Java Reference
In-Depth Information
refer to it. Therefore,
s1 == s2
evaluates to
true
. On the other hand,
x1
and
x2
are not
literals but actual
String
objects created dynamically at runtime, making them distinct
objects. Therefore,
x1
and
x2
point to different objects and cannot be equal. The output of
the
ReferenceDemo
program is
f1 != f2
today == now
s1 == s2
The important point to take from this discussion is that evaluating
==
and
!=
on reference
types only compares whether or not the two references point to the same object. If you want to
compare the actual contents of two objects, the
equals
method is used, which we discuss next.
Equality of Objects
The exam objectives address the ability to “determine the equality of two objects or two
primitives.” As we saw in the previous section, you use the
==
operator to determine if
two primitives are equal. We also saw that two references are equal if and only if they
point to the same object. But what does it mean for two
objects
to be equal? (Don't forget:
references and objects are different entities!) As a Java programmer, you get to decide what
it means for two objects to be equal. The
java.lang.Object
class contains an
equals
method with the following signature:
public boolean equals (Object obj)
The default implementation in
Object
tests for reference equality, which we can already
perform with
==
. The general rule of thumb is to override
equals
in all your classes to
defi ne what it means for two objects of your class type to be equal. Equality should be
based on the business logic of your application.
The
equals
Method
Because the
equals
method is defi ned in
Object
, you can invoke
equals
on any object,
passing in any other object. For example, the following statements are valid:
String s = “Hello”;
java.util.Date d = new java.util.Date();
boolean b = s.equals(d);
The value of
b
is
false
. Logic would tell us that a
String
object and a
Date
object should
never be equal, and that is the case. Typically two objects have to be of the same class
type for them to be equal. However, that doesn't stop you from comparing two objects of
different types, because the
equals
method can be invoked with any two objects.
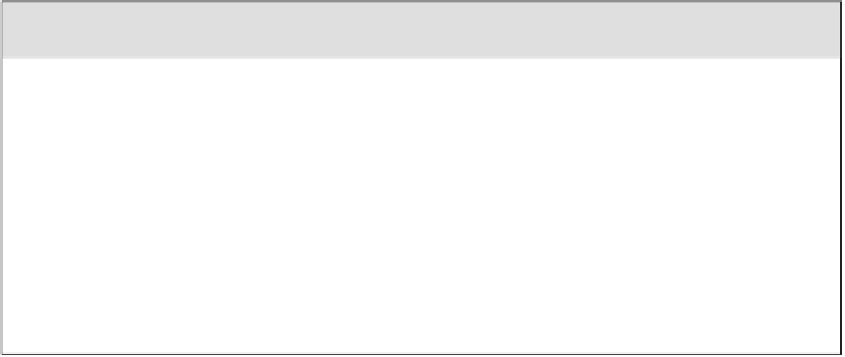
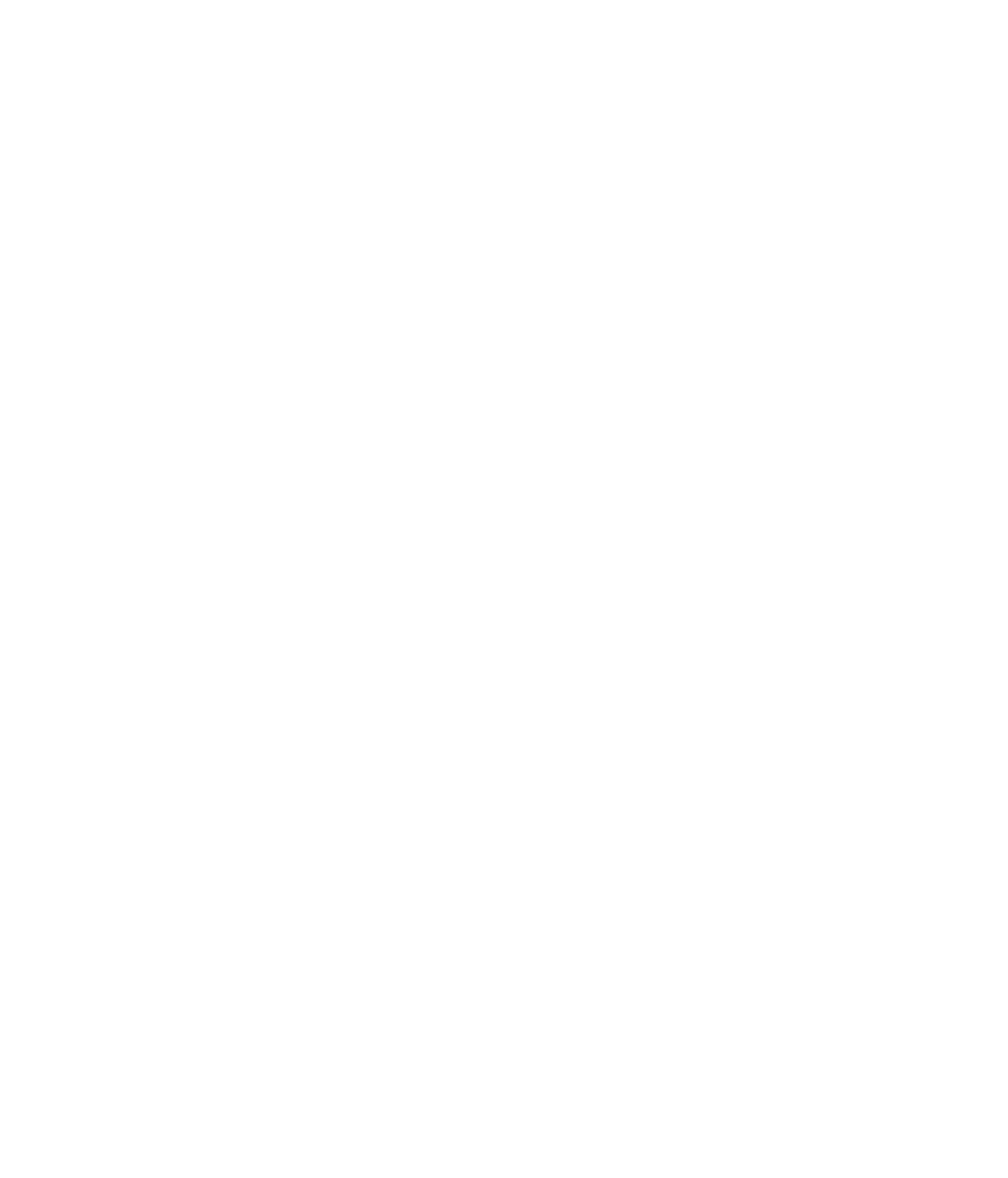




Search WWH ::

Custom Search