Java Reference
In-Depth Information
8.5.1 Non-interacting threads
The simplest situation with multiple threads is when each thread runs indepen-
dently without interacting with any other thread. Below is a simple example of
such a case. We have one
Thread
subclass called
IntCounter
that prints out
the values of an integer counter. We could do a more interesting calculation but
for demonstration purposes this will suffice.
/** Demo Thread class to show how threads could do
* calculations in parallel. **/
class
IntCounter
extends Thread
{
int fId=0;
int fCounter = 0;
int fMaxIter = 0;
Outputable fOutput;
/** Constructor to initialize parameters. **/
IntCounter (int id, Outputable out) {
fId = id;
fMaxIter
=
100000
fOutput
=
out;
}
// ctor
/** Simulate a calculation with an integer sum.**/
public void run ()
{
while (fCounter
<
maxIter) fCounter++;
fOutput.println (
"
Thread
"
+ fId +
"
: sum
="
+ fCounter);
}
} // class IntCounter
The program
NonInteractApplet
,which implements the
Outputable
interface discussed in Chapter 6, provides a text area and “Go” and “Clear” but-
tons (see Figure 8.3). Clicking on “Go” invokes the applet's
start()
method,
which creates three instances of this
Thread
subclass and starts them:
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class
NonInteractApplet
extends JApplet
implements Outputable, ActionListener
{
. ..Build interface...
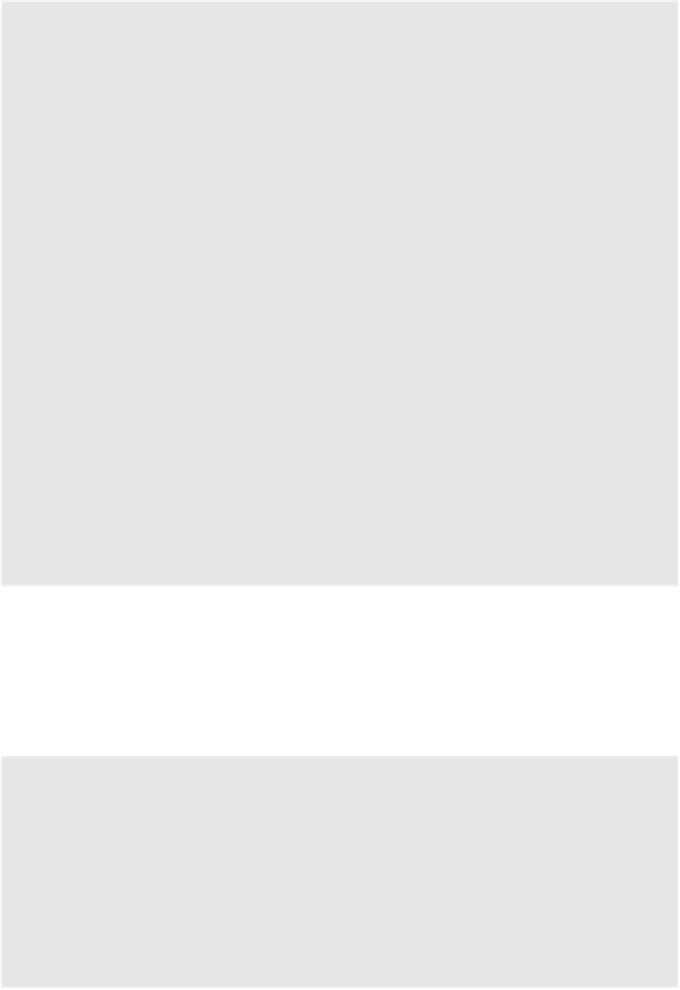
Search WWH ::

Custom Search