Java Reference
In-Depth Information
The number and type of arguments you can use inside the parentheses with
new
are
defined by the class itself using a special method called a
constructor
. (You'll learn more
about constructors later today.) If you try to create a new instance of a class with the
wrong number or type of arguments (or if you give it no arguments and it needs some),
you'll receive an error when you try to compile your Java program.
Here's an example of creating different types of objects with different numbers and types
of arguments: the
StringTokenizer
class, part of the
java.util
package, divides a
string into a series of shorter strings called
tokens
.
A string is divided into tokens by applying some kind of character or characters as a
delimiter. For example, the text
“02/20/67”
could be divided into three tokens—
02
,
20
,
and
67
—using the slash character (“/”) as a delimiter.
Listing 3.1 is a Java program that creates
StringTokenizer
objects by using
new
in two
different ways and then displays each token the objects contain.
3
LISTING 3.1
The Full Text of
TokenTester.java
1: import java.util.StringTokenizer;
2:
3: class TokenTester {
4:
5: public static void main(String[] arguments) {
6: StringTokenizer st1, st2;
7:
8: String quote1 = “VIZY 3 -1/16”;
9: st1 = new StringTokenizer(quote1);
10: System.out.println(“Token 1: “ + st1.nextToken());
11: System.out.println(“Token 2: “ + st1.nextToken());
12: System.out.println(“Token 3: “ + st1.nextToken());
13:
14: String quote2 = “NPLI@9 27/32@3/32”;
15: st2 = new StringTokenizer(quote2, “@”);
16: System.out.println(“\nToken 1: “ + st2.nextToken());
17: System.out.println(“Token 2: “ + st2.nextToken());
18: System.out.println(“Token 3: “ + st2.nextToken());
19: }
20: }
When you compile and run the program, the output should resemble the following:
Token 1: VIZY
Token 2: 3
Token 3: -1/16
Token 1: NPLI
Token 2: 9 27/32
Token 3: 3/32

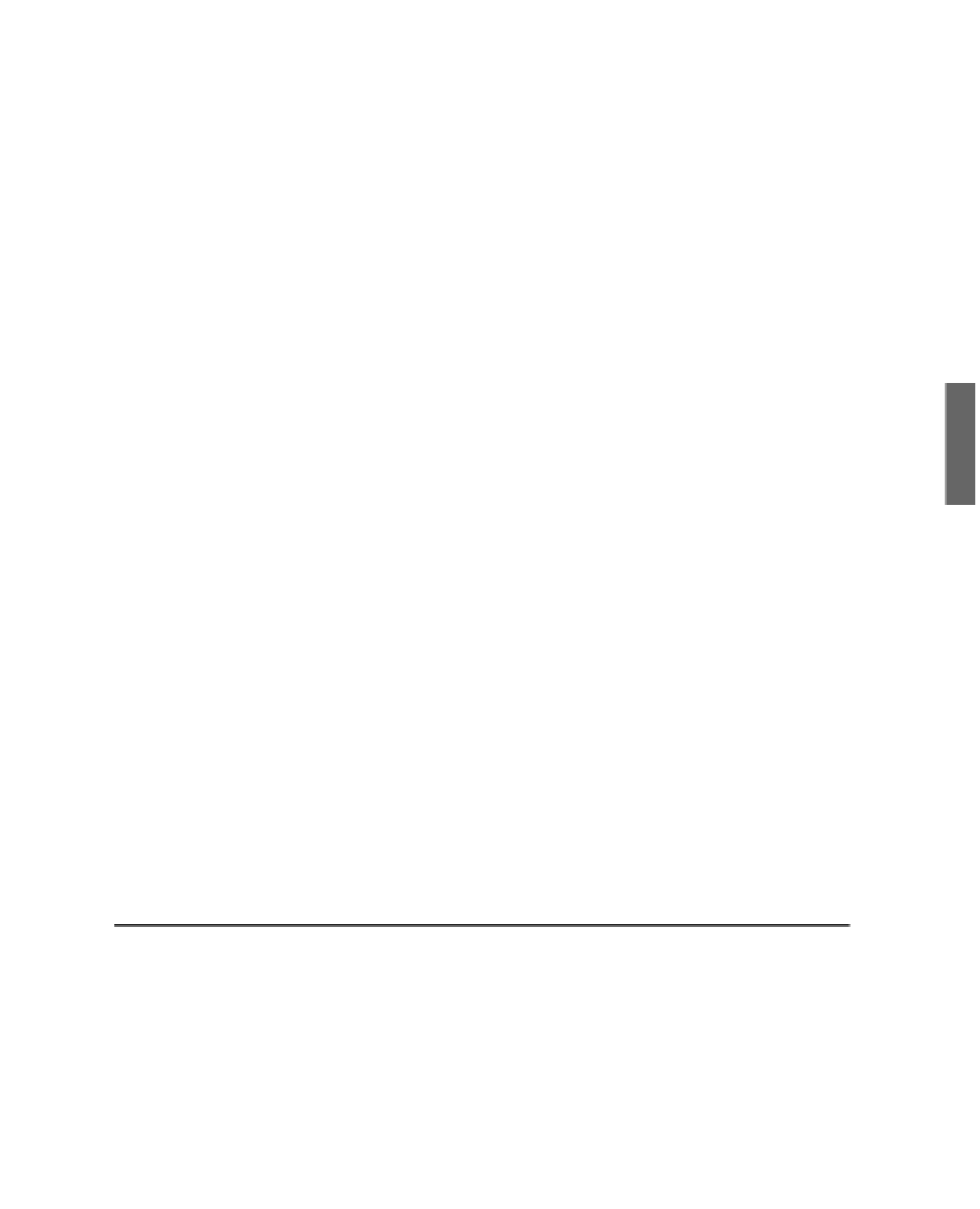