Java Reference
In-Depth Information
And when you move your mouse off of the text, it returns to normal.
In the page's body, a
<div/>
element is defi ned with an
id
of
divAdvert
. Hook up the
mouseover
and
mouseout
events to the
divAdvert_onMouseOver()
and
divAdvert_onMouseOut()
functions,
respectively, which are defi ned in the
<script/>
block in the head of the page.
When the mouse pointer enters the <div/> element, the divAdvert_onMouseOver() function is
called.
function divAdvert_onMouseOver()
{
var divAdvert = document.getElementById(“divAdvert”);
divAdvert.style.fontStyle = “italic”;
divAdvert.style.textDecoration = “underline”;
}
Before you can do anything to the
<div/>
element, you must fi rst retrieve it. You do this simply by using the
getElementById()
method. Now that you have the element, you manipulate its style by fi rst italicizing
the text with the
fontStyle
property. Next, you underline the text by using the
textDecoration
property
and assigning its value to
underline
.
Naturally, you do not want to keep the text italicized and underlined; so the mouseout event allows you
to change the text back to its original state. When this event fi res, the divAdvert_onMouseOut() func-
tion is called.
function divAdvert_onMouseOut()
{
var divAdvert = document.getElementById(“divAdvert”);
divAdvert.style.fontStyle = “normal”;
divAdvert.style.textDecoration = “none”;
}
The code for this function resembles the code for the
divAdvert_onMouseOver()
function. First, you
retrieve the
divAdvert
element and then set the
fontStyle
property to
normal
, thus removing the
italics. Then you set the
textDecoration
to
none
, which removes the underline from the text.
Changing the class Attribute
You can assign a CSS class to elements by using the element's
class
attribute. This attribute is exposed
in the DOM by the
className
property and can be changed through JavaScript to associate a different
style rule with the element.
element.className = sNewClassName;
Using the
className
property to change an element's style is advantageous in two ways:
❑
It reduces the amount of JavaScript you have to write, which no one is likely to complain about.
❑
It keeps style information out of the JavaScript fi le and puts it into the CSS fi le where it belongs.
Making any type of changes to the style rules is easier because you do not have to have several
fi les open in order to change them.


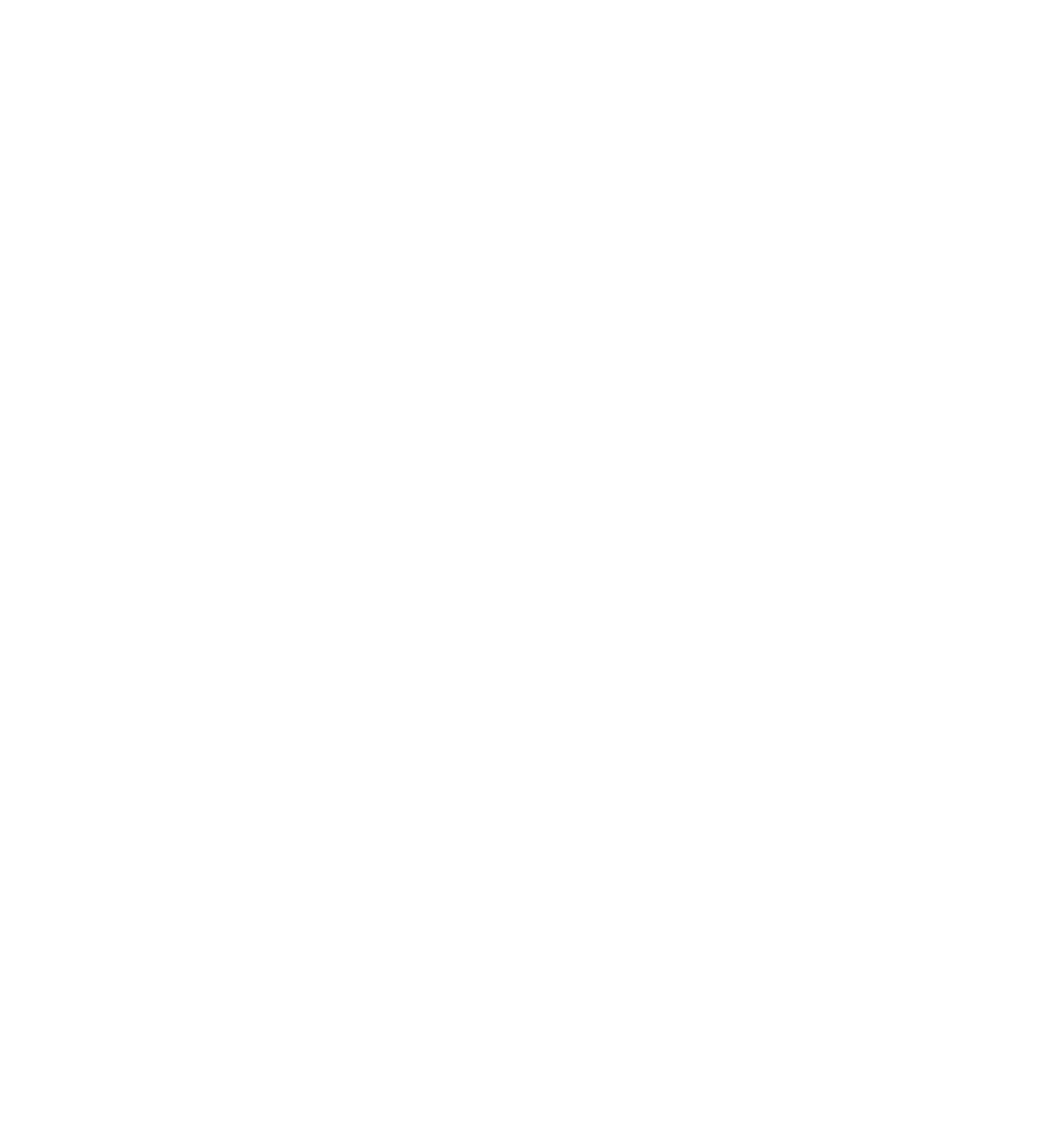





