Java Reference
In-Depth Information
Having confi rmed that a window has been opened at some point, you now need to check whether it's
still open, and the window object's closed property does just that. If it returns true, the window is
closed, and if it returns false, it's still open. (Do not confuse this closed property with the close()
method you saw previously.)
In the
if
statement, you'll see that checking if
newWindow
is defi ned comes fi rst, and this is no accident.
If
newWindow
really were undefi ned,
newWindow.closed
would cause an error, because there are no
data inside
newWindow
. However, you are taking advantage of the fact that if an
if
statement's condition
will be
true
or
false
at a certain point regardless of the remainder of the condition, the remainder of
the condition is not checked.
function butGetText_onclick()
{
if (typeof(newWindow) == “undefined” || newWindow.closed == true)
{
alert(“No window is open”);
}
If newWindow exists and is open, the else statement's code will execute. Remember that newWindow
will contain a reference to the window object of the window opened. This means you can access the
form in newWindow, just as you'd access a form on the page the script's running in, by using the docu-
ment object inside the newWindow window object.
else
{
document.form1.text1.value = newWindow.document.form1.text1.value;
}
}
The last of the three functions is
window_onunload()
, which is connected to the
onunload
event of this
page and fi res when either the browser window is closed or the user navigates to another page. In the
window_onunload()
function, you check to see if
newWindow
is valid and open in much the same way
that you just did. You must check to see if the
newWindow
variable is defi ned fi rst. With the
&&
operator,
JavaScript checks the second part of the operation only if the fi rst part evaluates to
true
. If
newWindow
is defi ned, and does therefore hold a
window
object (even though it's possibly a closed window), you can
check the
closed
property of the window. However, if
newWindow
is undefi ned, the check for its
closed
property won't happen, and no errors will occur. If you check the
closed
property fi rst and
newWindow
is undefi ned, an error will occur, because an undefi ned variable has no
closed
property.
function window_onunload()
{
if (typeof(newWindow) != “undefined” && newWindow.closed == false)
{
newWindow.close();
}
}
If newWindow is defi ned and open, you close it. This prevents the newWindow's Get Text button from
being clicked when there is no opener window in existence to get text from (since this function fi res
when the opener window is closed).


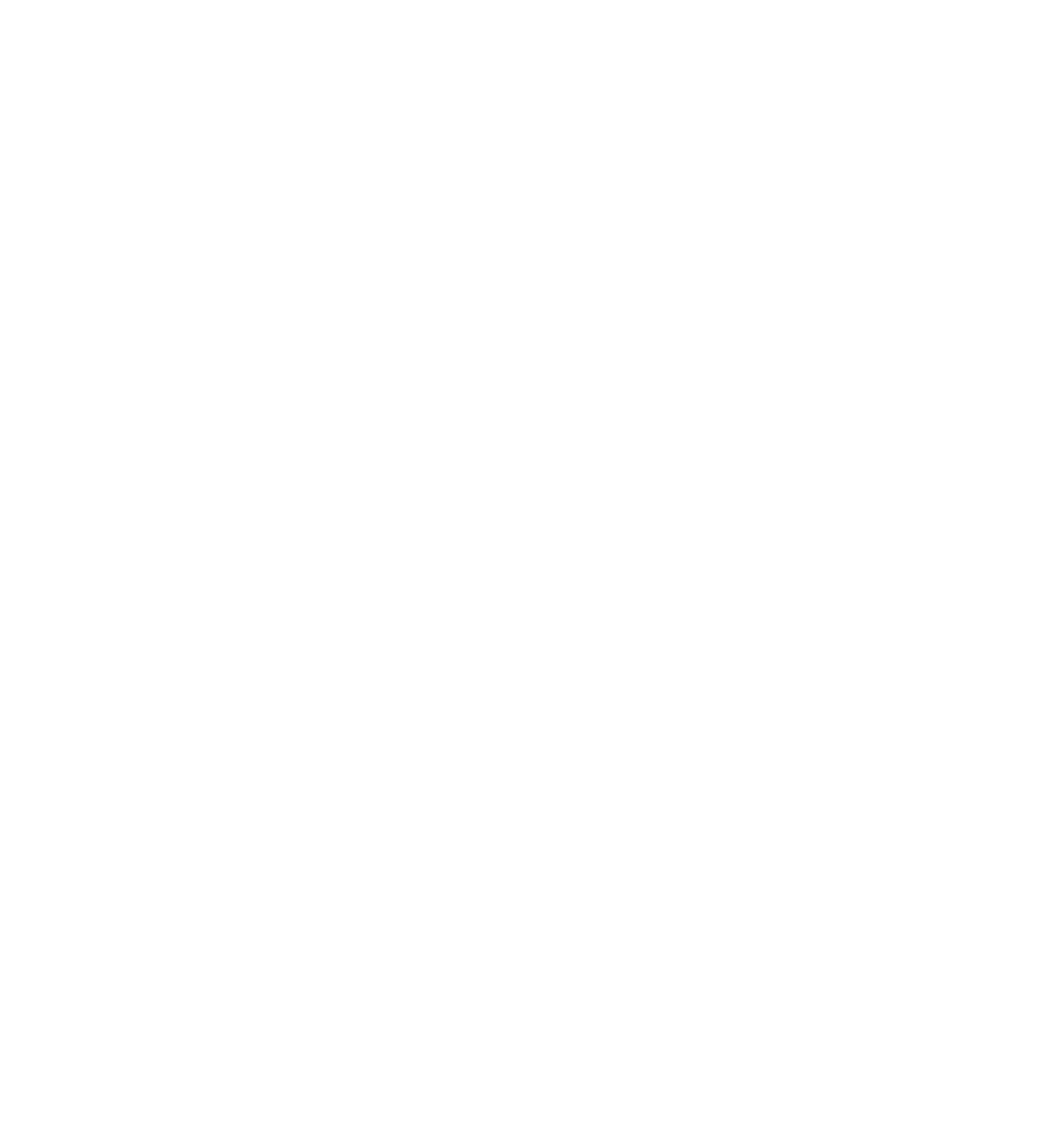



