Java Reference
In-Depth Information
Example 17−1: ExecuteSQL.java (continued)
try {
String driver = null, url = null, user = "", password = "";
// Parse all the command-line arguments
for(int n = 0; n < args.length; n++) {
if (args[n].equals("-d")) driver = args[++n];
else if (args[n].equals("-u")) user = args[++n];
else if (args[n].equals("-p")) password = args[++n];
else if (url == null) url = args[n];
else throw new IllegalArgumentException("Unknown argument.");
}
// The only required argument is the database URL.
if (url == null)
throw new IllegalArgumentException("No database specified");
// If the user specified the classname for the DB driver, load
// that class dynamically. This gives the driver the opportunity
// to register itself with the DriverManager.
if (driver != null) Class.forName(driver);
// Now open a connection the specified database, using the
// user-specified username and password, if any. The driver
// manager will try all of the DB drivers it knows about to try to
// parse the URL and connect to the DB server.
conn = DriverManager.getConnection(url, user, password);
// Now create the statement object we'll use to talk to the DB
Statement s = conn.createStatement();
// Get a stream to read from the console
BufferedReader in =
new BufferedReader(new InputStreamReader(System.in));
// Loop forever, reading the user's queries and executing them
while(true) {
System.out.print("sql> "); // prompt the user
System.out.flush(); // make the prompt appear now.
String sql = in.readLine(); // get a line of input from user
// Quit when the user types "quit".
if ((sql == null) || sql.equals("quit")) break;
// Ignore blank lines
if (sql.length() == 0) continue;
// Now, execute the user's line of SQL and display results.
try {
// We don't know if this is a query or some kind of
// update, so we use execute() instead of executeQuery()
// or executeUpdate() If the return value is true, it was
// a query, else an update.
boolean status = s.execute(sql);
// Some complex SQL queries can return more than one set
// of results, so loop until there are no more results
do {
if (status) { // it was a query and returns a ResultSet
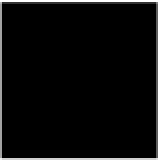
