Java Reference
In-Depth Information
The next project, the
BufferDemo
application, writes a series of bytes to a buffered output
stream associated with a text file. The first and last integers in the series are specified as
two arguments, as in the following JDK command:
java BufferDemo 7 64
15
After writing to the text file,
BufferDemo
creates a buffered input stream from the file
and reads the bytes back in. Listing 15.3 contains the source code.
LISTING 15.3
The Full Text of
BufferDemo.java
1: import java.io.*;
2:
3: public class BufferDemo {
4: public static void main(String[] arguments) {
5: int start = 0;
6: int finish = 255;
7: if (arguments.length > 1) {
8: start = Integer.parseInt(arguments[0]);
9: finish = Integer.parseInt(arguments[1]);
10: } else if (arguments.length > 0)
11: start = Integer.parseInt(arguments[0]);
12: ArgStream as = new ArgStream(start, finish);
13: System.out.println(“\nWriting: “);
14: boolean success = as.writeStream();
15: System.out.println(“\nReading: “);
16: boolean readSuccess = as.readStream();
17: }
18: }
19:
20: class ArgStream {
21: int start = 0;
22: int finish = 255;
23:
24: ArgStream(int st, int fin) {
25: start = st;
26: finish = fin;
27: }
28:
29: boolean writeStream() {
30: try {
31: FileOutputStream file = new
32: FileOutputStream(“numbers.dat”);
33: BufferedOutputStream buff = new
34: BufferedOutputStream(file);
35: for (int out = start; out <= finish; out++) {
36: buff.write(out);
37: System.out.print(“ “ + out);
38: }

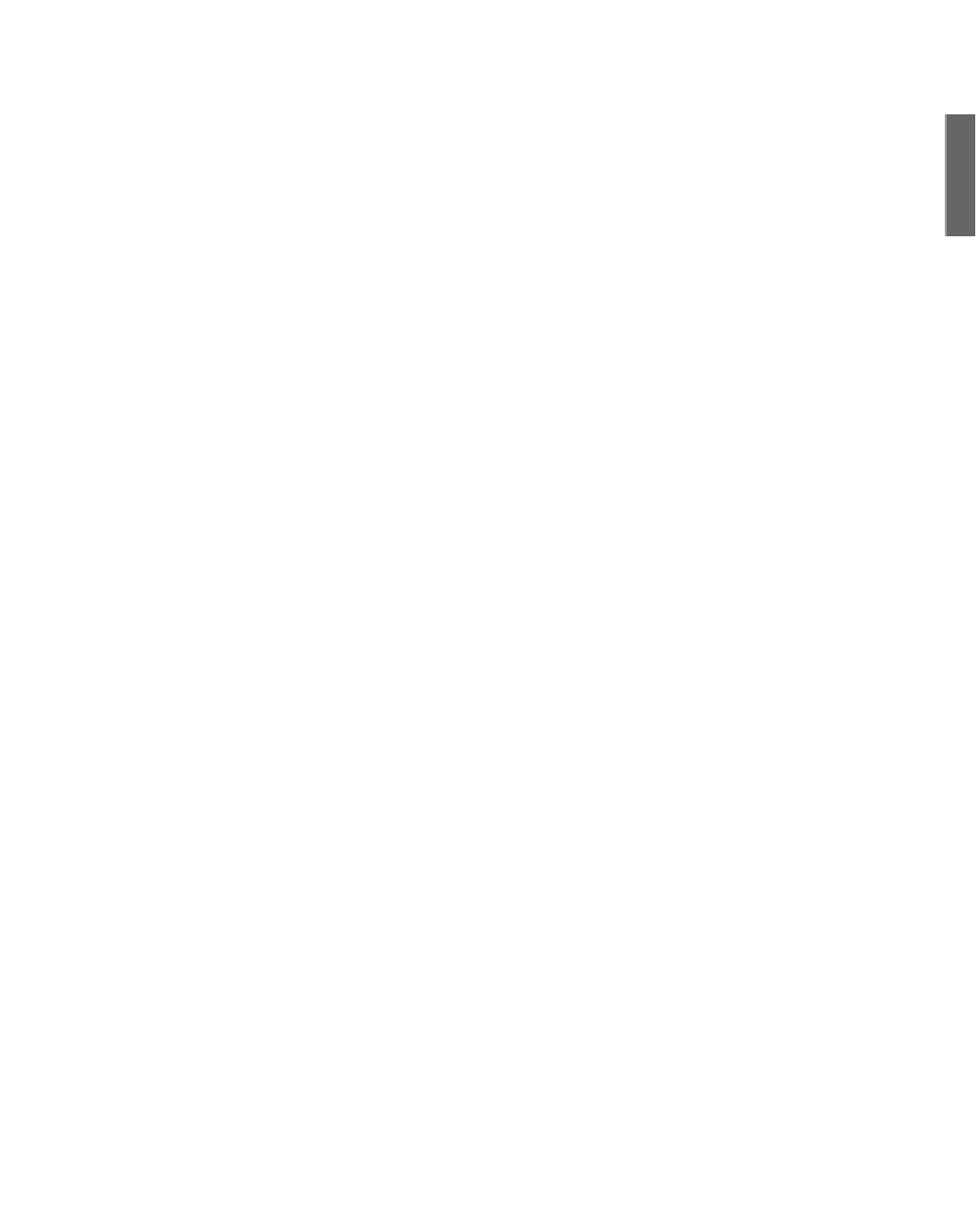