Java Reference
In-Depth Information
Example 16−7: MudClient.java (continued)
// Now go process the command. What follows is a huge repeated
// if/else statement covering each of the commands supported by
// this client. Many of these commands simply invoke one of
// the remote methods of the current RemoteMudPlace object.
// Some have to do a bit of additional processing.
// LOOK: Describe the place and its things, people, and exits
if (cmd.equals("look")) look(location);
// EXAMINE: Describe a named thing
else if (cmd.equals("examine"))
System.out.println(location.examineThing(arg));
// DESCRIBE: Describe a named person
else if (cmd.equals("describe")) {
try {
RemoteMudPerson p = location.getPerson(arg);
System.out.println(p.getDescription());
}
catch(RemoteException e) {
System.out.println(arg + " is having technical " +
"difficulties. No description " +
"is available.");
}
}
// GO: Go in a named direction
else if (cmd.equals("go")) {
location = location.go(me, arg);
mudname = location.getServer().getMudName();
placename = location.getPlaceName();
look(location);
}
// SAY: Say something to everyone
else if (cmd.equals("say")) location.speak(me, arg);
// DO: Do something that will be described to everyone
else if (cmd.equals("do")) location.act(me, arg);
// TALK: Say something to one named person
else if (cmd.equals("talk")) {
try {
RemoteMudPerson p = location.getPerson(arg);
String msg = getLine("What do you want to say?: ");
p.tell(myname + " says \"" + msg + "\"");
}
catch (RemoteException e) {
System.out.println(arg + " is having technical " +
"difficulties. Can't talk to them.");
}
}
// CHANGE: Change my own description
else if (cmd.equals("change"))
me.setDescription(
getMultiLine("Describe yourself for others: "));
// CREATE: Create a new thing in this place
else if (cmd.equals("create")) {
if (arg.length() == 0)
throw new IllegalArgumentException("name expected");
String desc = getMultiLine("Please describe the " +
arg + ": ");
location.createThing(me, arg, desc);
}
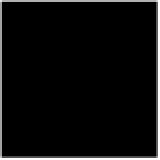
