Java Reference
In-Depth Information
■
Note
The reason that we've specified threads slicing out in the
middle
of line 1 or 2 is that the
add
method for both
Vector
s and
ArrayList
s are methods that perform several actions before actually adding
the requested object to the underlying store.
Vector
s are not superior to
ArrayList
s, nor are
ArrayList
s superior to
Vector
s. There are
times when you do not need the overhead of synchronization on the
Collection
, and there are
times when you absolutely must have it. For example, a local method variable might as well be
an
ArrayList
, because it only exists in the context of that particular method; thus, there is no
opportunity for any other threads to modify it.
However, where possible we recommend against using the
Vector
class. There is the pos-
sibility that a junior programmer may incorrectly believe that use of a
Vector
is providing
thread safety other than what synchronized classes actually guarantee. Plus, there is the risk
that a later programmer may change the
Vector
to some other class, not realizing that a syn-
chronized class was required.
Synchronized objects have their purpose, as do unsynchronized ones. Part of earning
your Java developer certification is knowing what those purposes are.
Client Synchronization
Client synchronization is the process of making sure that no other thread interrupts your
method while it is mid-stride. For example, while
myVector.add(Object)
is a synchronized
operation, the internal synchronization of the
Vector
object did not guarantee that another
thread would not empty the
myVector
before the next step of your method executed. Consider
the following example:
1 public boolean addDVD(DVD dvd ){
2 for (int i = 0; i < myVector.length(); i++) {
3 doSomethingWith(myVector.get(i));
4 }
5 }
While you are guaranteed that line 2 will not be hijacked mid-stride, you are not guaran-
teed that some other thread will not set empty the
myVector
object by the time you get to line 3.
The current thread could slice out between lines 2 and 3, and the thread that picks up could
just happen to be one that corrupts
myVector
. The chances are probably small, but the possi-
bility for mischief does exist.
Be aware of such risks, even if you decide to accept them: Another thread could always
slice in between two unsynchronized lines of code even if they're calls to synchronized methods.
To guarantee exclusivity, you could modify the method in one of two ways. The first way is
to synchronize the method:
1 public synchronized boolean addDVD(DVD dvd ) {
2 for (int i = 0; i < myVector.length(); i++) {
3 doSomethingWith(myVector.get(i));
4 }
5 }
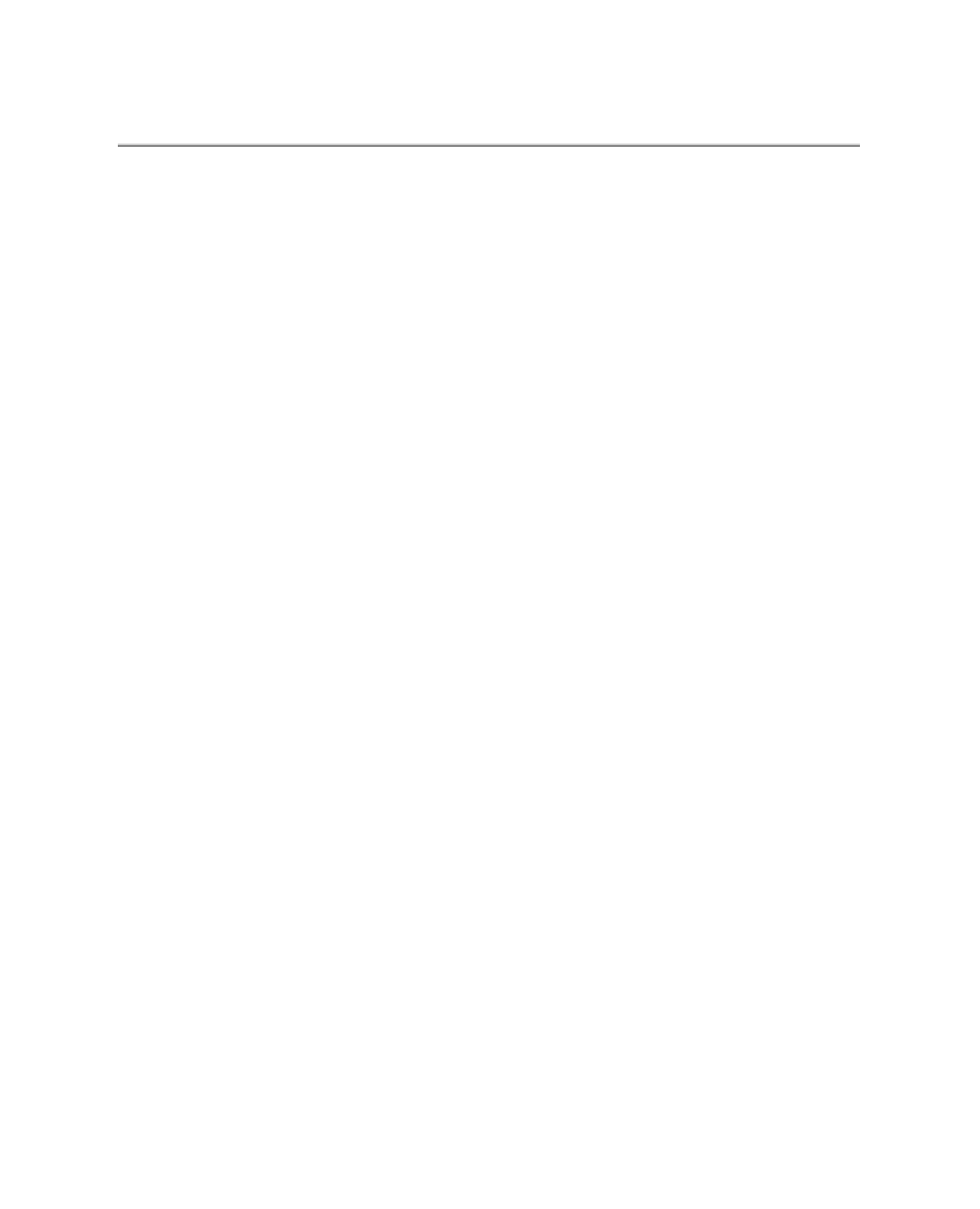

