Java Reference
In-Depth Information
Note that as with
catch
, you can use a superclass of a group of exception to indicate that
your method might throw any subclass of that exception. For instance:
public void loadFormula() throws IOException {
// ...
}
Keep in mind that adding a
throws
clause to your method definition simply means that
the method might throw an exception if something goes wrong, not that it actually will.
The
throws
clause provides extra information to your method definition about potential
exceptions and allows Java to make sure that your method is being used correctly by
other people.
Think of a method's overall description as a contract between the designer of that
method and the caller of the method. (You can be on either side of that contract, of
course.)
Usually the description indicates the types of a method's arguments, what it returns, and
the particulars of what it normally does. By using
throws
, you are adding information
about the abnormal things the method can do. This new part of the contract helps sepa-
rate and make explicit all the places where exceptional conditions should be handled in
your program, and that makes large-scale design easier.
Which Exceptions Should You Throw?
After you decide to declare that your method might throw an exception, you must decide
which exceptions it might throw and actually throw them or call a method that will throw
them (you learn about throwing your own exceptions in the next section).
In many instances, this is apparent from the operation of the method itself. Perhaps
you're already creating and throwing your own exceptions, in which case, you'll know
exactly which exceptions to throw.
You don't really have to list all the possible exceptions that your method could throw;
some exceptions are handled by the runtime itself and are so common that you don't
have to deal with them.
In particular, exceptions of either the
Error
or
RuntimeException
class or any of their
subclasses do not have to be listed in your
throws
clause.
They get special treatment because they can occur anywhere within a Java program and
are usually conditions that you, as the programmer, did not directly cause.
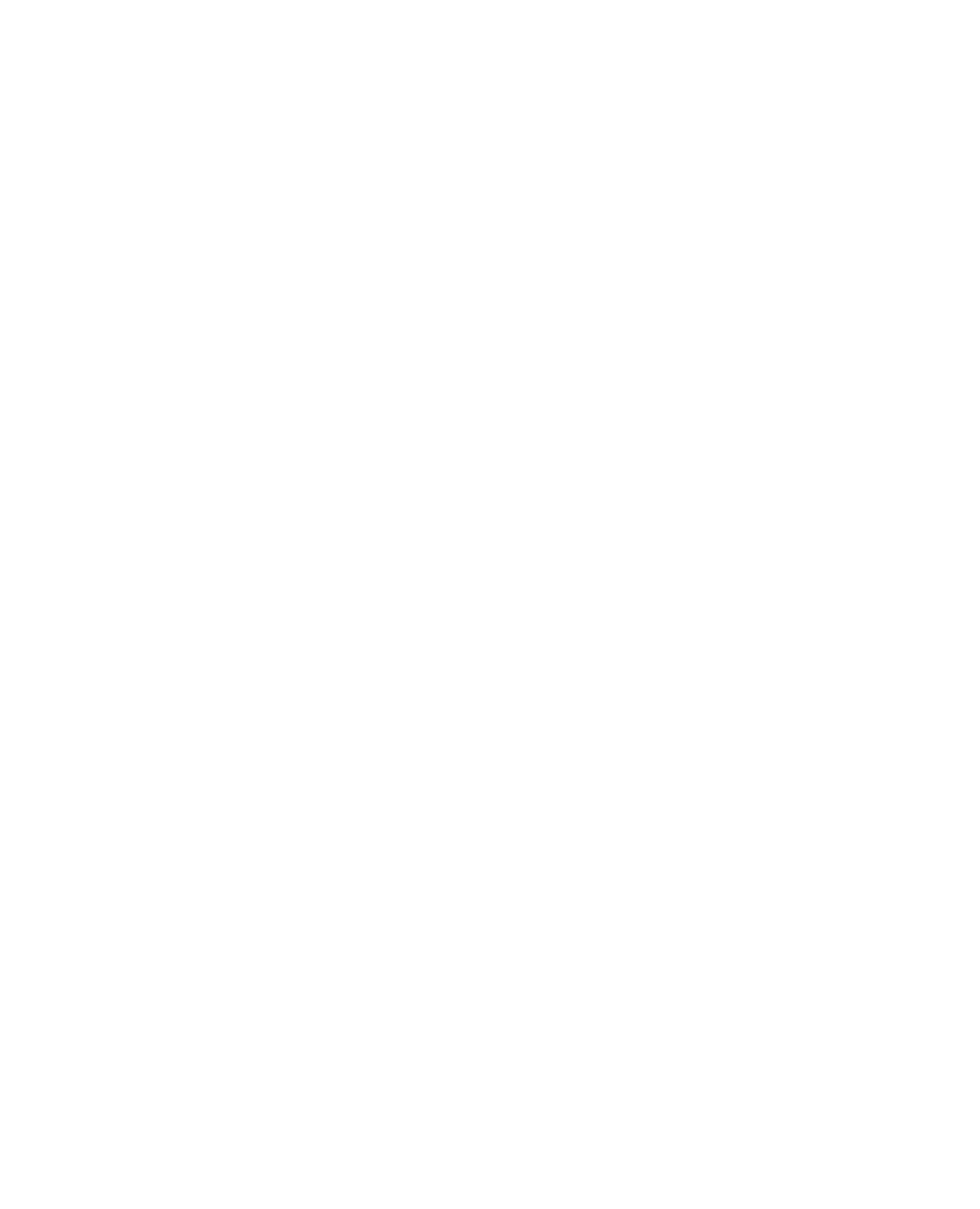