Java Reference
In-Depth Information
Example 10•21: WebBrowser.java (continued)
}
/**
* Print the contents of the text pane using the java.awt.print API
* Note that this API does not work efficiently in Java 1.2
* All the hard work is done by the PrintableDocument class.
**/
public void print() {
// Get a PrinterJob object from the system
PrinterJob job = PrinterJob.getPrinterJob();
// This is the object that we are going to print
PrintableDocument pd = new PrintableDocument(textPane);
// Tell the PrinterJob what we want to print
job.setPageable(pd);
// Display a print dialog, asking the user what pages to print, what
// printer to print to, and giving the user a chance to cancel.
if (job.printDialog()) { // If the user did not cancel
try { job.print(); } // Start printing!
catch(PrinterException ex) { // display errors nicely
messageLine.setText("Couldn't print: " + ex.getMessage());
}
}
}
/**
* This method implements HyperlinkListener. It is invoked when the user
* clicks on a hyperlink, or move the mouse onto or off of a link
**/
public void hyperlinkUpdate(HyperlinkEvent e) {
HyperlinkEvent.EventType type = e.getEventType(); // what happened?
if (type == HyperlinkEvent.EventType.ACTIVATED) { // Click!
displayPage(e.getURL()); // Follow the link; display new page
}
else if (type == HyperlinkEvent.EventType.ENTERED) { // Mouse over!
// When mouse goes over a link, display it in the message line
messageLine.setText(e.getURL().toString());
}
else if (type == HyperlinkEvent.EventType.EXITED) { // Mouse out!
messageLine.setText(" "); // Clear the message line
}
}
/**
* This method implements java.beans.PropertyChangeListener. It is
* invoked whenever a bound property changes in the JEditorPane object.
* The property we are interested in is the "page" property, because it
* tells us when a page has finished loading.
**/
public void propertyChange(PropertyChangeEvent e) {
if (e.getPropertyName().equals("page")) // If the page property changed
stopAnimation();
// Then stop the loading... animation
}
/**
* The fields and methods below implement a simple animation in the
* web browser message line; they are used to provide user feedback
* while web pages are loading.
**/
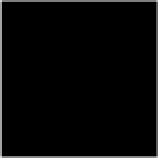
