Java Reference
In-Depth Information
A class that contains
assert
statements can be compiled normally, as long as you're
using a current version of the JDK.
The compiler includes support for assertions in the class file (or files) that it produces,
but the feature must be turned on.
There are several ways to turn on assertions in the JDK's Java interpreter.
To enable assertions in all classes except those in the Java class library, use the
-ea
argu-
ment, as in this example:
java -ea PriceChecker
To enable assertions only in one class, follow
-ea
with a colon (“:”) and the name of the
class, like this:
java -ea:PriceChecker PriceChecker
You also can enable assertions for a specific package by following
-ea:
with the name of
the package (or “...” for the default package).
There's also an
-esa
flag that enables assertions in the Java class
library. There isn't much reason for you to do this because you're
probably not testing the reliability of that code.
TIP
When a class that contains assertions is run without an
-ea
or
-esa
flag, all
assert
state-
ments will be ignored.
Because Java has added the
assert
keyword, you must not use it as the name of a vari-
able in your programs, even if they are not compiled with support for assertions enabled.
The next project,
CalorieCounter
, is a calculator application that makes use of an asser-
tion. Listing 7.2 contains the source.
LISTING 7.2
The full source of
CalorieCounter.java
1: public class CalorieCounter {
2: float count;
3:
4: public CalorieCounter(float calories, float fat, float fiber) {
5: if (fiber > 4) {
6: fiber = 4;
7: }
8: count = (calories / 50) + (fat / 12) - (fiber / 5);
9: assert count > 0 : “Adjusted calories < 0”;
7

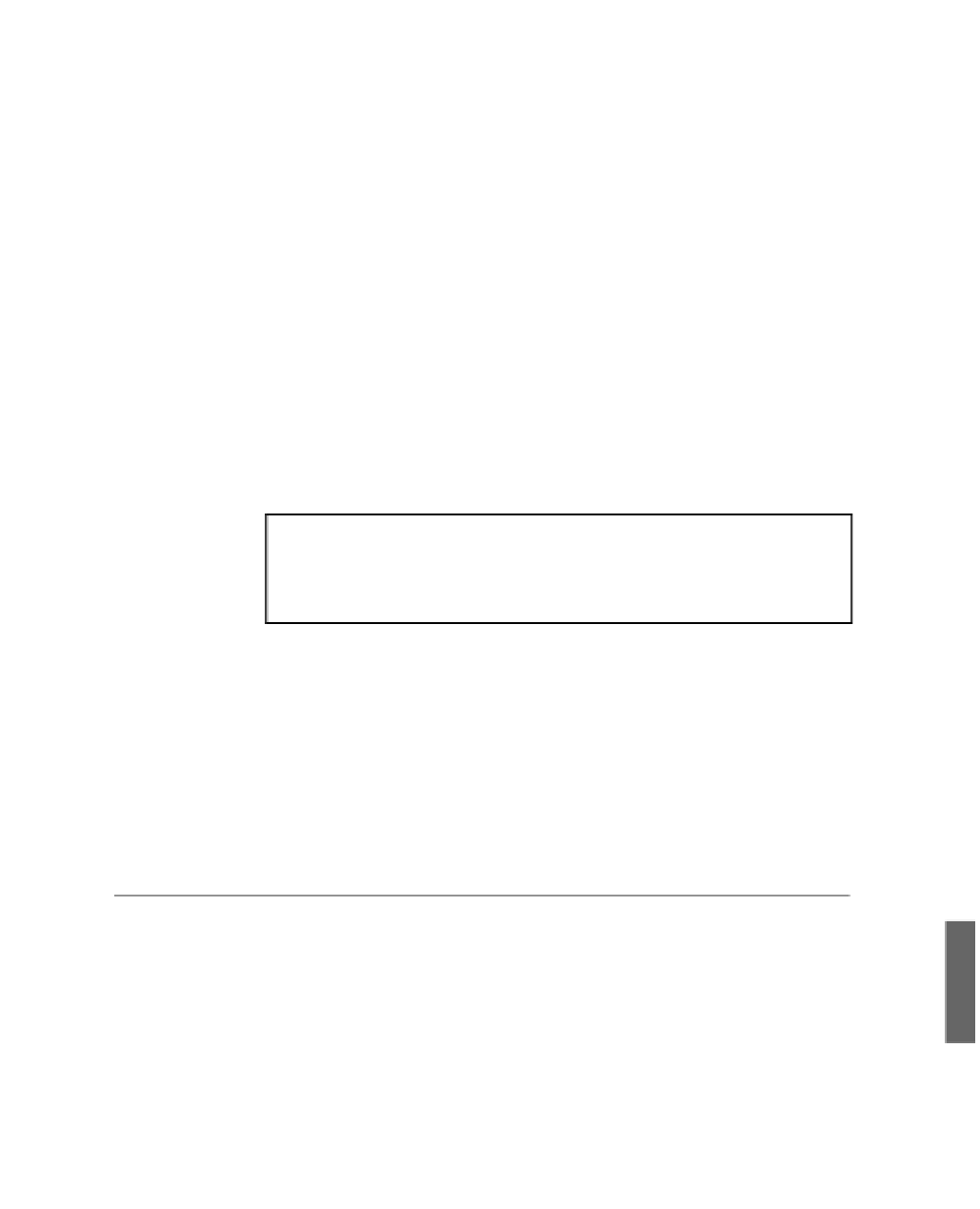