Java Reference
In-Depth Information
All RMI implementations must be thread-safe because invocations on a particular object
could occur concurrently if the RMI runtime spawns multiple threads. There is no guarantee
regarding RMI thread management. Thus, it is your responsibility to make sure that remote
object implementations are thread-safe. In our case, using an RMI factory pattern in collabo-
ration with the
reserveDVD
and
releaseDVD
methods in the
DvdDatabase
class ensures that our
implementation is thread-safe.
If you choose not to extend
UnicastRemoteObject
but rather call the class method
UnicastRemoteObject.export
in the constructor, then the object methods such as
hashcode
,
toString
, and
equals
must be implemented. Listing 6-10 shows how the class constructor
and declaration would accommodate that approach (the rest of the code has been omitted
for conciseness).
Listing 6-10.
Exporting UnicastRemoteObject
public class DvdDatabaseImpl implements DvdDatabaseRemote
{
public DvdDatabaseImpl() throws RemoteException {
UnicastRemoteObject.exportObject(this);
this.dvdDatabase = new DvdDatabase();
}
}
Stubs and Skeletons
Only clients actually invoke methods on a
stub
, which is a local representation, or proxy,
of the remote object. While it appears that the client is calling the remote object directly,
unbeknownst to the client it is actually calling a proxy method in the stub that initiates com-
munication with the destination VM. The stub is responsible for packaging the parameters of
the remote method call prior to sending them across the network. The process of packaging
the parameters prior to shipping them across a network is called
marshaling
.
On the other side of the wire, or server side of the network, the incoming invocation is
eventually received by the remote reference layer, which demarshals the arguments and dis-
patches the call to the actual server object. The server then marshals the return value back to
the caller on the client side.
J2SE 1.5 introduces a new feature that alleviates the need to separately generate stub
classes for the client using the tool
rmic
. Now stub classes can be dynamically generated at
runtime by the JVM. (However, we are still required to use
rmic
for stub generation in our
submission.) To unconditionally generate stubs dynamically, set the JVM parameter
java.rmi.server.ignoreStubClasses
to true. This can be done using the
-D
option (i.e.,
java -Djava.rmi.server.ignoreStubClasses=true)
.
■
Note
You must still generate stub classes with
rmic
for backward compatibility with pre-J2SE 1.5
clients.
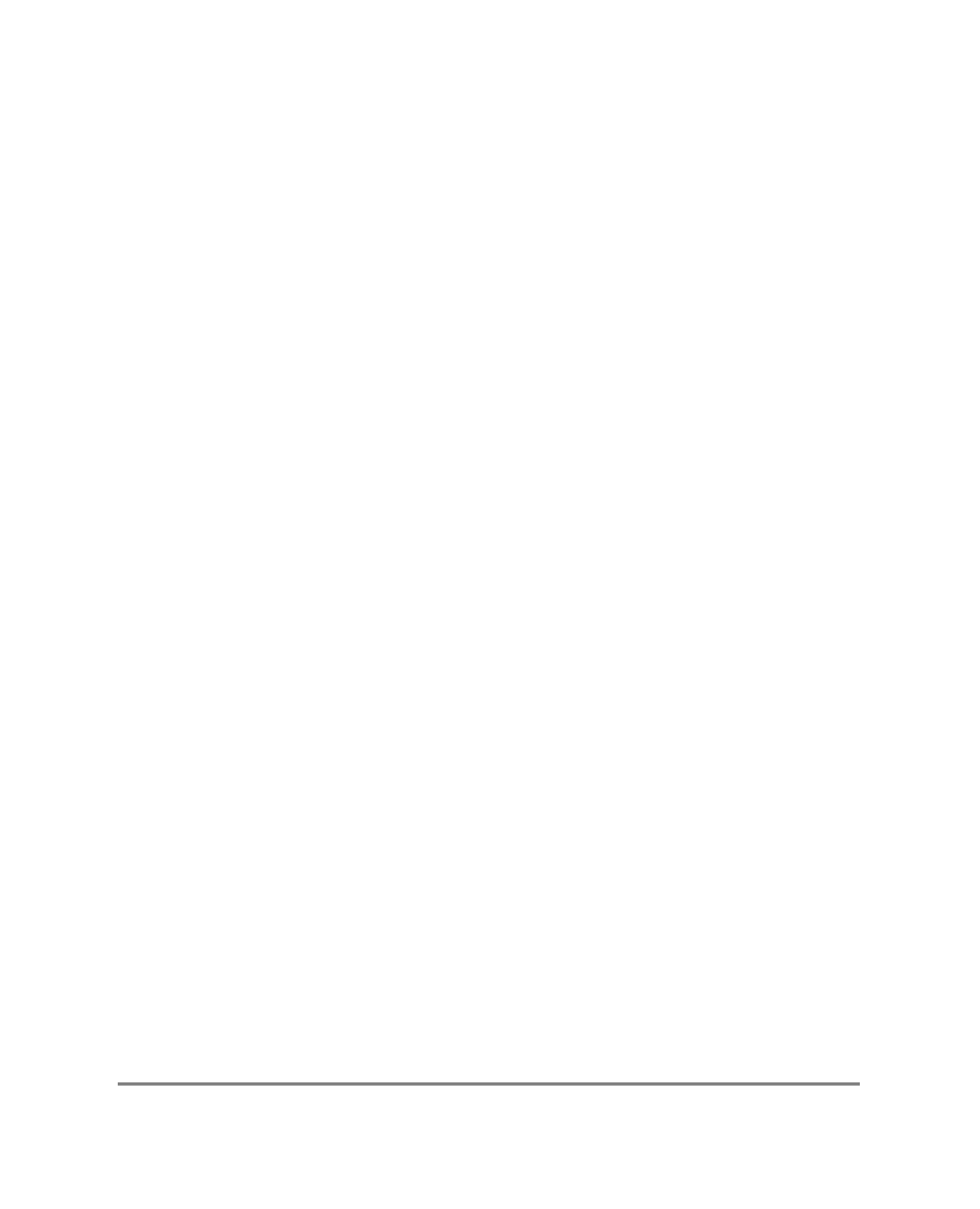

