Java Reference
In-Depth Information
Example 4−5: Timer.java (continued)
// Go back to top of the loop to see if we've been stopped
continue;
}
// Now acquire a lock on the set of tasks
synchronized(tasks) {
long timeout; // how many ms 'till the next execution?
if (tasks.isEmpty()) { // If there aren't any tasks
timeout = 0; // Wait 'till notified of a new task
}
else {
// If there are scheduled tasks, then get the first one
// Since the set is sorted, this is the next one.
TimerTask t = (TimerTask) tasks.first();
// How long 'till it is next run?
timeout = t.nextTime - System.currentTimeMillis();
// Check whether it needs to run now
if (timeout <= 0) {
readyToRun = t; // Save it as ready to run
tasks.remove(t); // Remove it from the set
// Break out of the synchronized section before
// we run the task
continue;
}
}
// If we get here, there is nothing ready to run now,
// so wait for time to run out, or wait 'till notify() is
// called when something new is added to the set of tasks.
try { tasks.wait(timeout); }
catch (InterruptedException e) {}
// When we wake up, go back up to the top of the while loop
}
}
}
}
/** This inner class defines a test program */
public static class Test {
public static void main(String[] args) {
final TimerTask t1 = new TimerTask() { // Task 1: print "boom"
public void run() { System.out.println("boom"); }
};
final TimerTask t2 = new TimerTask() { // Task 2: print "BOOM"
public void run() { System.out.println("\tBOOM"); }
};
final TimerTask t3 = new TimerTask() { // Task 3: cancel the tasks
public void run() { t1.cancel(); t2.cancel(); }
};
// Create a timer, and schedule some tasks
final Timer timer = new Timer();
timer.schedule(t1, 0, 500); // boom every .5sec starting now
timer.schedule(t2, 2000, 2000); // BOOM every 2s, starting in 2s
timer.schedule(t3, 5000);
// Stop them after 5 seconds
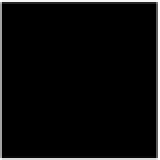
