Java Reference
In-Depth Information
Incrementing and Decrementing
Another common task required in programming is to add or subtract 1 from an integer
variable. There are special operators for these expressions, which are called increment
and decrement operations.
Incrementing
a variable means to add 1 to its value, and
decrementing
a variable means to subtract 1 from its value.
The increment operator is
++
, and the decrement operator is
--
. These operators are
placed immediately after or immediately before a variable name, as in the following code
example:
int x = 7;
x = x++;
In this example, the statement
x = x++
increments the
x
variable from 7 to 8.
These increment and decrement operators can be placed before or after a variable name,
and this affects the value of expressions that involve these operators.
Increment and decrement operators are called
prefix
operators if listed before a variable
name and
postfix
operators if listed after a name.
In a simple expression such as
standards--;
, using a prefix or postfix operator produces
the same result, making the operators interchangeable. When increment and decrement
operations are part of a larger expression, however, the choice between prefix and postfix
operators is important.
Consider the following code:
int x, y, z;
x = 42;
y = x++;
z = ++x;
The three expressions in this code yield very different results because of the difference
between prefix and postfix operations.
When you use postfix operators, as in
y = x++
,
y
receives the value of
x
before it is
incremented by one. When using prefix operators, as in
z = ++x
,
x
is incremented by
one before the value is assigned to
z
. The end result of this example is that
y
equals
42
,
z
equals
44
, and
x
equals
44
.
If you're still having some trouble figuring this out, here's the example again with com-
ments describing each step:
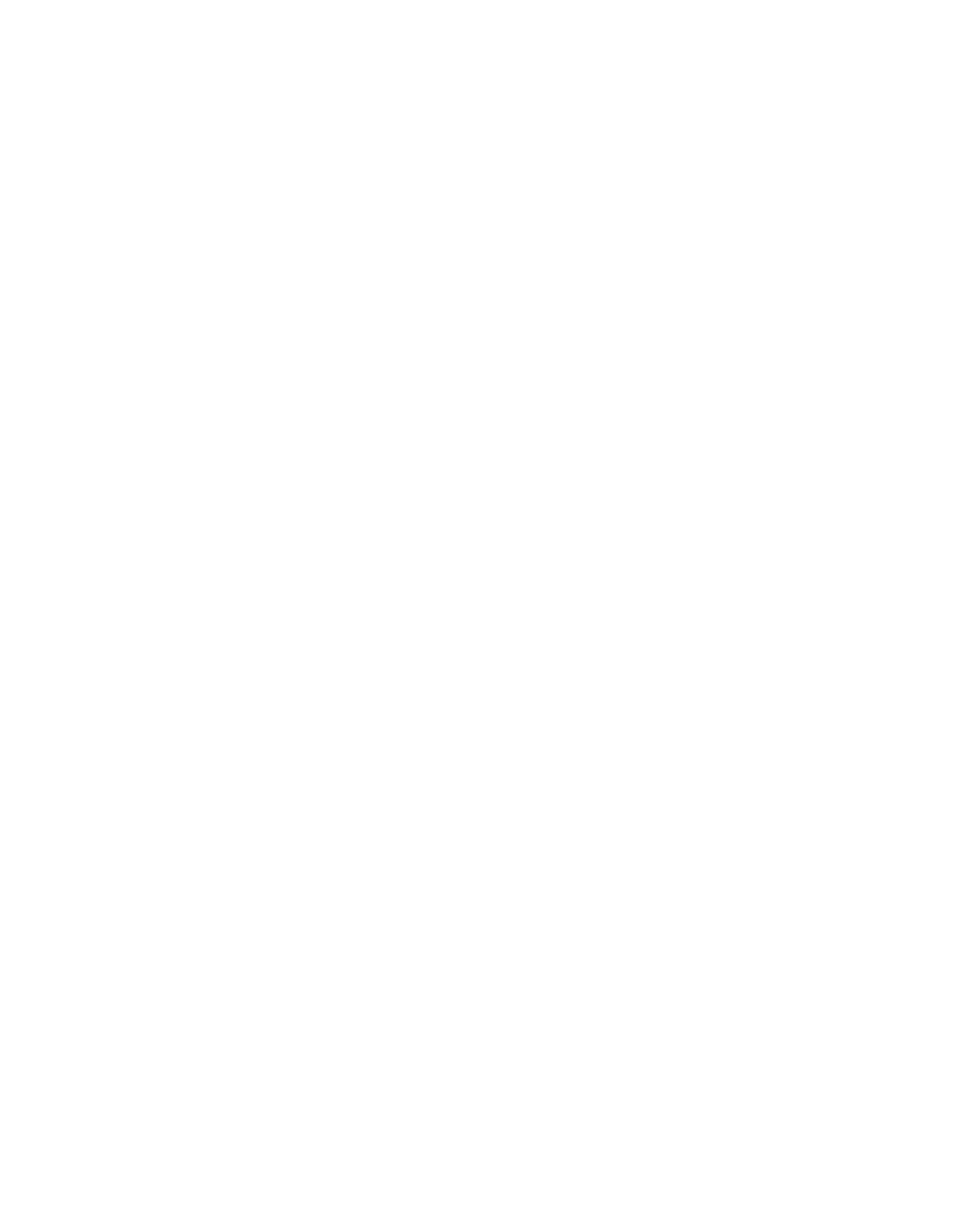