Java Reference
In-Depth Information
var namesArray = new Array();
while ( (inputName = prompt(“Enter a name”,”“)) != “” )
{
namesArray[namesArray.length] = inputName;
}
namesArray.sort();
var namesList = namesArray.join(“<br/>”)
document.write(namesList);
</script>
</body>
</html>
Save this as
ch05_q2.htm
.
First you declare two variables:
inputName
, which will hold the name entered by the user, and
namesArray
, which holds an
Array
object that stores each of the names entered.
You use a
while
loop to keep getting another name from the user as long as the user hasn't left the
prompt box blank. Note that the use of parentheses in the
while
condition is essential. By placing the
following code inside parentheses, you ensure that this is executed fi rst and that a name is obtained
from the user and stored in the
inputName
variable.
(inputName = prompt(“Enter a name”,””))
Then you compare the value returned inside the parentheses — whatever was entered by the user —
with an empty string (denoted by
“”
). If they are not equal — that is, if the user did enter a value, you
loop around again.
Now, to sort the array into order, you use the
sort()
method of the
Array
object.
namesArray.sort();
Finally, to create a string containing all values contained in the array elements with each being on a
new line, you use the HTML
<br/>
element and write the following:
var namesList = namesArray.join(“<br/>”)
document.write(namesList);
The code
namesArray.join(“<br/>”)
creates the string of array elements with a
<br/>
between
each. Finally, you write the string into the page with
document.write()
.
Exercise 3 Question
In this chapter, you learned how you can use the
pow()
method inventively to fi x a number to a certain
number of decimal places. However, there is a fl aw in the function you created. A proper
fix()
function
should return
2.1
fi xed to three decimal places like this:
2.100


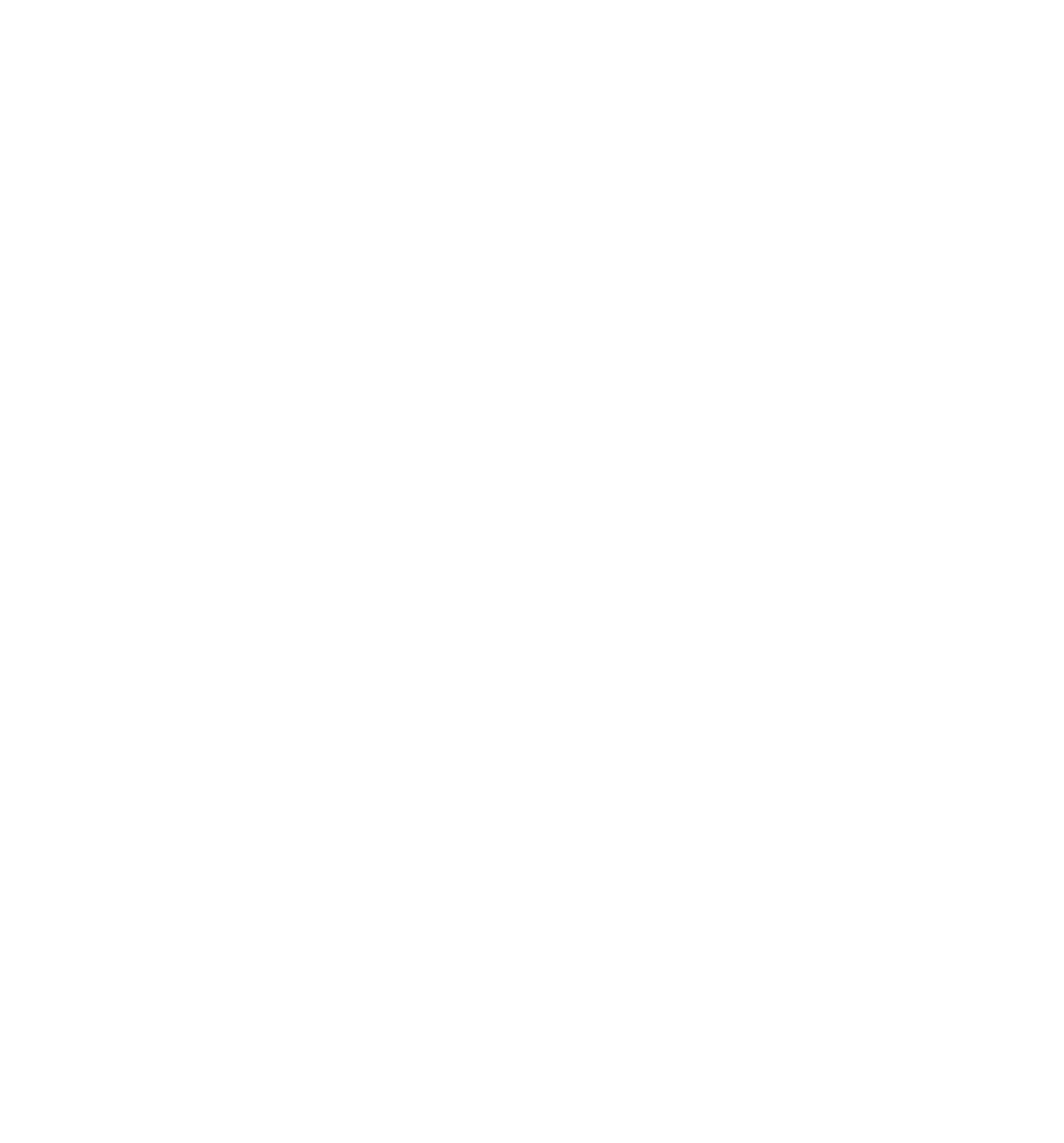



