Java Reference
In-Depth Information
The rest of this chapter is devoted to examples that demonstrate these features of
the Java 2D API. Most of the examples are implementations of the
GraphicsExam-
ple
interface, which is shown in Example 11-5.
Example 11•5: GraphicsExample.java
package com.davidflanagan.examples.graphics;
import java.awt.*;
/**
* This interface defines the methods that must be implemented by an
* object that is to be displayed by the GraphicsExampleFrame object
*/
public interface GraphicsExample {
public String getName(); // Return the example name
public int getWidth(); // Return its width
public int getHeight(); // Return its height
public void draw(Graphics2D g, Component c); // Draw the example
}
You can run these examples using the
GraphicsExampleFrame
frame program,
which is shown at the end of the chapter. This program creates a simple GUI,
instantiates the classes listed on the command line, and displays them in a win-
dow. For example, to display the
Shapes
example we'll see in the next section,
you can use
GraphicsExampleFrame
as follows:
% java com.davidflanagan.examples.graphics.GraphicsExampleFrame Shapes
Many of the figures shown in the chapter are drawn at high resolution; they are
not typical screenshots of a running program.
GraphicsExampleFrame
includes
printing functionality that generated these figures. Even though
GraphicsExample-
Frame
doesn't do any graphics drawing of its own and is therefore more of an
example of Java's printing capabilities, it is still listed in Example 11-18 in this
chapter.
Drawing and Filling Shapes
Example 11-6 lists a
GraphicsExample
implementation that shows how various
Shape
objects can be defined, drawn, and filled. The example produces the output
shown in Figure 11-4. Although the Java 2D API allows basic shapes to be drawn
and filled using the methods demonstrated in Example 11-1, this example uses a
different approach. It defines each shape as a
Shape
object, using various classes,
mostly from
java.awt.geom
.
Each
Shape
is drawn using the
draw()
method of the
Graphics2D
class and filled
using the
fill()
method. Note that each
Shape
object is defined with one corner
at (or near) the origin, rather than at the location where it is displayed on the
screen. This creates position-independent objects that can easily be reused. To
draw the shapes at particular locations, the example uses the
translate()
method
of
Graphics2D
to move the origin of the coordinate system. Finally, the call to
set-
Stroke()
specifies that drawing be done with a two-pixel-wide line, while the call
to
setRenderingHint()
requests that drawing be done using antialiasing.
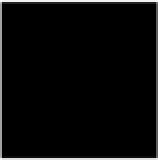
