Java Reference
In-Depth Information
numbers rather than actual letters. It just happens that Unicode numbers match the order in the alphabet.
However, lowercase letters are given a different sequence of numbers, which come after the uppercase
letters. So the array with elements Adam, adam, Zoë, zoë, will be sorted to the order Adam, Zoë, adam, zoë.
Note that in your for statement you've used the Array object's length property in the condition state-
ment, rather than inserting the length of the array (5), like this:
for (elementIndex = 0; elementIndex < 5; elementIndex++)
Why do this? After all, you know in advance that there are fi ve elements in the array. Well, what would
happen if you altered the number of elements in the array by adding two more names?
var names = new Array(“Paul”,”Sarah”,”Jeremy”,”Adam”,”Bob”,”Karen”,”Steve”);
If you had inserted 5 rather than names.length, your loop code wouldn't work as you want it to. It
wouldn't display the last two elements unless you changed the condition part of the for loop to 7. By
using the length property, you've made life easier, because now there is no need to change code else-
where if you add array elements.
Okay, you've put things in ascending order, but what if you wanted descending order? That is where
the reverse() method comes in.
Putting Your Array into Reverse Order — The reverse() Method
The fi nal method you'll look at for the Array object is the reverse() method, which, no prizes for
guessing, reverses the order of the array so that the elements at the back are moved to the front. Let's
take the shopping list again as an example.
Index
0
1
2
3
4
Va lue
Eggs
Milk
Potatoes
Cereal
Banana
If you use the
reverse()
method
var myShopping = new Array(“Eggs”,”Milk”,”Potatoes”,”Cereal”,”Banana”);
myShopping.reverse();
you end up with the array elements in this order:
Index
0
1
2
3
4
Va lue
Banana
Cereal
Potatoes
Milk
Eggs
To prove this, you could write it to the page with the
join()
method you saw earlier.
var myShoppingList = myShopping.join(“<br />”)
document.write(myShoppingList);




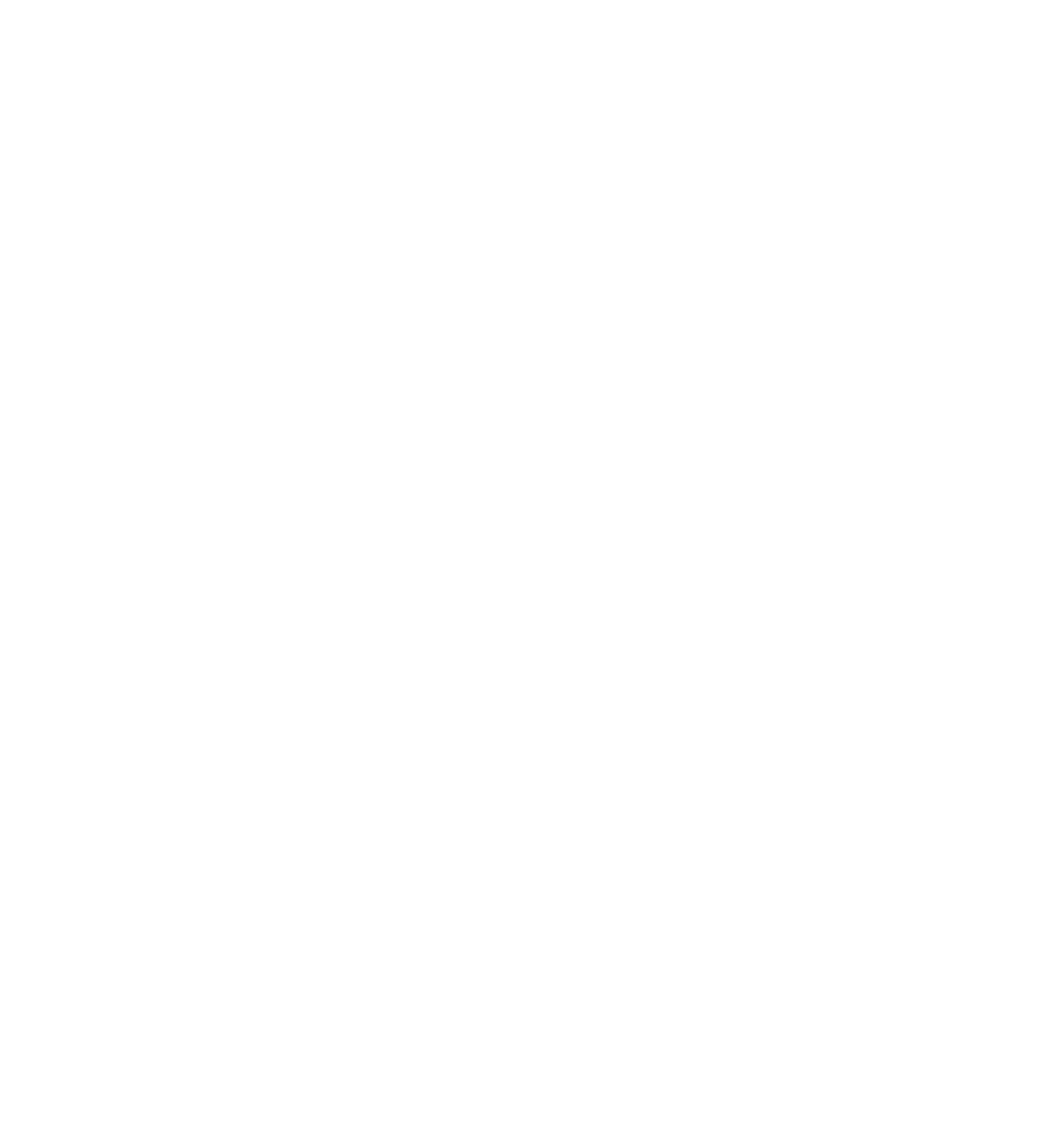



