Java Reference
In-Depth Information
Example 4−3: Deadlock.java (continued)
}
};
// Start the two threads. If all goes as planned, deadlock will occur,
// and the program will never exit.
t1.start();
t2.start();
}
}
Timers
Java 1.3 introduces the
java.util.Timer
class and the abstract
java.util.Timer-
Task
class. If you subclass
TimerTask
and implement its
run()
method, you can
then use a
Timer
object to schedule invocations of that
run()
method at a speci-
fied time or at multiple times at a specified interval. One
Timer
object can sched-
ule and invoke many
TimerTask
objects.
Timer
is quite useful, as it simplifies many
programs that would otherwise have to create their own threads to provide the
same functionality. Note that
java.util.Timer
is not at all the same as the Java 1.2
class
javax.swing.Timer
.
Example 4-4 and Example 4-5 are simple implementations of the
TimerTask
and
Timer
classes that can be used prior to Java 1.3. They implement the same API as
the Java 1.3 classes, except that they are in the
com.davidflanagan.exam-
ples.thread
package, instead of the
java.util
package. These implementations
are not intended to be as robust as the official implementations in Java 1.3, but
they are useful for simple tasks and are a good example of a nontrivial use of
threads. Note in particular the use of
wait()
and
notify()
in Example 4-5. After
studying these examples, you may be interested to compare them to the imple-
mentations that come with Java 1.3.
*
Example 4−4: TimerTask.java
package com.davidflanagan.examples.thread;
/**
* This class implements the same API as the Java 1.3 java.util.TimerTask.
* Note that a TimerTask can only be scheduled on one Timer at a time, but
* that this implementation does not enforce that constraint.
**/
public abstract class TimerTask implements Runnable {
boolean cancelled = false;
// Has it been cancelled?
long nextTime = -1;
// When is it next scheduled?
long period;
// What is the execution interval
boolean fixedRate;
// Fixed-rate execution?
protected TimerTask() {}
/**
* Cancel the execution of the task. Return true if it was actually
* running, or false if it was already cancelled or never scheduled.
*
If you have the Java SDK™ from Sun, look in the
src.jar
archive that comes with it.
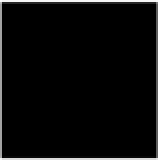
