Java Reference
In-Depth Information
document.write(“Too high!”);
break;
default:
document.write(“You did not enter a number between 1 and 5.”);
break;
}
If you load this into your browser and experiment with entering some different numbers, you should
see that it behaves exactly like the previous code.
Here, you are making use of the fact that if there is no
break
statement underneath the code for a certain
case
statement, execution will continue through each following
case
statement until a
break
statement
or the end of the
switch
is reached. Think of it as a sort of free fall through the
switch
statement until
you hit the
break
statement.
If the
case
statement for the value
1
is matched, execution simply continues until the
break
statement
under
case
2
, so effectively you can execute the same code for both cases. The same technique is used
for the
case
statements with values
4
and
5
.
Looping — The for and while Statements
Looping
means repeating a block of code when a condition is
true
. This is achieved in JavaScript with
the use of two statements, the
while
statement and the
for
statement. You'll be looking at these shortly,
but why would you want to repeat blocks of code anyway?
Well, take the situation where you have a series of results, say the average temperature for each month
in a year, and you want to plot these on a graph. The code needed for plotting each point will most likely
be the same. So, rather than write the code 12 times (once for each point), it's much easier to execute the
same code 12 times by using the next item of data in the series. This is where the
for
statement would
come in handy, because you know how many times you want the code to execute.
In another situation, you might want to repeat the same piece of code when a certain condition is
true
,
for example, while the user keeps clicking a Start Again button. In this situation, the
while
statement
would be very useful.
The for Loop
The
for
statement enables you to repeat a block of code a certain number of times. The syntax is illus-
trated in Figure 3-10.
Let's look at the makeup of a
for
statement. You can see from Figure 3-10 that, just like the
if
and
switch
statements, the
for
statement also has its logic inside parentheses. However, this time that logic split
into three parts, each part separated by a semicolon. For example, in Figure 3-10 you have the following:
(var loopCounter = 1; loopCounter <= 3; loopCounter++)



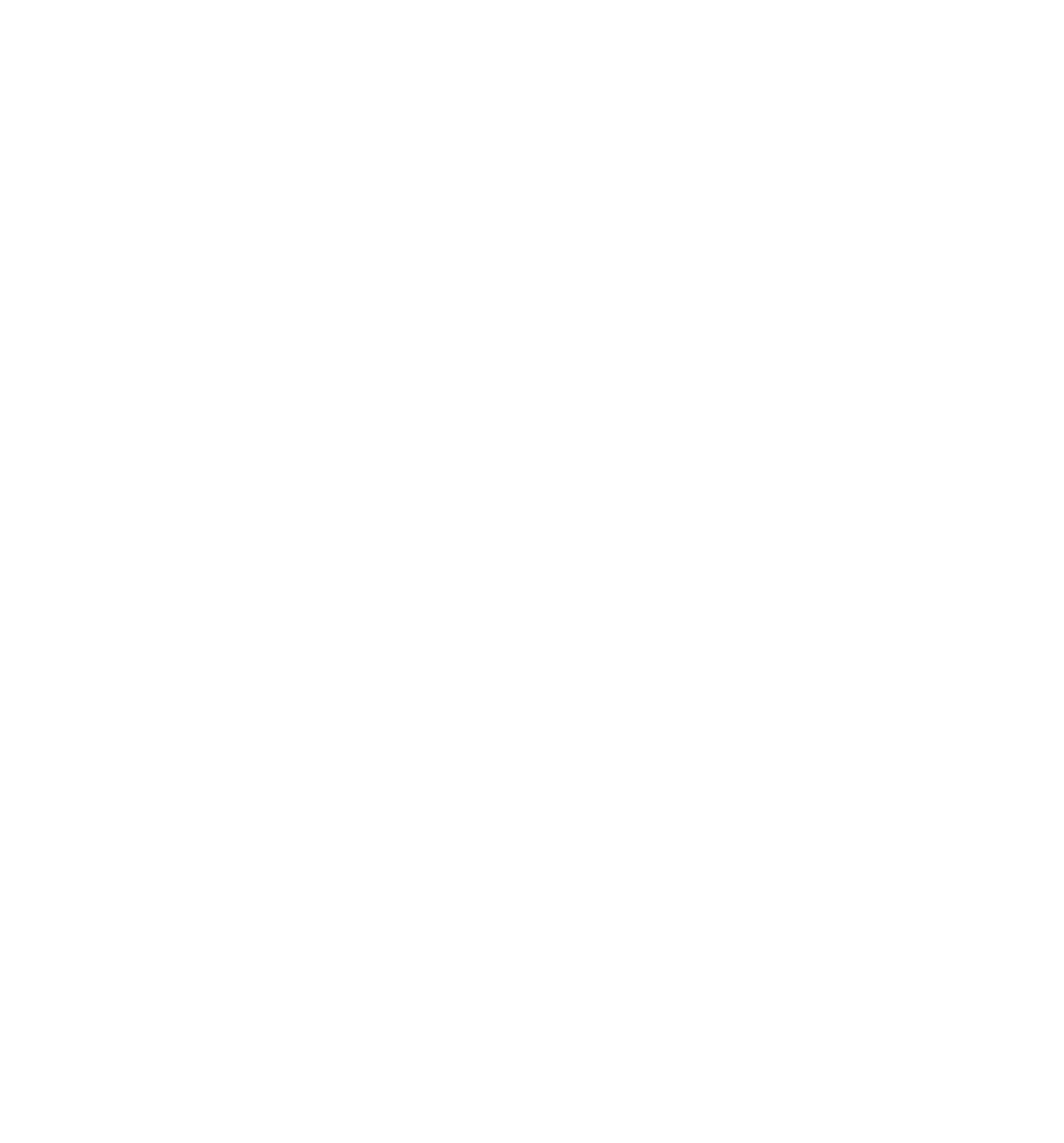



