Java Reference
In-Depth Information
The code for parsing the user's input and computing and printing the factorial is
the same as in Example 1-11, and again, it is enclosed within a
try
clause. In
Example 1-12, however, there is only a single
catch
clause to handle the possible
exceptions. This one handles any exception object of type
Exception
.
Exception
is the superclass of all exception types, so this one
catch
clause is invoked no
matter what type of exception is thrown.
Example 1−12: FactQuoter.java
package com.davidflanagan.examples.basics;
import java.io.*; // Import all classes in java.io package. Saves typing.
/**
* This program displays factorials as the user enters values interactively
**/
public class FactQuoter {
public static void main(String[] args) throws IOException {
// This is how we set things up to read lines of text from the user.
BufferedReader in=new BufferedReader(new InputStreamReader(System.in));
// Loop forever
for(;;) {
// Display a prompt to the user
System.out.print("FactQuoter> ");
// Read a line from the user
String line = in.readLine();
// If we reach the end-of-file,
// or if the user types "quit", then quit
if ((line == null) || line.equals("quit")) break;
// Try to parse the line, and compute and print the factorial
try {
int x = Integer.parseInt(line);
System.out.println(x + "! = " + Factorial4.factorial(x));
}
// If anything goes wrong, display a generic error message
catch(Exception e) { System.out.println("Invalid Input"); }
}
}
}
Using a StringBuffer
One of the things you may have noticed about the
String
class that is used to rep-
resent strings in Java is that it is immutable. In other words, there are no methods
that allow you to change the contents of a string. Methods that operate on a string
return a new string, not a modified copy of the old one. When you want to oper-
ate on a string in place, you must use a
StringBuffer
object instead.
Example 1-13 demonstrates the use of a
StringBuffer
. It interactively reads a line
of user input, as Example 1-12 did, and creates a
StringBuffer
to contain the line.
The program then encodes each character of the line using the
rot13
substitution
cipher, which simply “rotates” each letter 13 places through the alphabet, wrap-
ping around from Z back to A when necessary. Because a
StringBuffer
object is
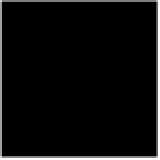
