Java Reference
In-Depth Information
Example 18−2: Counter.java (continued)
}
// This method is called when the web server stops the servlet (which
// happens when the web server is shutting down, or when the servlet is
// not in active use.) This method saves the counts to a file so they
// can be restored when the servlet is restarted.
public void destroy() {
try { saveState(); } // Try to save the state
catch(Exception e) {} // Ignore any problems: we did the best we could
}
// These constants define the request parameter and attribute names that
// the servlet uses to find the name of the counter to increment.
public static final String PARAMETER_NAME = "counter";
public static final String ATTRIBUTE_NAME =
"com.davidflanagan.examples.servlet.Counter.counter";
/**
* This method is called when the servlet is invoked. It looks for a
* request parameter named "counter", and uses its value as the name of
* the counter variable to increment. If it doesn't find the request
* parameter, then it uses the URL of the request as the name of the
* counter. This is useful when the servlet is mapped to a URL suffix.
* This method also checks how much time has elapsed since it last saved
* its state, and saves the state again if necessary. This prevents it
* from losing too much data if the server crashes or shuts down without
* calling the destroy() method.
**/
public void doGet(HttpServletRequest request, HttpServletResponse response)
throws IOException
{
// Get the name of the counter as a request parameter
String counterName = request.getParameter(PARAMETER_NAME);
// If we didn't find it there, see if it was passed to us as a
// request attribute, which happens when the output of this servlet
// is included by another servlet
if (counterName == null)
counterName = (String) request.getAttribute(ATTRIBUTE_NAME);
// If it wasn't a parameter or attribute, use the request URL.
if (counterName == null) counterName = request.getRequestURI();
Integer count; // What is the current count?
// This block of code is synchronized because multiple requests may
// be running at the same time in different threads. Synchronization
// prevents them from updating the counts hashtable at the same time
synchronized(counts) {
// Get the counter value from the hashtable
count = (Integer)counts.get(counterName);
// Increment the counter, or if it is new, log and start it at 1
if (count != null) count = new Integer(count.intValue() + 1);
else {
// If this is a counter we haven't used before, send a message
// to the log file, just so we can track what we're counting
log("Starting new counter: " + counterName);
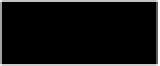
