Java Reference
In-Depth Information
object, so you can instantiate an array using the
new
keyword and assigning a reference
to it, just like any other object. Arrays are fi xed in size and cannot dynamically grow or
shrink. (If you need a dynamically sized data structure, use one of the classes in the Java
Collections API found in the
java.util
package discussed in Chapter 7, “Collections and
Generics.”) This section discusses the details of declaring array references and instantiating
array objects, including the following topics:
How to declare array references
How to instantiate array objects
How to access the elements of an array
Multidimensional arrays
Array initializers
What arrays look like in memory
Array References
An
array reference
is a reference that denotes the data type of the values to be stored in the
array, using square brackets to denote the array reference. For example, the following code
declares three array references:
4. int [] finishTimes;
5. String lastNames [];
6. GregorianCalendar [] july;
Notice
lastNames
demonstrates how the square brackets can appear after the identifi er.
This technique is not recommended, though, because the code is more readable when the
square brackets appear before the identifi er.
The
finishTimes
reference can point to any array of
ints
. Similarly,
lastNames
can point to any array of
String
references and
july
can point to any array of
GregorianCalendar
references. Notice I didn't use the term “objects” when referring to
the elements of the array. The array is the object, but the contents of the array are either
primitive types or references, as we will see next.
Declaring an Array Reference
In Java it is not valid to declare a size for the array when declaring a reference. An array
reference can point to arrays of any length. The following code is not valid:
int [20] finishTimes; //not valid
String lastNames [100]; //not valid
When declaring an array reference, we are only specifying the data type of the elements
of the array. The size of the array is determined only when the array object is instantiated.
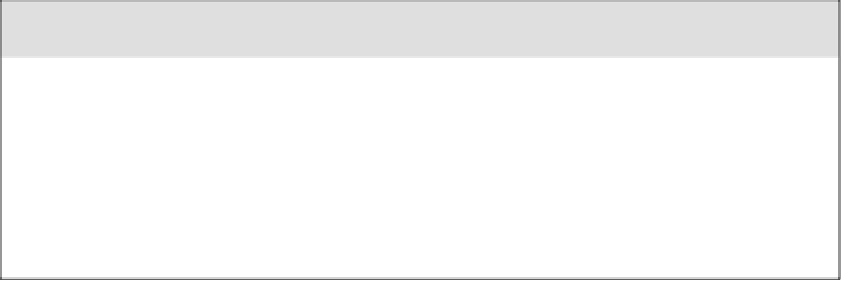





Search WWH ::

Custom Search