Java Reference
In-Depth Information
Example 11•7: Transforms.java (continued)
/** Draw the defined shape and label, using each transform */
public void draw(Graphics2D g, Component c) {
// Define basic drawing attributes
g.setColor(Color.black); // black
g.setStroke(new BasicStroke(2.0f, BasicStroke.CAP_SQUARE, // 2-pixel
BasicStroke.JOIN_BEVEL));
g.setRenderingHint(RenderingHints.KEY_ANTIALIASING,
// antialias
RenderingHints.VALUE_ANTIALIAS_ON);
// Now draw the shape once using each of the transforms we've defined
for(int i = 0; i < transforms.length; i++) {
AffineTransform save = g.getTransform(); // save current state
g.translate(i*125 + 50, 50); // move origin
g.transform(transforms[i]); // apply transform
g.draw(shape); // draw shape
g.drawString(transformLabels[i], -25, 125); // draw label
g.drawRect(-40, -10, 80, 150);
// draw box
g.setTransform(save);
// restore transform
}
}
}
Line Styles with BasicStroke
In the last couple of examples, we've used the
BasicStroke
class to draw lines
that are wider than the one-pixel lines supported by the
Graphics
class. Wide lines
are more complicated than thin lines, however, so
BasicStroke
allows you to
specify other line attributes as well: the
cap style
of a line specifies how the end-
points of lines look, and the
join style
specifies how the corners, or vertices, of
shapes look. These style options for endpoints and vertices are shown in Figure
11-6. The figure also illustrates the use of a dot-dashed patterned line, which is
another feature of
BasicStroke
. The figure was produced using the code listed in
Example 11-8, which demonstrates how to use
BasicStroke
to draw wide lines
with a variety of cap and join styles and to draw patterned lines.
Figure 11•6. Line styles with BasicStroke
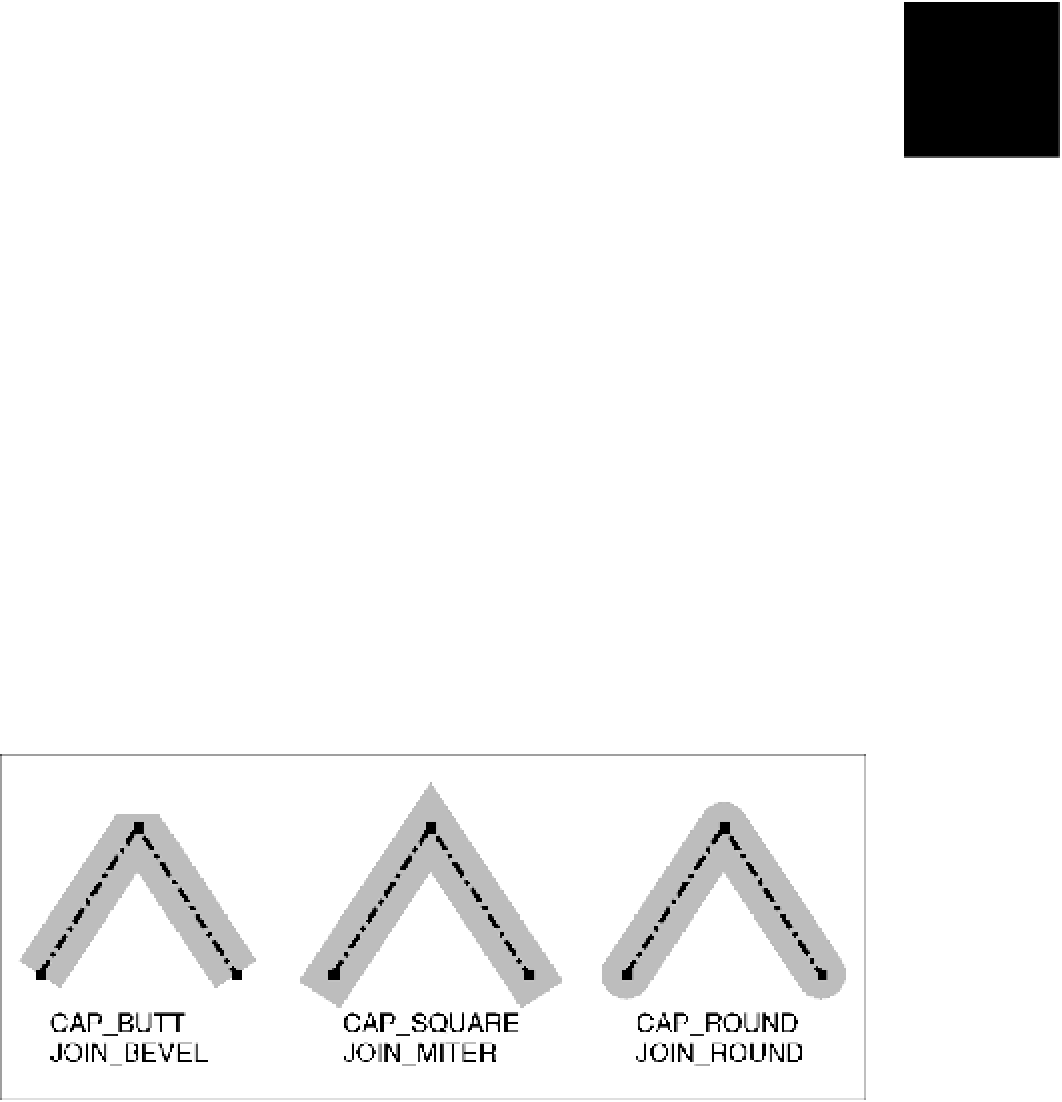
