Java Reference
In-Depth Information
Example 11•13: ImageOps.java (continued)
0.0f, -0.75f, 0.0f,
-0.75f, 4.0f, -0.75f,
0.0f, -0.75f, 0.0f})),
// 9) Edge detect using yet another matrix
new ConvolveOp(new Kernel(3, 3, new float[] {
0.0f, -0.75f, 0.0f,
-0.75f, 3.0f, -0.75f,
0.0f, -0.75f, 0.0f})),
// 10) Compute a mirror image using the transform defined above
new AffineTransformOp(mirrorTransform,AffineTransformOp.TYPE_BILINEAR),
// 11) Rotate the image 180 degrees about its center point
new AffineTransformOp(AffineTransform.getRotateInstance(Math.PI,64,95),
AffineTransformOp.TYPE_NEAREST_NEIGHBOR),
// 12) Rotate the image 15 degrees about the bottom left
new AffineTransformOp(AffineTransform.getRotateInstance(Math.PI/12,
0, 190),
AffineTransformOp.TYPE_NEAREST_NEIGHBOR),
};
/** Draw the example */
public void draw(Graphics2D g, Component c) {
// Create a BufferedImage big enough to hold the Image loaded
// in the constructor. Then copy that image into the new
// BufferedImage object so that we can process it.
BufferedImage bimage = new BufferedImage(image.getWidth(c),
image.getHeight(c),
BufferedImage.TYPE_INT_RGB);
Graphics2D ig = bimage.createGraphics();
ig.drawImage(image, 0, 0, c); // copy the image
// Set some default graphics attributes
g.setFont(new Font("SansSerif", Font.BOLD, 12)); // 12pt bold text
g.setColor(Color.green);
// Draw in green
g.translate(10, 10);
// Set some margins
// Loop through the filters
for(int i = 0; i < filters.length; i++) {
// If the filter is null, draw the original image, otherwise,
// draw the image as processed by the filter
if (filters[i] == null) g.drawImage(bimage, 0, 0, c);
else g.drawImage(filters[i].filter(bimage, null), 0, 0, c);
g.drawString(filterNames[i], 0, 205); // Label the image
g.translate(137, 0); // Move over
if (i % 4 == 3) g.translate(-137*4, 215); // Move down after 4
}
}
}
A Custom Shape
Figure 11-4 showed how Java 2D can be used to draw and fill various types of
shapes. One of the shapes shown in that figure is a spiral, which was drawn using
the
Spiral
class, a custom
Shape
implementation shown in Example 11-14.
The
Shape
interface defines three important methods (some of which have multi-
ple overloaded versions) that all shapes must implement. The
contains()
methods
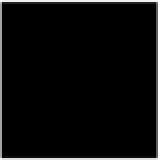
