Java Reference
In-Depth Information
array. The function these methods use must follow one rule. The function must accept three arguments
like the following code.
function functionName(value, index, array) {
// do something here
}
When this function is executed, JavaScript passes three arguments to your function. The fi rst is the
value of the element, next is the index of the element, and fi nally is the array itself. With these param-
eters, you should be able to perform any operation or comparison you need.
Testing Each Element — The every(), some(), and f lter() Methods
Let's look at the every() and some() methods fi rst. These are testing methods. The every() method
tests whether all elements in the array pass the test in your function. Consider the following code:
var numbers = new Array(1, 2, 3, 4, 5);
function isLessThan3(value, index, array)
{
var returnValue = false;
if (value < 3)
{
returnValue = true;
}
return returnValue;
}
alert(numbers.every(isLessThan3));
The fi rst line shows the creation of an array called numbers; its elements hold the values 1 through
5. The next line defi nes the isLessThan3() function. It accepts the three mandatory arguments and
determines if the value of each element is less than 3. The last line alerts the outcome of the every()
test. Because not every value in the array is less than 3, the result of the every() test is false.
Contrast this with the some() method. Unlike every(), the some() test only cares if some of the ele-
ments pass the test in your function. Using the same numbers array and isLessThan3() function, con-
sider this line of code:
alert(numbers.some(isLessThan3));
The result is true because some of the elements in the array are less than 3. It's easy to keep these two
methods straight. Just remember the every() method returns true if, and only if, all elements in the
array pass the test in your function; the some() method returns true if, and only if, some of the ele-
ments in the array pass your function's test.
Let's assume you want to retrieve the elements that have a value less than 3. You already know some
elements meet this criterion, but how do you identify those elements and retrieve them? This is where
the filter() method becomes useful.


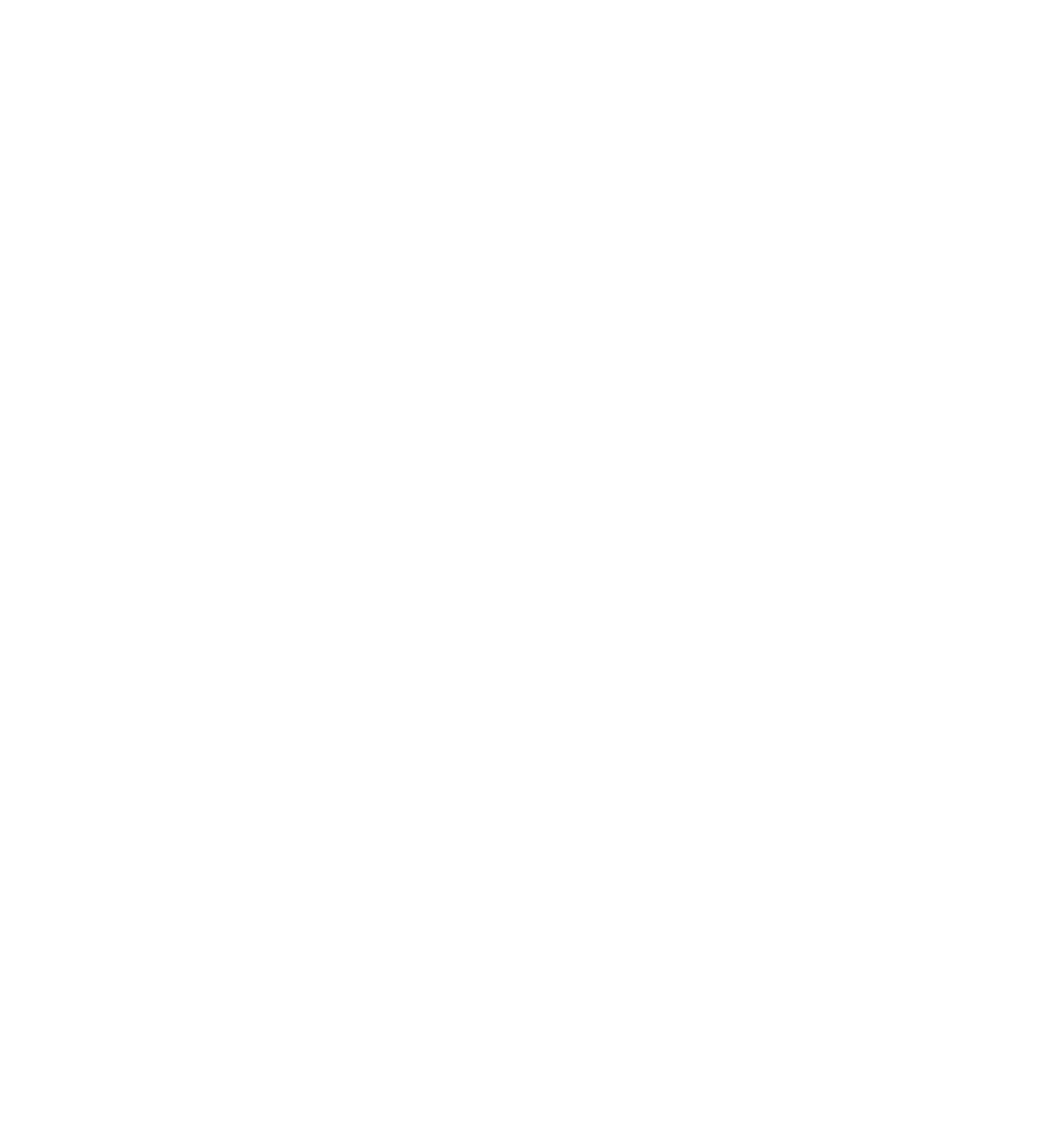



