Java Reference
In-Depth Information
documentation will cause frustration for the end user, and in your real employment will cause
your support calls to be more aggravating than they need to be. Writing your documentation
up front means that it is less likely to be rushed, and also means that you are more likely to go
back to it several times during the course of your development and improve it.
Assertions
Assertions were added in JDK 1.4, and provide a useful confirmation and documentation of
assumptions for you as the developer without affecting deployment of your application.
The syntax of assertions is as follows:
assert <expression with a boolean result>;
or
assert <expression with a boolean result> : <any statement>;
If
<expression with a boolean result>
returns false, and assertion checking is turned on
at deploy time, an
AssertionError
is thrown.
Programmers sometimes make assumptions about how two parts of their program inter-
act—for example, they might assume that the value of a parameter in a private method will
never exceed a certain value. However, if the assumption is incorrect, the problems it causes
might not become obvious for quite some time. In such a case, it can be worthwhile to include
an assert statement at the start of the method that can be used while testing to confirm your
assumptions but that will not have any effect on the deployed code.
■
Caution
You should never use assertions to validate the inputs on public methods—these are methods
that other programmers may use, and they may not honor your requirements. You should validate these
inputs regardless of whether or not assertions are turned on at runtime.
Here is some example code to show validating a value:
public class AssertionTest {
public static void main(String[] args) {
new AssertionTest(11);
}
public AssertionTest(int withdrawalAmount) {
int balance = reduceBalance(withdrawalAmount);
// reduceBalance should never return a number less than zero
assert (balance < 0) : "Business rule: balance cannot be < 0";
}
}
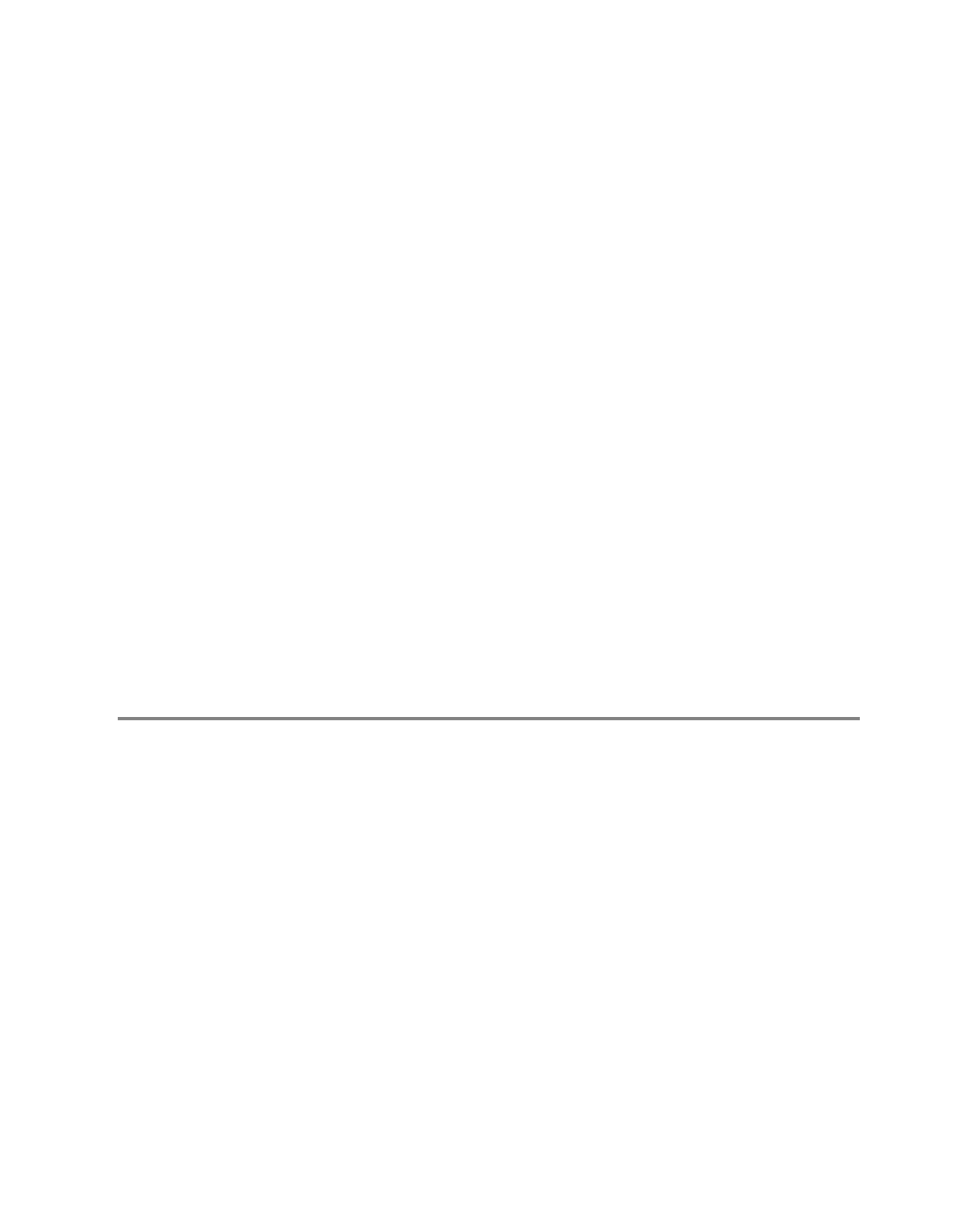

