Java Reference
In-Depth Information
The replace() Method
You've already looked at the syntax and usage of the replace() method. However, something unique
to the replace() method is its ability to replace text based on the groups matched in the regular expres-
sion. You do this using the $ sign and the group's number. Each group in a regular expression is given a
number from 1 to 99; any groups greater than 99 are not accessible. Note that in earlier browsers, groups
could only go from 1 to 9 (for example, in IE 5 or earlier or Netscape 4 and earlier). To refer to a group, you
write $ followed by the group's position. For example, if you had the following:
var myRegExp = /(\d)(\W)/g;
then $1 refers to the group(\d), and $2 refers to the group (\W). You've also set the global fl ag g to
ensure that all matching patterns are replaced — not just the fi rst one.
You can see this more clearly in the next example. Say you have the following string:
var myString = “1999, 2000, 2001”;
If you wanted to change this to “the year 1999, the year 2000, the year 2001”, how could you do
it with regular expressions?
First, you need to work out the pattern as a regular expression, in this case four digits.
var myRegExp = /\d{4}/g;
But given that the year is different every time, how can you substitute the year value into the replaced
string?
Well, you change your regular expression so that it's inside a group, as follows:
var myRegExp = /(\d{4})/g;
Now you can use the group, which has group number 1, inside the replacement string like this:
myString = myString.replace(myRegExp, “the year $1”);
The variable myString now contains the required string “the year 1999, the year 2000, the year
2001”.
Let's look at another example in which you want to convert single quotes in text to double quotes. Your
test string is this:
'Hello World' said Mr. O'Connerly.
He then said 'My Name is O'Connerly, yes that's right, O'Connerly'.
One problem that the test string makes clear is that you want to replace the single-quote mark with a
double only where it is used in pairs around speech, not when it is acting as an apostrophe, such as in
the word that's, or when it's part of someone's name, such as in O'Connerly.


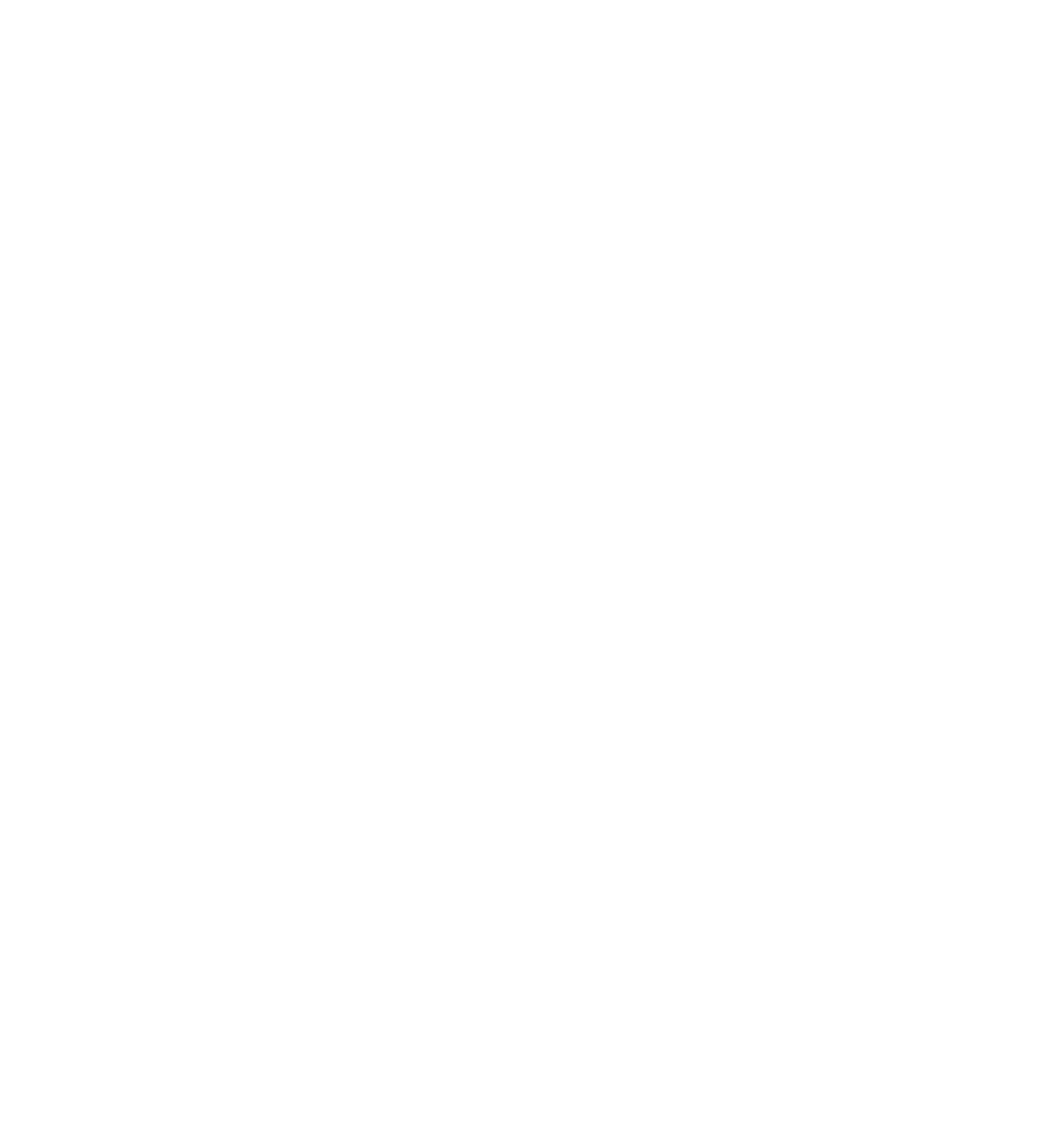



