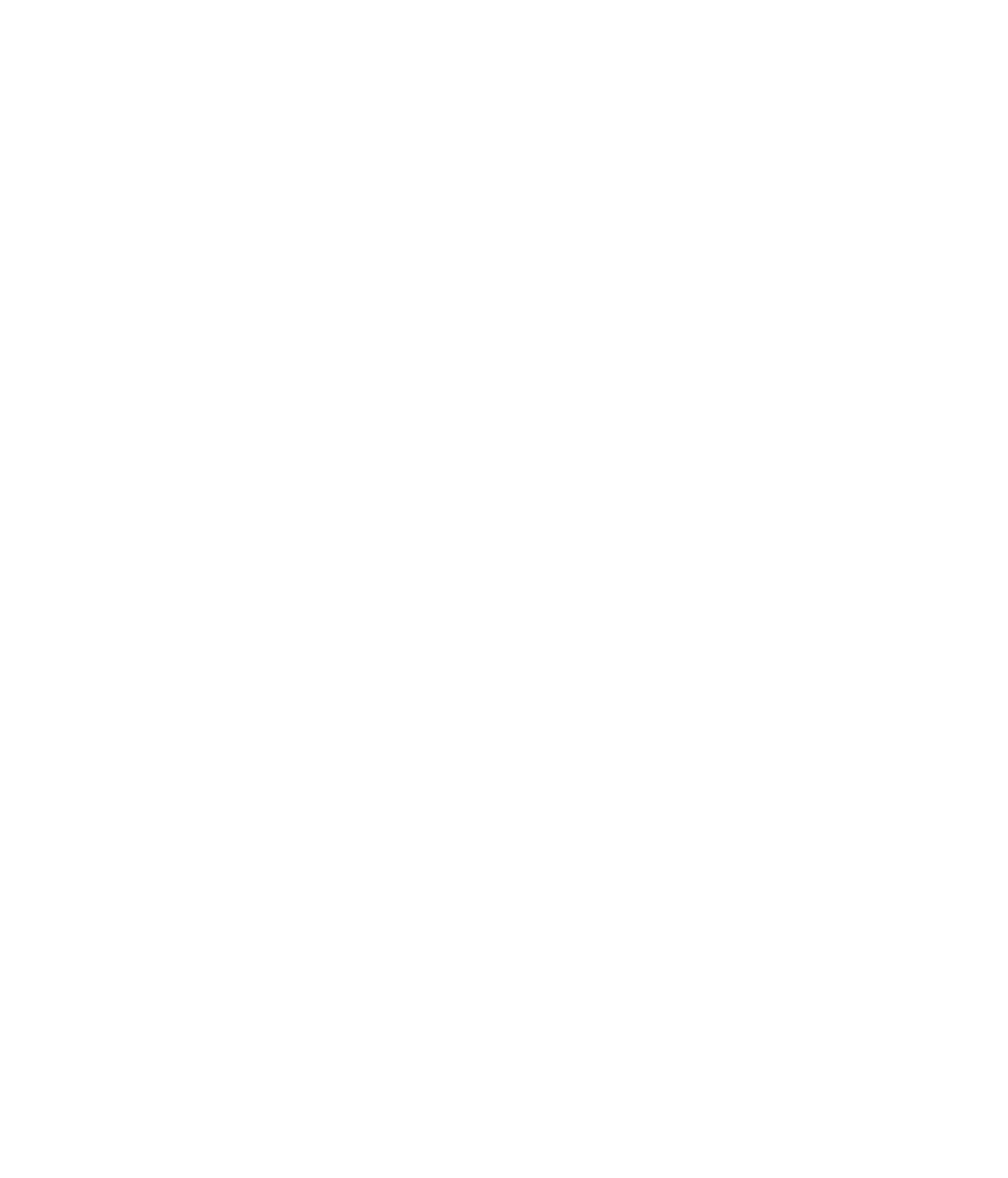




Search WWH ::

Custom Search