Java Reference
In-Depth Information
LISTING 8.2
Continued
31: CodeKeeper keeper = new CodeKeeper(arguments);
32: }
33: }
8
This class compiles successfully, but there's an ominous warning that it uses “
unchecked
or unsafe operations.”
This isn't as severe as it sounds—the code works properly as
written and is not unsafe.
The warning serves as a not-so-subtle hint that there's a better way to work with vectors
and other data structures. You learn about this technique later today.
The
CodeKeeper
class uses a
Vector
instance variable named
list
to hold the text codes.
First, five built-in codes are read from a string array into the vector (lines 10-12).
Next, any codes provided by the user as command-line arguments are added (lines
14-16).
Codes are added by calling the
addCode()
method (lines 24-28), which only adds a new
text code if it isn't already present, using the vector's
contains(
Object
)
method to
make this determination.
After the codes have been added to the vector, its contents are displayed. Running the
class with the command-line arguments “gamma”, “beta”, and “delta” produces the fol-
lowing output:
alpha
lambda
gamma
delta
zeta
beta
A
for
loop can be employed to iterate through a data structure. The loop takes the form
for (
variable
:
structure
)
. The
structure
is a data structure that implements the
Iterator
interface. The
variable
section declares an object that holds each element of
the structure as the loop progresses.
This new
for
loop uses an iterator and its methods to traverse an entire vector named
list
:
for (Object name : list) {
System.out.println(name);
}
The new loop can be used with any data structure that works with
Iterator
.

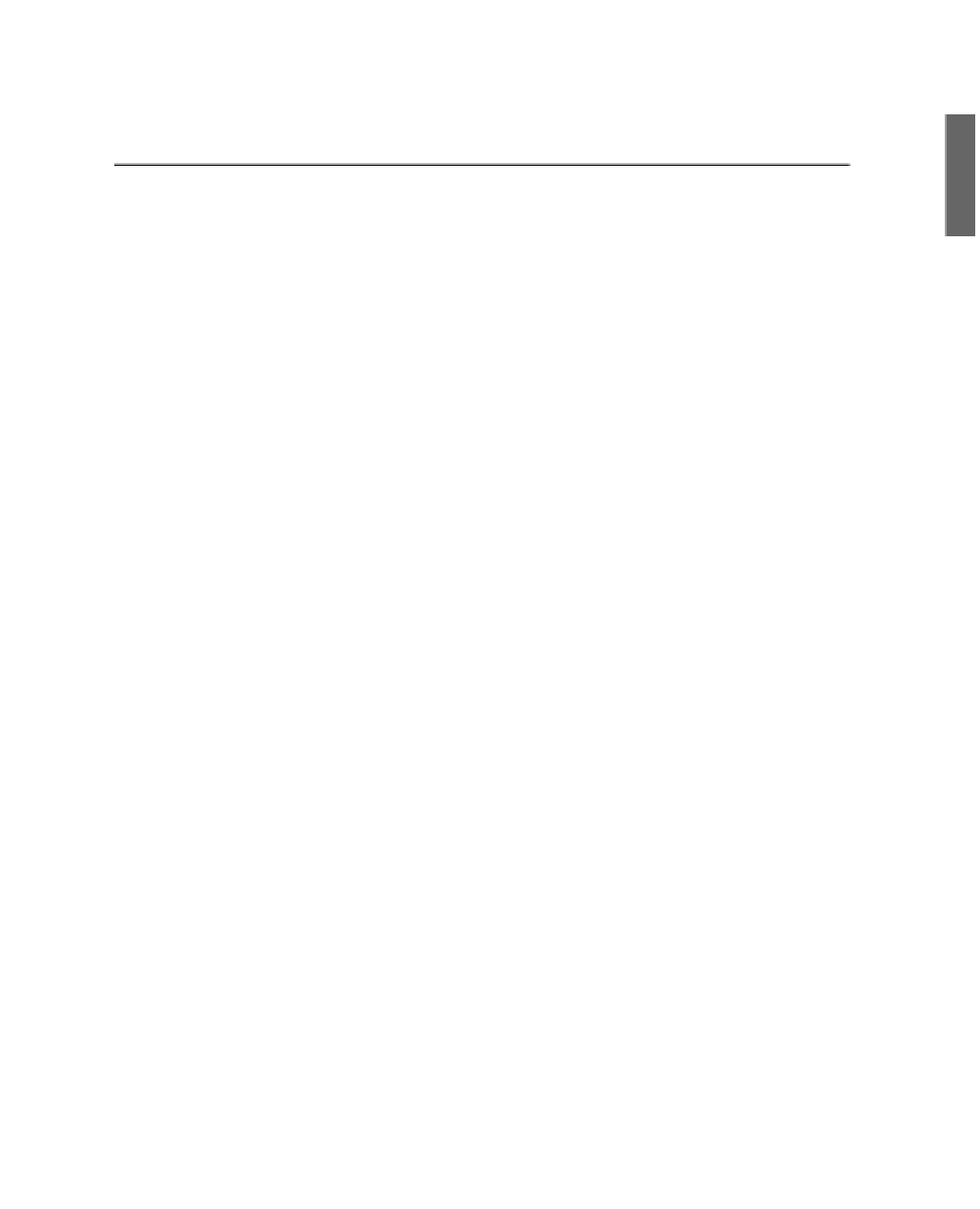