Java Reference
In-Depth Information
The curly braces, {}, have a special purpose in JavaScript: They mark out a
block
of code. Marking out
lines of code as belonging to a single block means that JavaScript will treat them all as one piece of
code. If the condition of an if statement is true, JavaScript executes the next line or block of code fol-
lowing the if statement. In the preceding example, the block of code has only one statement, so we
could equally as well have written this:
if (roomTemperature > 80)
roomTemperature = roomTemperature - 10;
However, if you have a number of lines of code that you want to execute, you need the braces to mark
them out as a single block of code. For example, a modifi ed version of the example with three statements
of code would have to include the braces.
if (roomTemperature > 80)
{
roomTemperature = roomTemperature - 10;
alert(“It's getting hot in here”);
alert(“Air conditioning switched on”);
}
A particularly easy mistake to make is to forget the braces when marking out a block of code to be
executed. Instead of the code in the block being executed when the condition is true, you'll fi nd that
only the fi rst line
after the if statement is executed. However, the other lines will always be executed
regardless of the outcome of the test condition. To avoid mistakes like these, it's a good idea to always
use braces, even where there is only one statement. If you get into this habit, you'll be less likely to leave
them out when they are actually needed.
Try It Out The if Statement
Let's return to the temperature converter example from Chapter 2 and add some decision-making
functionality.
1.
Enter the following code and save it as
ch3_examp1.htm
:
<!DOCTYPE html PUBLIC “-//W3C//DTD XHTML 1.0 Transitional//EN”
“http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd”>
<html xmlns=”http://www.w3.org/1999/xhtml”>
<body>
<script type=”text/javascript”>
var degFahren = Number(prompt(“Enter the degrees Fahrenheit”,32));
var degCent;
degCent = 5/9 * (degFahren - 32);
document.write(degFahren + “\xB0 Fahrenheit is “ + degCent +
“\xB0 centigrade<br />“);
if (degCent < 0)
{
document.write(“That's below the freezing point of water”);




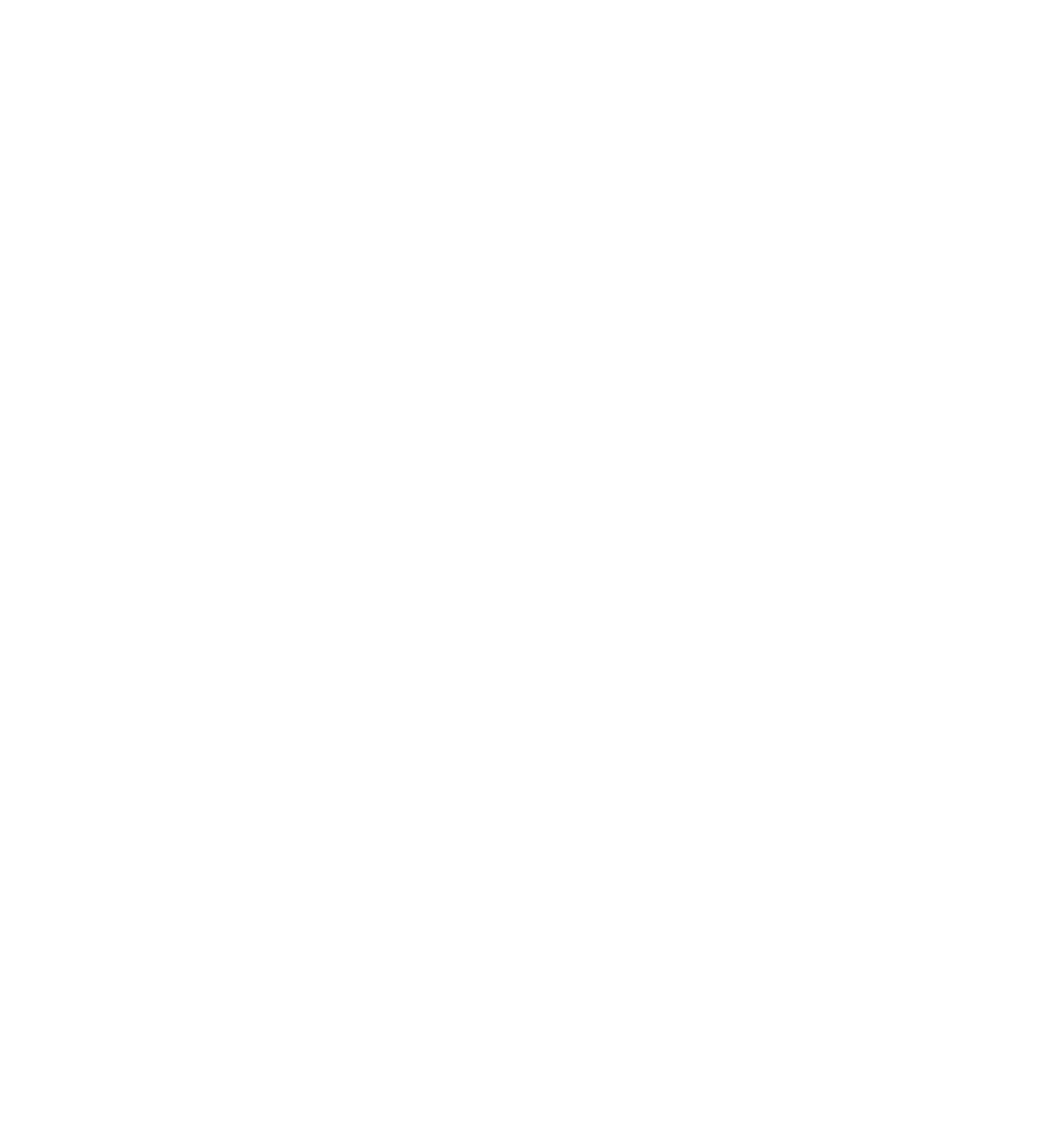



