Java Reference
In-Depth Information
alert(“The server returned a status code of ” + oHttp.status);
}
}
}
oHttp.open(“GET”, “http://localhost/myTextFile.txt”, true);
oHttp.onreadystatechange = oHttp_readyStateChange;
oHttp.send(null);
This code fi rst defi nes the
oHttp_readyStateChange()
function, which handles the
readystatechange
event; it fi rst checks if the request completed by comparing
readyState
to
4
. The function then checks
the request's status to make sure the server returned the requested data. Once these two criteria are met,
the code alerts the value of the
responseText
property (the actual requested data in plain text format).
Note the
open()
method's call; the fi nal argument passed to the method is
true
. This makes the
XMLHttpRequest
object request data asynchronously.
The benefi ts of using asynchronous communication are well worth the added complexity of the
readystatechange
event, as the browser can continue to load the page and execute your other JavaScript
code while the request object sends and receives data. Perhaps a user-defi ned module that wraps an
XMLHttpRequest
object could make asynchronous requests easier to use and manage.
An
XMLHttpRequest
object also has a property called
responseXML,
which attempts to load the
received data into an XML DOM (whereas
responseText
returns plain text). This is the only way
Safari 2 can load XML data into a DOM.
Creating a Simple Ajax Module
The concept of code reuse is important in programming; it is the reason why functions are defi ned to
perform specifi c, common, and repetitive tasks. Chapter 5 introduced you to the object-oriented construct
of code reuse: reference types. These constructs contain properties that contain data and/or methods that
perform actions with that data.
In this section, you write your own Ajax module called
HttpRequest
, thereby making asynchronous
requests easier to make and manage. Before getting into writing this module, let's go over the proper-
ties and methods the
HttpRequest
reference type exposes.
Planning the HttpRequest Module
There's only one piece of information that you need to keep track of: the underlying
XMLHttpRequest
object. Therefore, this module will have only one property:
request
, which contains the underlying
XMLHttpRequest
object.
The methods are equally easy to identify.
❑
— Creates the
XMLHttpRequest
object for all supporting browsers.
It is essentially a copy of the function of the same name written earlier in the chapter.
createXmlHttpRequest()
❑
send()
— Sends the request to the server.


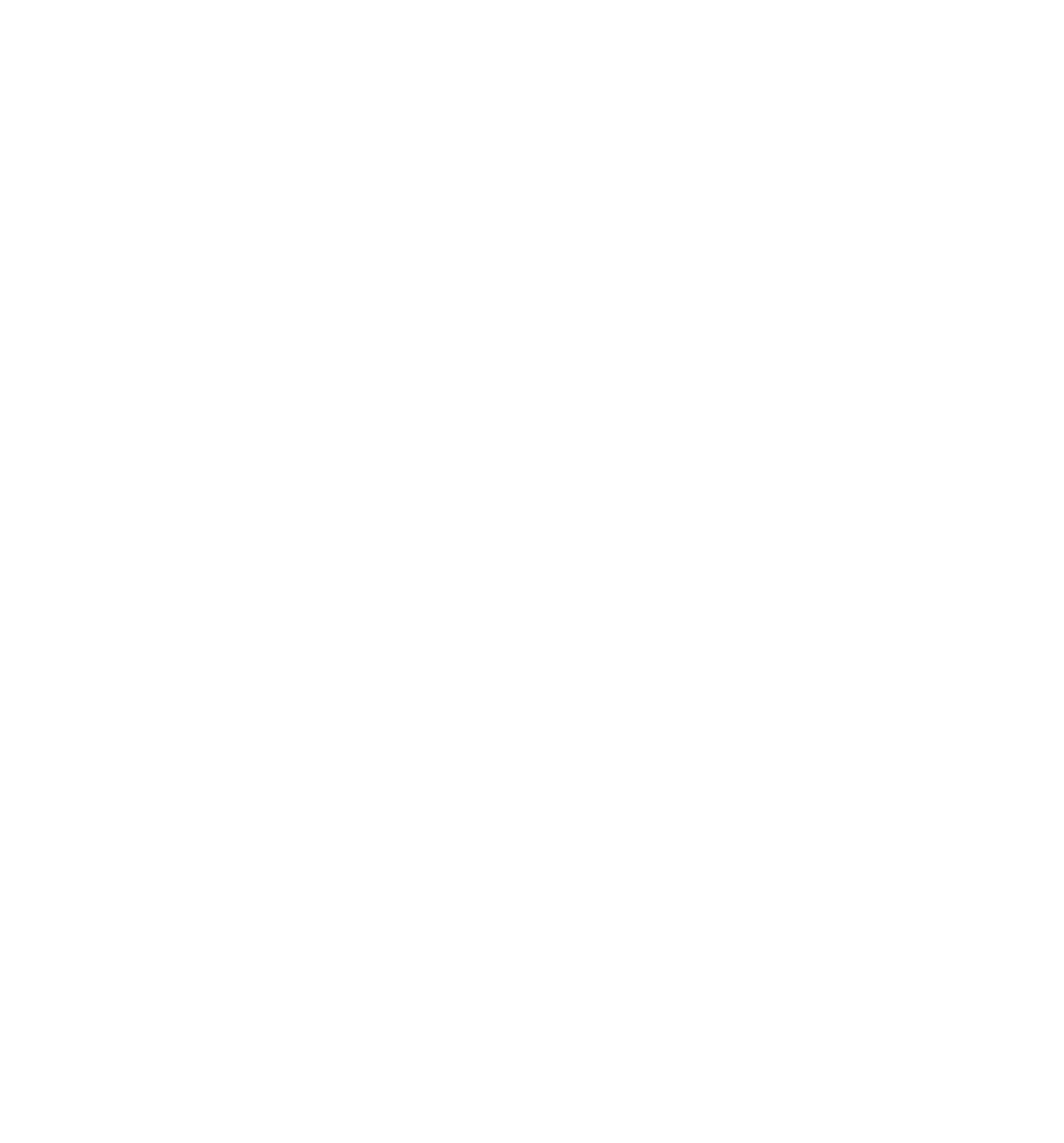



