Java Reference
In-Depth Information
The
contains()
method checks whether an object is stored in the hash table. This
method searches for an object value in the hash table instead of searching for a key. The
following code shows how to use the
contains()
method:
boolean isThere = hash.contains(new Rectangle(0, 0, 5, 5));
Similar to
contains()
, the
containsKey()
method searches a hash table but is based on
a key rather than a value:
boolean isThere = hash.containsKey(“Small”);
As mentioned earlier, a hash table rehashes itself when it determines that it must increase
its capacity. You can force a rehash yourself by calling the
rehash()
method:
hash.rehash();
The practical use of a hash table comes from its capability to represent data that are too
time-consuming to search or reference by value. The data structure comes in handy when
you're working with complex data and it's more efficient to access the data by using a
key rather than comparing the data objects themselves.
Furthermore, hash tables typically compute a key for elements, which is called a
hash
code
. For example, a string can have an integer hash code computed for it that uniquely
represents the string. When a bunch of strings are stored in a hash table, the table can
access the strings by using integer hash codes as opposed to using the contents of the
strings themselves. This results in much more efficient searching and retrieving capabili-
ties.
A
hash code
is a computed key that uniquely identifies each element in a hash table.
This technique of computing and using hash codes for object storage and reference is
exploited heavily throughout the Java class library. The parent of all classes,
Object
,
defines a
hashCode()
method overridden in most standard Java classes. Any class that
defines a
hashCode()
method can be efficiently stored and accessed in a hash table. A
class that wants to be hashed also must implement the
equals()
method, which defines a
way of telling whether two objects are equal. The
equals()
method usually just per-
forms a straight comparison of all the member variables defined in a class.
The next project you undertake today uses tables for a shopping application.
The
ComicBooks
application prices collectible comic topics according to their base value
and their condition. The condition is described as one of the following: mint, near mint,
very fine, fine, good, or poor.
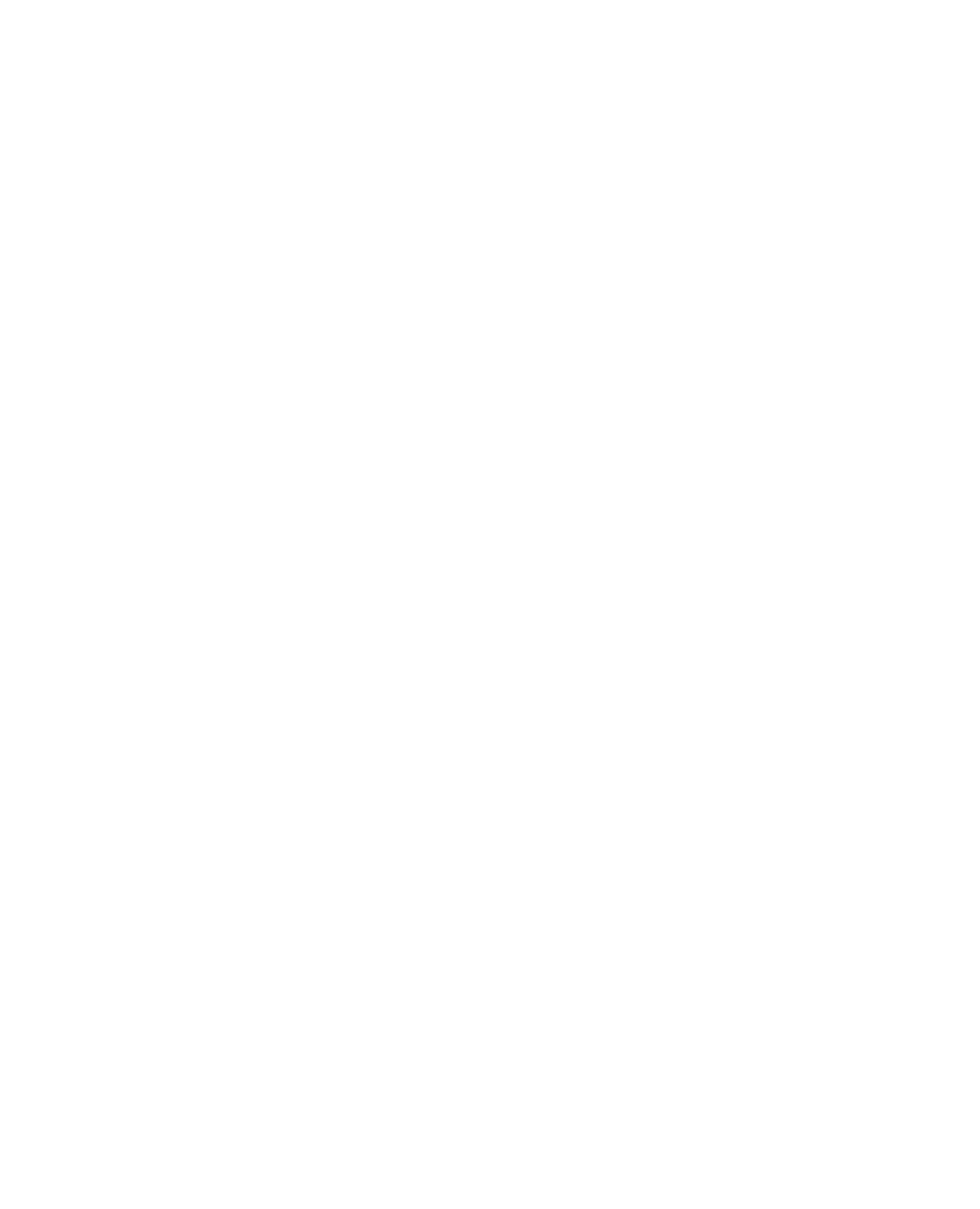