Java Reference
In-Depth Information
the
orderTotal
instance variable itself holds an object, and that object has its own
instance variable called
layaway
, you could refer to it as in this statement:
boolean onLayaway = myCustomer.orderTotal.layaway;
Dot expressions are evaluated from left to right, so you start with
myCustomer
's variable
orderTotal
, which points to another object with the variable
layaway
. You end up with
the value of that
layaway
variable.
Changing Values
Assigning a value to that variable is equally easy; just tack on an assignment operator to
the right side of the expression:
myCustomer.orderTotal.layaway = true;
This example sets the value of the
layaway
variable to
true
.
Listing 3.2 is an example of a program that tests and modifies the instance variables in a
Point
object.
Point
, a class in the
java.awt
package, represents points in a coordinate
system with
x
and
y
values.
LISTING 3.2
The Full Text of PointSetter.java
1: import java.awt.Point;
2:
3: class PointSetter {
4:
5: public static void main(String[] arguments) {
6: Point location = new Point(4, 13);
7:
8: System.out.println(“Starting location:”);
9: System.out.println(“X equals “ + location.x);
10: System.out.println(“Y equals “ + location.y);
11:
12: System.out.println(“\nMoving to (7, 6)”);
13: location.x = 7;
14: location.y = 6;
15:
16: System.out.println(“\nEnding location:”);
17: System.out.println(“X equals “ + location.x);
18: System.out.println(“Y equals “ + location.y);
19: }
20: }
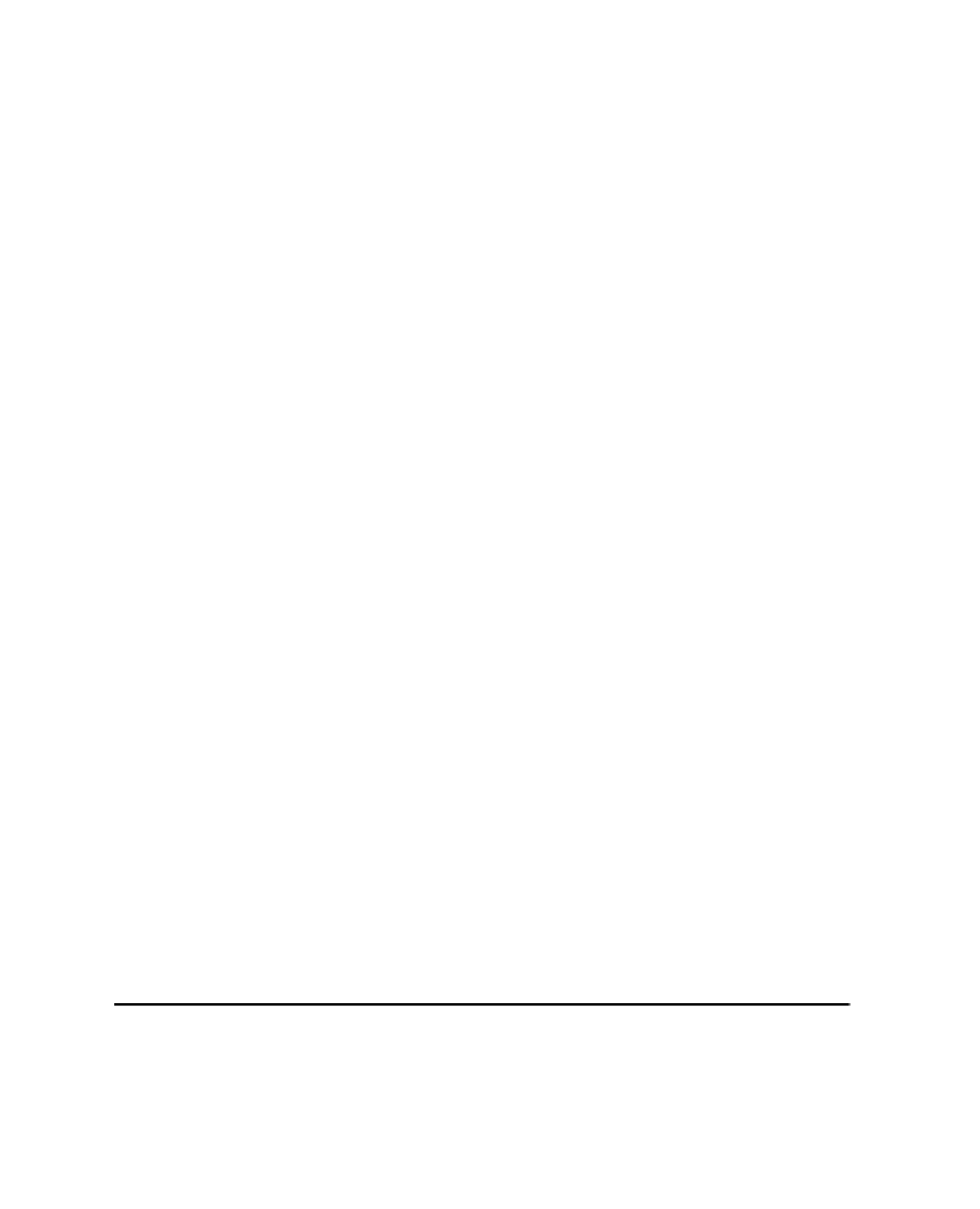