Java Reference
In-Depth Information
xmlDocument.load(“ch12_examp11.xml”);
The workhorse of this page is the next function,
displayDogs()
. Its job is to build a table and populate
it with the information from the XML fi le.
function displayDogs()
{
var dogNodes = xmlDocument.getElementsByTagName(“dog”);
var table = document.createElement(“table”);
table.setAttribute(“cellPadding”,5); //Give the table some cell padding.
table.setAttribute(“width”, “100%”);
table.setAttribute(“border”, “1”);
The fi rst thing this function does is use the getElementsByTagName() method to retrieve the <dog/>
elements and assign the resulting node list to dogNodes. Next, create a <table/> element by using the
document.createElement() method, and set its cellPadding, width, and border attributes using
setAttribute().
Next, create the table header and heading cells. For the column headers, use the element names of the
<dog/>
element's children (
name
,
breed
,
age
, and so on).
var tableHeader = document.createElement(“thead”);
var tableRow = document.createElement(“tr”);
for (var i = 0; i < dogNodes[0].childNodes.length; i++)
{
var currentNode = dogNodes[0].childNodes[i];
//Loop code here.
}
tableHeader.appendChild(tableRow);
table.appendChild(tableHeader);
The fi rst few lines of this code create <thead/> and <tr/> elements. Then the code loops through the
fi rst <dog/> element's child nodes (more on this later). After the loop, append the <tr/> element to the
table header and add the header to the table. Now let's look at the loop.
for (var i = 0; i < dogNodes[0].childNodes.length; i++)
{
var currentNode = dogNodes[0].childNodes[i];
if (currentNode.nodeType == 1)
{
var tableHeaderCell = document.createElement(“th”);
var textData = document.createTextNode(currentNode.nodeName);
tableHeaderCell.appendChild(textData);
tableRow.appendChild(tableHeaderCell);
}
}
The goal is to use the element names as headers for the column. However, you're looping through
every child node of a
<dog/>
element, so any instance of whitespace between elements is counted as a
child node in DOM supported browsers. To solve this problem, check the current node's type with the


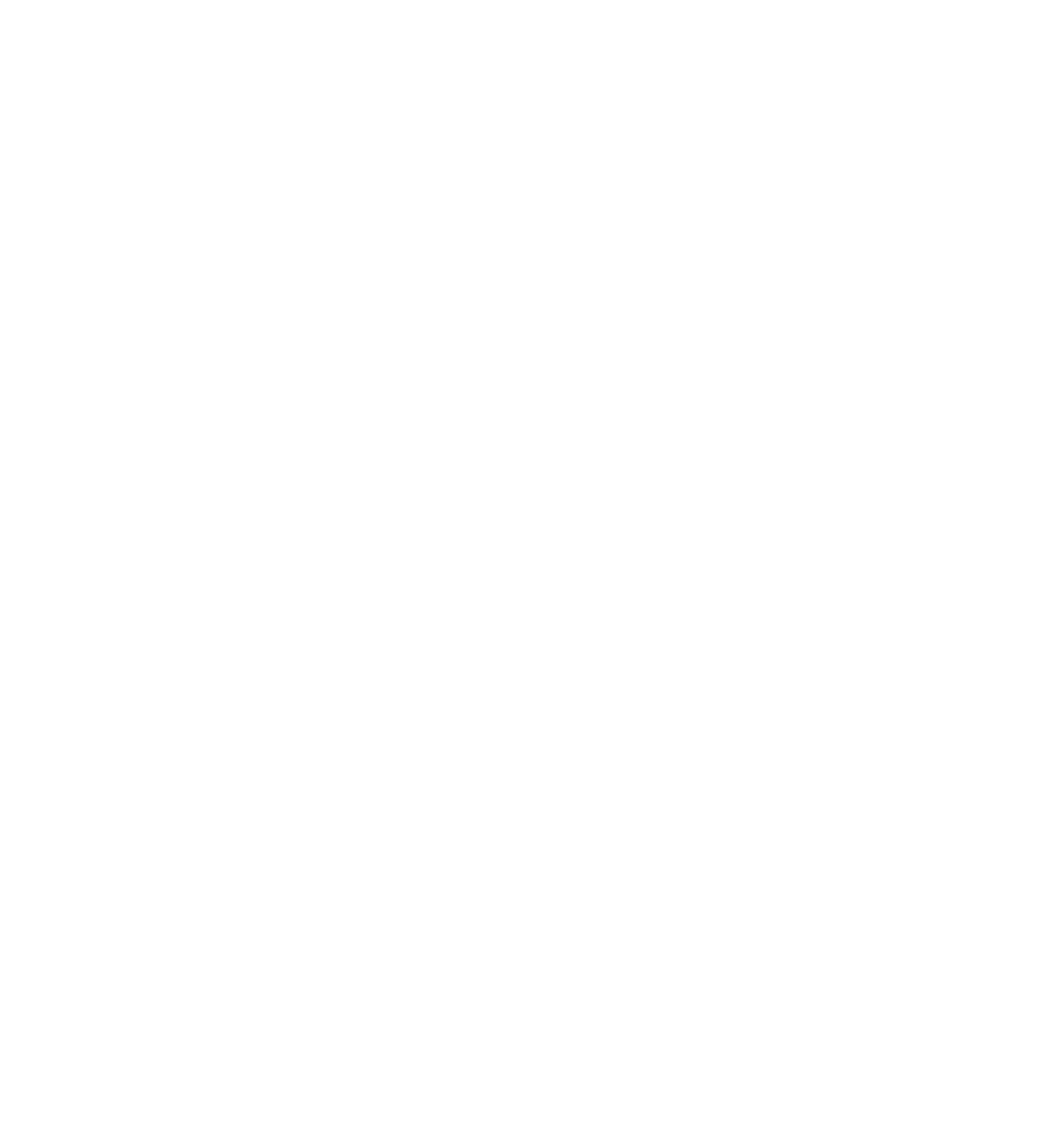



