Java Reference
In-Depth Information
ComplexNumber
defines two
add()
methods and two
multiply()
methods that per-
form addition and multiplication of complex numbers. The difference between the
two versions of each method is that one is an instance method and one is a class,
or static, method. Consider the
add()
methods, for example. The instance method
adds the value of the current instance of
ComplexNumber
to another specified
Com-
plexNumber
object. The class method doesn't have a current instance; it simply
adds the values of two specified
ComplexNumber
objects. The instance method is
invoked through an instance of the class, like this:
ComplexNumber sum = a.add(b);
The class method, however, is invoked through the class itself, rather than through
an instance:
ComplexNumber sum = ComplexNumber.add(a, b);
Example 2•5: ComplexNumber.java
package com.davidflanagan.examples.classes;
/**
* This class represents complex numbers, and defines methods for performing
* arithmetic on complex numbers.
**/
public class ComplexNumber {
// These are the instance variables. Each ComplexNumber object holds
// two double values, known as x and y. They are private, so they are
// not accessible from outside this class. Instead, they are available
// through the real() and imaginary() methods below.
private double x, y;
/** This is the constructor. It initializes the x and y variables */
public ComplexNumber(double real, double imaginary) {
this.x = real;
this.y = imaginary;
}
/**
* An accessor method. Returns the real part of the complex number.
* Note that there is no setReal() method to set the real part. This means
* that the ComplexNumber class is "immutable".
**/
public double real() { return x; }
/** An accessor method. Returns the imaginary part of the complex number */
public double imaginary() { return y; }
/** Compute the magnitude of a complex number */
public double magnitude() { return Math.sqrt(x*x + y*y); }
/**
* This method converts a ComplexNumber to a string. This is a method of
* Object that we override so that complex numbers can be meaningfully
* converted to strings, and so they can conveniently be printed out with
* System.out.println() and related methods
**/
public String toString() { return "{" + x + "," + y + "}"; }
/**
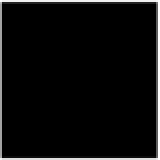
