Java Reference
In-Depth Information
11 Runner thread1 = new Runner(lock1, lock2);
12 Runner thread2 = new Runner(lock2, lock1);
13
14 thread1.start();
15 thread2.start();
16 }
17
18 /**
19 * Lock two objects in the order they were specified in the constructor.
20 */
21 static class Runner extends Thread {
22 private Object lockA;
23 private Object lockB;
24
25 public Runner(Object firstLockToGet, Object secondLockToGet) {
26 this.lockA = firstLockToGet;
27 this.lockB = secondLockToGet;
28 }
29
30 public void run() {
31 String name = Thread.currentThread().getName();
32 synchronized (lock1) {
33 System.out.println(name + ": locked " + lockA);
34 delay(name);
35 System.out.println(name + ": trying to get " + lockB);
36 synchronized (lock2) {
37 System.out.println(name + ": locked " + lockB);
38 }
39 }
40 }
41 }
42
43 /**
44 * build in a delay to allow the other thread time to lock the object
45 * the delaying thread would like to get.
46 */
47 private static void delay(String name) {
48 try {
49 System.out.println(name + ": delaying 1 second");
50 Thread.sleep(1000L);
51 } catch (InterruptedException ie) {
52 ie.printStackTrace();
53 }
54 }
55 }
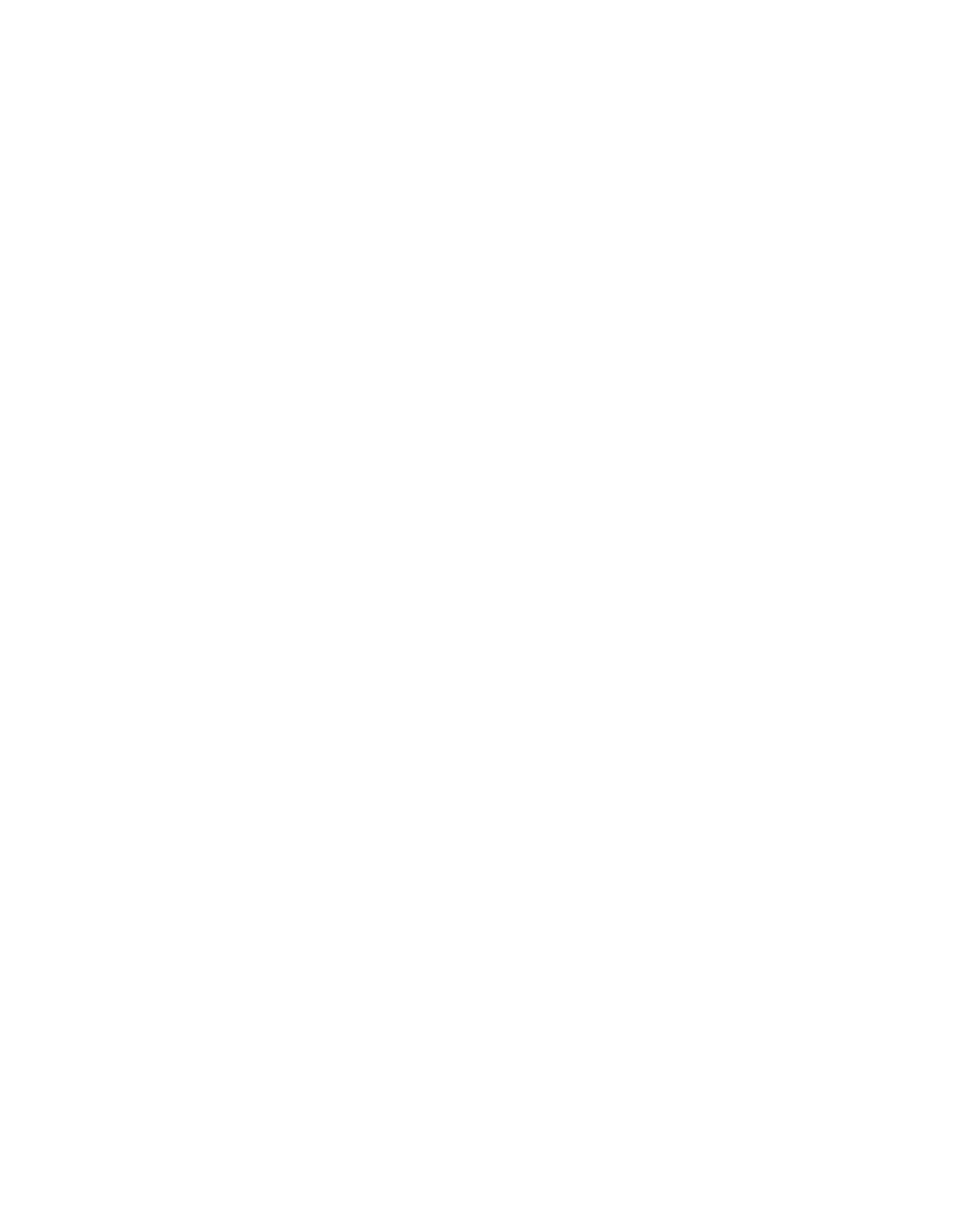