Java Reference
In-Depth Information
The switch Statement
You saw earlier how the if and else if statements could be used for checking various conditions; if the
fi rst condition is not valid, then another is checked, and another, and so on. However, when you want
to check the value of a particular variable for a large number of possible values, there is a more effi cient
alternative, namely the switch statement. The structure of the switch statement is given in Figure 3-7.
The best way to think of the switch statement is “Switch to the code where the case matches.” The
switch statement has four important elements:
❑
The test expression
❑
The
case
statements
❑
The
break statements
❑
The
default
statement
Variable expression being checked
These curly braces mark out
the start and end of the switch
statement's case statements.
Checking for possible values.
If a match is found, then execution
starts below the case statement
and ends at the break statement.
switch ( myName )
{
case “Paul”:
// some code
break;
case “John”:
// some other code
break;
default:
//default code
break;
This code executes when none of
the case statements match.
}
Figure 3-7
The test expression is given in the parentheses following the switch keyword. In the previous example,
you are testing using the variable myName. Inside the parentheses, however, you could have any valid
expression.
Next come the case statements. The case statements do the condition checking. To indicate which
case statements belong to your switch statement, you must put them inside the curly braces follow-
ing the test expression. Each case statement specifi es a value, for example “Paul”. The case statement
then acts like if (myName == “Paul”). If the variable myName did contain the value “Paul”, execution
would commence from the code starting below the case “Paul” statement and would continue to the
end of the switch statement. This example has only two case statements, but you can have as many as
you like.
In most cases, you want only the block of code directly underneath the relevant case statement to
execute, not
all
the code below the relevant case statement, including any other case statements.






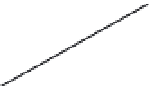



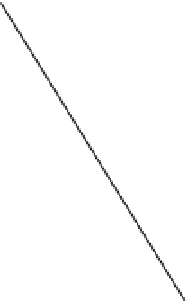

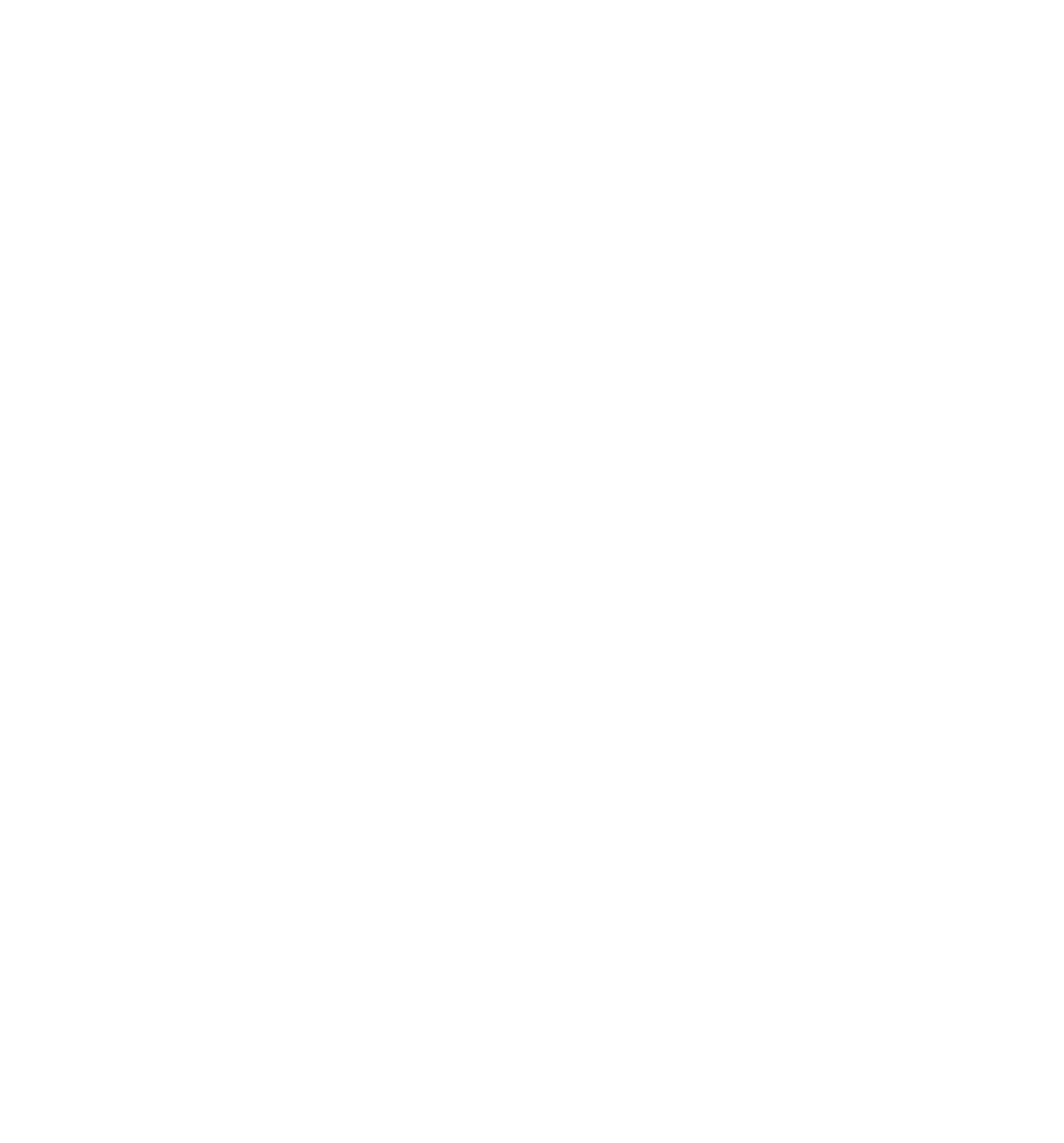




