Java Reference
In-Depth Information
break;
case “N”:
document.write(“First character was a number”);
break;
default:
document.write(“First character was not a character or a number”);
}
That completes the example, but before moving on, it's worth noting that this example is just that — an
example of using
charCodeAt()
. In practice, it would be much easier to just write
if (char >= “A” && char <= “Z”)
rather than
if (charCode >= “A”.charCodeAt(0) && charCode <= “Z”.charCodeAt(0))
which you have used here.
Converting Character Codes to a String — The fromCharCode() Method
The method
fromCharCode()
can be thought of as the opposite of
charCodeAt()
, in that you pass it a
series of comma-separated numbers representing character codes, and it converts them to a single string.
However, the
fromCharCode()
method is unusual in that it's a
static
method — you don't need to have
created a
String
object to use it with, it's always available to you.
For example, the following lines put the string
“ABC”
into the variable
myString
:
var myString;
myString = String.fromCharCode(65,66,67);
The
fromCharCode()
method can be very useful when used with variables. For example, to build up a
string consisting of all the uppercase letters of the alphabet, you could use the following code:
var myString = “”;
var charCode;
for (charCode = 65; charCode <= 90; charCode++)
{
myString = myString + String.fromCharCode(charCode);
}
document.write(myString);
You use the
for
loop to select each character from A to Z in turn and concatenate this to
myString
.
Note that while this is fi ne as an example, it is more effi cient and less memory-hungry to simply write
this instead:
var myString = “ABCDEFGHIJKLMNOPQRSTUVWXYZ”;


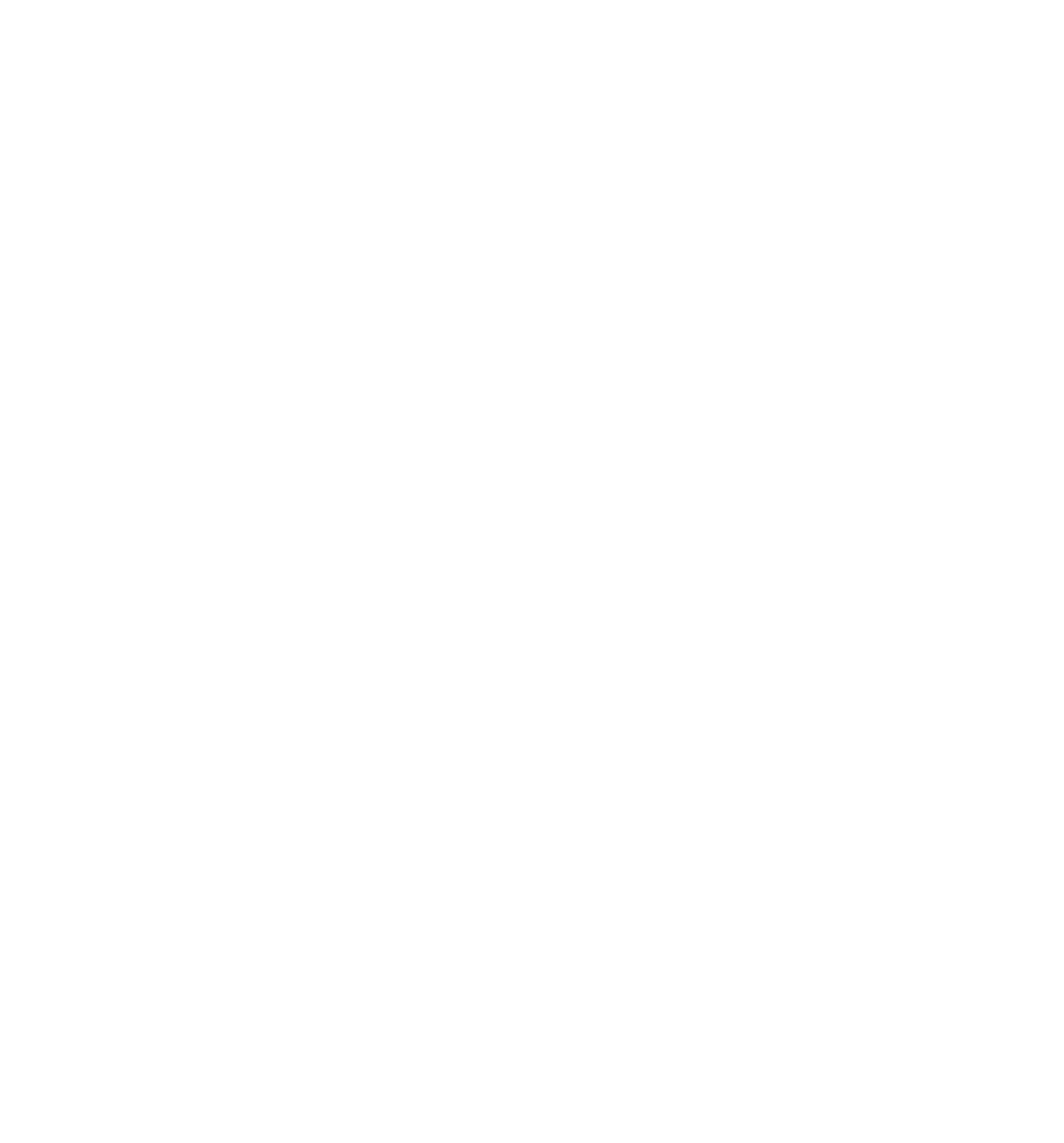





