Java Reference
In-Depth Information
Example 13−2: Scribble.java (continued)
}
}
return scribble;
}
// ========= The following methods implement the Shape interface ========
/** Return the bounding box of the Shape */
public Rectangle getBounds() {
return new Rectangle((int)(minX-0.5f), (int)(minY-0.5f),
(int)(maxX-minX+0.5f), (int)(maxY-minY+0.5f));
}
/** Return the bounding box of the Shape */
public Rectangle2D getBounds2D() {
return new Rectangle2D.Double(minX, minY, maxX-minX, maxY-minY);
}
/** Our shape is an open curve, so it never contains anything */
public boolean contains(Point2D p) { return false; }
public boolean contains(Rectangle2D r) { return false; }
public boolean contains(double x, double y) { return false; }
public boolean contains(double x, double y, double w, double h) {
return false;
}
/**
* Determine if the scribble intersects the specified rectangle by testing
* each line segment individually
**/
public boolean intersects(Rectangle2D r) {
if (numPoints < 4) return false;
int i = 0;
double x1, y1, x2 = 0.0, y2 = 0.0;
while(i < numPoints) {
if (Double.isNaN(points[i])) { // If we're beginning a new line
i++;
// Skip the NaN
x2 = points[i++];
y2 = points[i++];
}
else {
x1 = x2;
y1 = y2;
x2 = points[i++];
y2 = points[i++];
if (r.intersectsLine(x1, y1, x2, y2)) return true;
}
}
return false;
}
/** Test for intersection by invoking the method above */
public boolean intersects(double x, double y, double w, double h){
return intersects(new Rectangle2D.Double(x,y,w,h));
}
/**
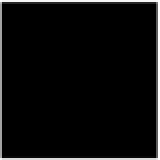
