Java Reference
In-Depth Information
face. In this case, the objects themselves have
compareTo()
methods, so they can
be compared directly.
Note that in Java 1.2 and later, the
java.util.Arrays
class defines a number of
sort()
methods for sorting arrays of objects or primitive values. Also,
java.util.Collections
defines
sort()
methods to sort
java.util.List
objects
(these classes are part of the Java collection framework introduced in Java 1.2).
These classes and methods are preferred over the sorting methods developed
here. Nevertheless, Example 2-9 is still an interesting and useful example. Note
also that the sorting methods in
Arrays
and
Collections
use
java.util.Compara-
tor
and
java.lang.Comparable
interfaces, which are similar to the
Comparer
and
Comparable
interfaces in this example.
Example 2-9 rounds out this chapter. If you've skimmed ahead and looked at the
program, you probably noticed that it is a rather complex example. As such, it is
worth studying carefully. In particular, the program makes heavy use of inner
classes, so you should be sure you understand how inner classes work before you
examine this code in detail. As usual, there is an inner
Test
class at the end of the
example, but inner classes and interfaces are used throughout the program.
Example 2•9: Sorter.java
package com.davidflanagan.examples.classes;
// These are some classes we need for internationalized string sorting
import java.text.Collator;
import java.text.CollationKey;
import java.util.Locale;
/**
* This class defines a bunch of static methods for efficiently sorting
* arrays of Strings or other objects. It also defines two interfaces that
* provide two different ways of comparing objects to be sorted.
**/
public class Sorter {
/**
* This interface defines the compare() method used to compare two objects.
* To sort objects of a given type, you must provide a Comparer
* object with a compare() method that orders those objects as desired
**/
public static interface Comparer {
/**
* Compare objects, return a value that indicates their relative order:
* if (a > b) return > 0;
* if (a == b) return 0;
* if (a < b) return < 0.
**/
public int compare(Object a, Object b);
}
/**
* This is an alternative interface that can be used to order objects. If
* a class implements this Comparable interface, then any two instances of
* that class can be directly compared by invoking the compareTo() method.
**/
public static interface Comparable {
/**
* Compare objects, return a value that indicates their relative order:
* if (this > other) return > 0
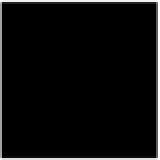
