Java Reference
In-Depth Information
parallel if multiple clients are working on a multiple-CPU system, for example, converting
between a
DVD
value object and the bytes on file, or searching through the data file. In
addition, if we were to make the
DvdFileAccess
class a singleton, any class that uses the
DvdFileAccess
class would have to be coded differently than if it is a standard class—if we
were to later decide that this same class can be used to process multiple data files (with some
simple modifications), we would have to modify all the classes that use
DvdFileAccess
. There-
fore, this class is not a singleton.
As mentioned earlier,
DvdDatabase
is the façade through which all other classes should
access the data. Therefore, no other classes should call
DvdFileAccess
directly. To ensure this,
default access is set on the class itself—only classes within the
sampleproject.db
package can
access this class—as shown in line 31. As mentioned earlier, line numbers are not contiguous,
as source code comments have been removed.
1 package sampleproject.db;
2
3 import java.io.*;
4 import java.util.*;
5 import java.util.concurrent.locks.*;
6 import java.util.logging.*;
7 import java.util.regex.*;
..
31 class DvdFileAccess {
..
35 private static final String DATABASE_NAME = "dvd_db.dvd";
..
41 private Logger log = Logger.getLogger("sampleproject.db");
..
46 private static RandomAccessFile database = null;
..
51 private static Map<String, Long> recordNumbers
52 = new HashMap<String, Long>();
While most of the fields listed use the standard format from all previous versions of the
JDK, the
recordNumbers
collection uses the new generics declarations so that the compiler can
check that we are using the
generic
collection in a type-safe manner. This almost removes the
risk of us getting a
ClassCastException
at runtime. We will discuss the use of this particular
variable in the section following the class constructors.
58 private static ReadWriteLock recordNumbersLock
59 = new ReentrantReadWriteLock();
..
66 private static String emptyRecordString = null;
..
71 private static String dbPath = null;
..
77 static {
78 emptyRecordString = new String(new byte[DVD.RECORD_LENGTH]);
79 }
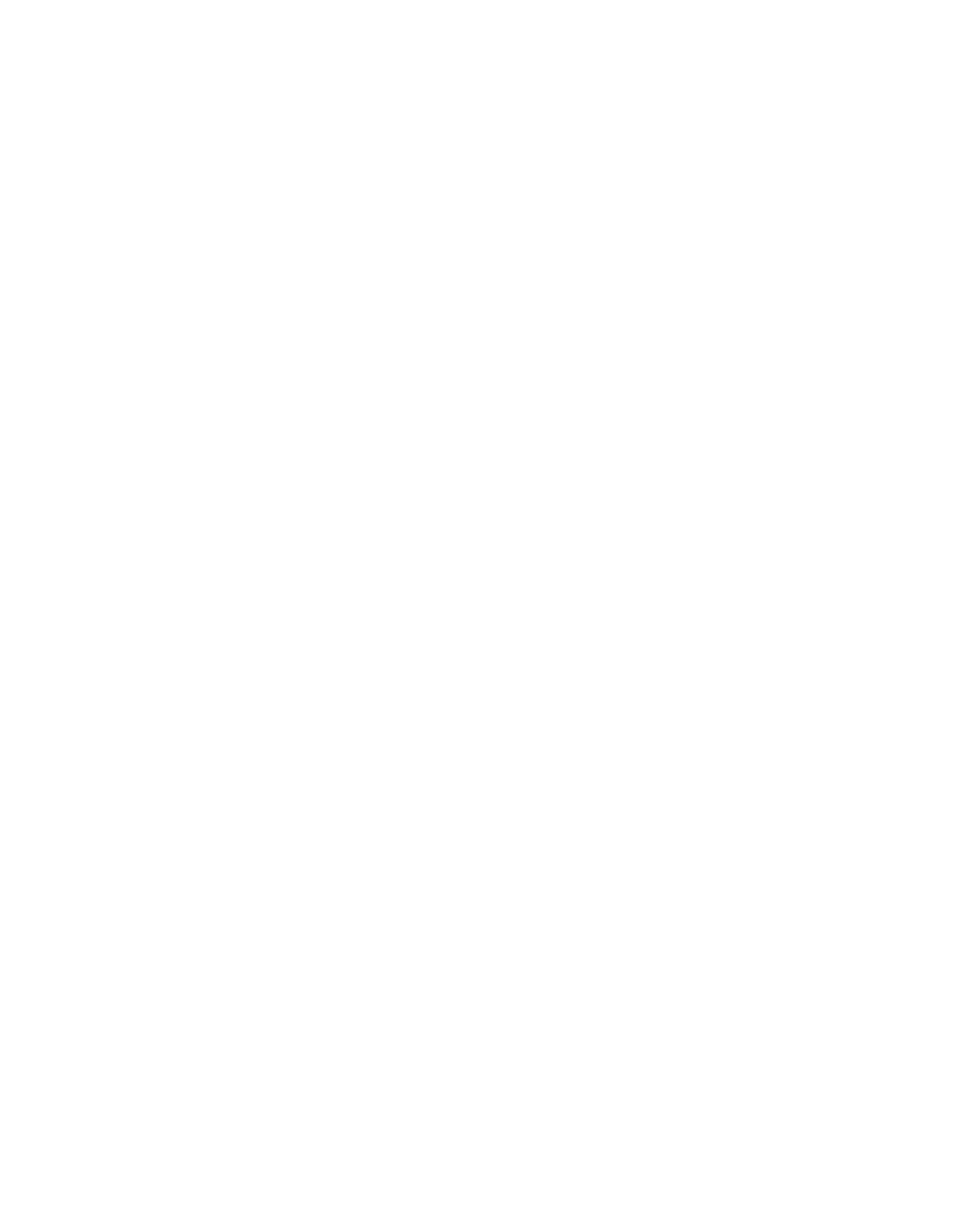