Java Reference
In-Depth Information
To give you a more complete picture of how these classes work together, the day's final
project is
NewFingerServer
, a web application that uses a nonblocking socket channel to
handle finger requests.
Enter the text of Listing 17.5, save it as
NewFingerServer.java
, and compile the appli-
cation.
LISTING 17.5
The Full Text of
NewFingerServer.java
1: import java.io.*;
2: import java.net.*;
3: import java.nio.*;
4: import java.nio.channels.*;
5: import java.util.*;
6:
7: public class NewFingerServer {
8:
9: public NewFingerServer() {
10: try {
11: // Create a nonblocking server socket channel
12: ServerSocketChannel sockChannel = ServerSocketChannel.open();
13: sockChannel.configureBlocking(false);
14:
15: // Set the host and port to monitor
16: InetSocketAddress server = new InetSocketAddress(
17: “localhost”, 79);
18: ServerSocket socket = sockChannel.socket();
19: socket.bind(server);
20:
21: // Create the selector and register it on the channel
22: Selector selector = Selector.open();
23: sockChannel.register (selector, SelectionKey.OP_ACCEPT);
24:
25: // Loop forever, looking for client connections
26: while (true) {
27: // Wait for a connection
28: selector.select();
29:
30: // Get list of selection keys with pending events
31: Set keys = selector.selectedKeys();
32: Iterator it = keys.iterator();
33:
34: // Handle each key
35: while (it.hasNext()) {
36:
37: // Get the key and remove it from the iteration
38: SelectionKey selKey = (SelectionKey) it.next();
39:
40: it.remove();
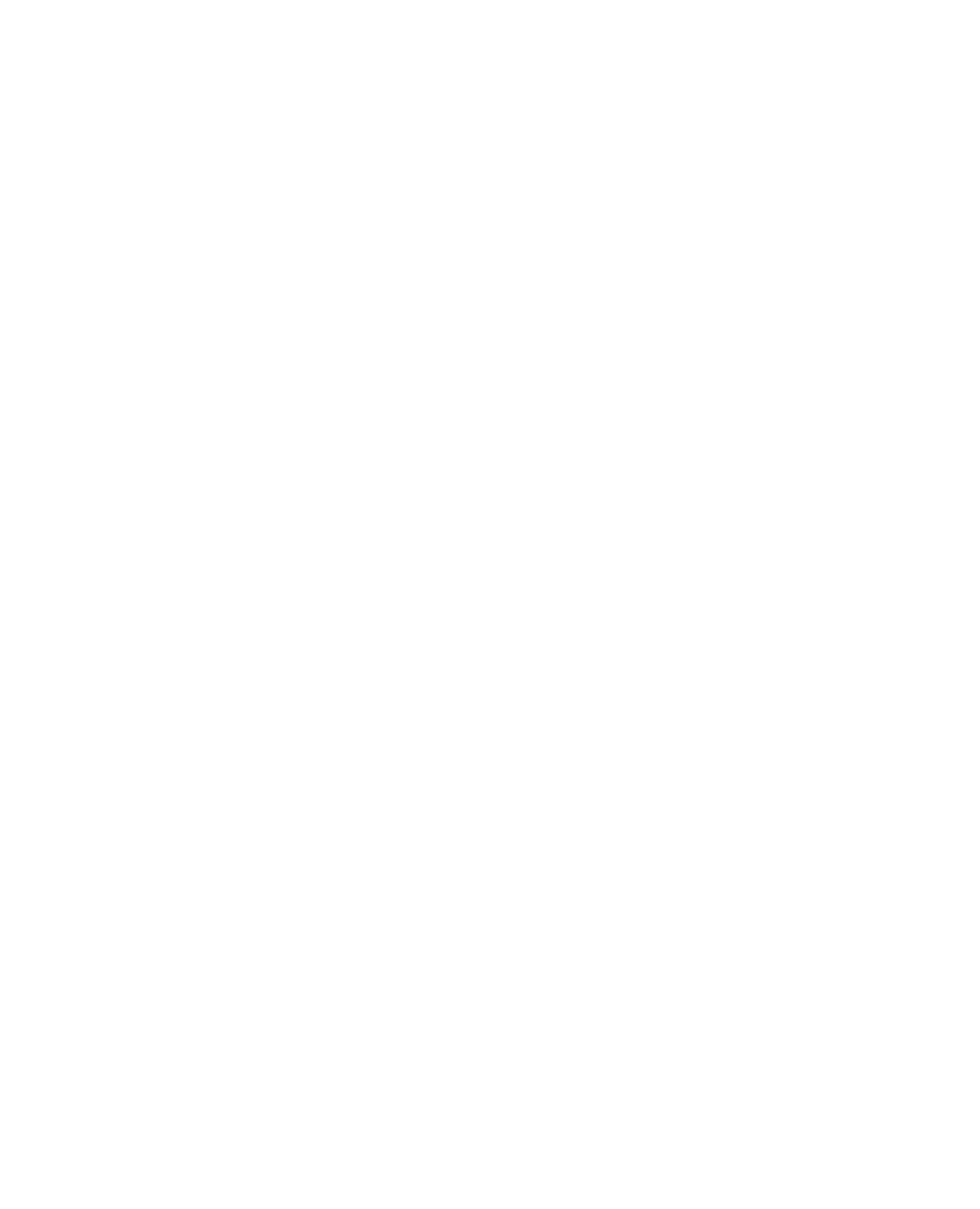