Java Reference
In-Depth Information
Finally we write the record to file, and return true to show that the record was persisted
to file.
384 // now that we have everything ready to go, we can go into our
385 // synchronized block & perform our operations as quickly as possible
386 // ensuring that we block other users for as little time as possible.
387
388 synchronized(database) {
389 database.seek(offset);
390 database.write(out.toString().getBytes());
391 }
392
393 log.exiting("DvdFileAccess", "persistDvd", persisted);
394 return true;
395 }
Most of the remaining methods do not need explaining. However, we will end with the
find
method, which shows the enhanced for loop, as well as using the regular expressions
classes introduced in JDK 1.4.
311 public Collection<DVD> find(String query)
312 throws IOException, PatternSyntaxException {
313 log.entering("DvdFileAccess", "find", query);
314 Collection<DVD> returnValue = new ArrayList<DVD>();
315 Pattern p = Pattern.compile(query);
316
317 for (DVD dvd : getDvds()) {
318 Matcher m = p.matcher(dvd.toString());
319 if (m.find()) {
320 returnValue.add(dvd);
321 }
322 }
323
324 log.exiting("DvdFileAccess", "find", returnValue);
325 return returnValue;
326 }
In line 315 we take the string provided from our GUI application and compile it into a Java
Pattern
. We then need another class, the
Matcher
class, which can attempt to match the pat-
tern against the provided string in various ways; we generate the
Matcher
for each DVD read in
line 318. Finally, in line 319 we tell the
Matcher
to find the next occurrence of the pattern in the
current DVD's string representation; if found we add the DVD to the collection of DVDs to be
returned. While we are only interested in finding the pattern as a subset of the entire DVD, the
Matcher
can perform other types of matching as well; for instance, it can compare two strings
in their entirety, or match starting with the beginning of the string.
Line 317 shows the enhanced for loop syntax in action. Using this form saved us the
drudgery of manually creating our own iterator, and using generics saves us from casting
objects. Contrast the simple use of line 308 with the code we would have had to use if the for
loop had not been enhanced:
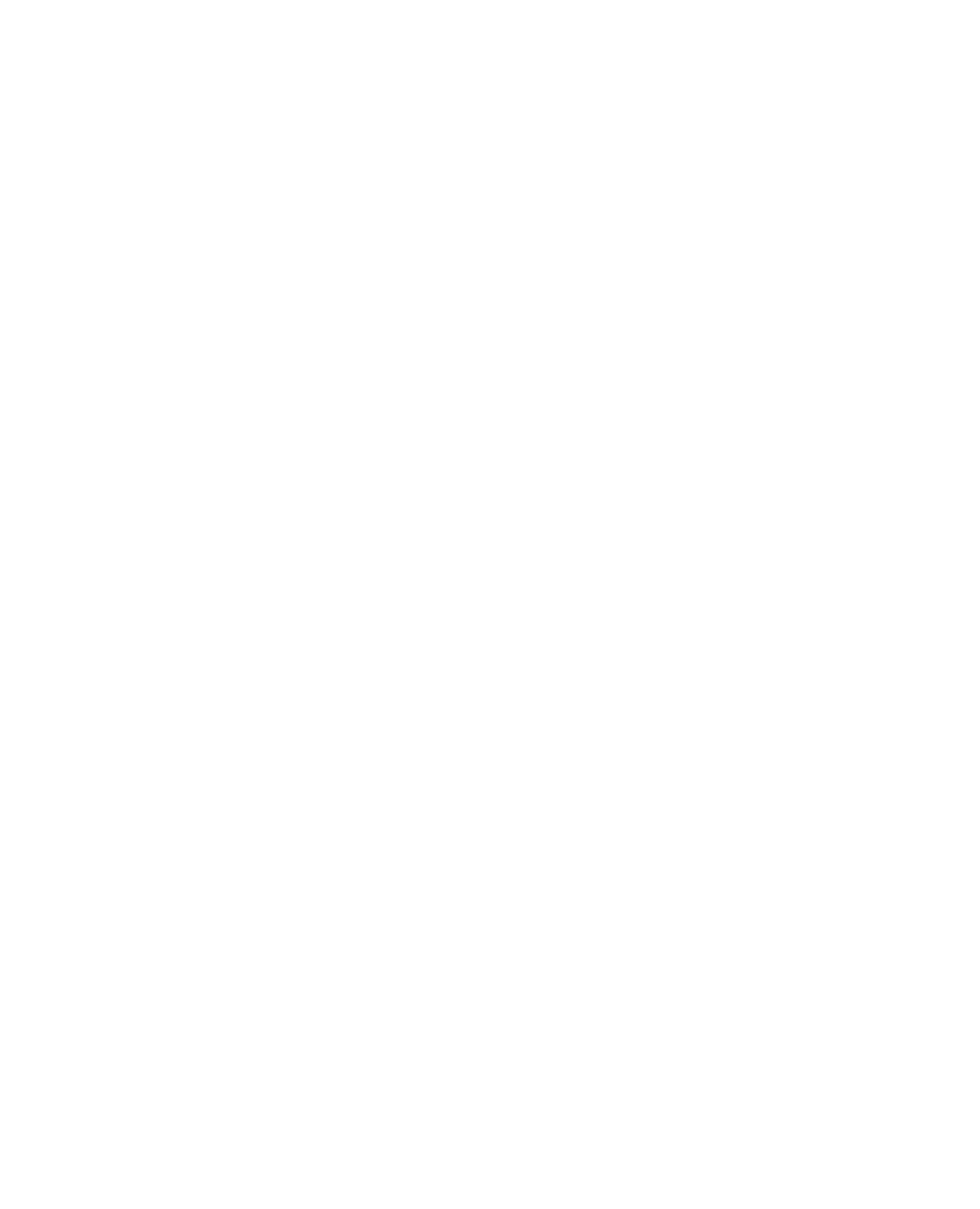