Java Reference
In-Depth Information
You've seen
try
and
catch
before, when you first dealt with threads. On Day 6,
“Packages, Interfaces, and Other Class Features,” you used code when using a
String
value to create an integer:
try {
float in = Float.parseFloat(input);
} catch (NumberFormatException nfe) {
System.out.println(input + “ is not a valid number.”);
}
Here's what's happening in these statements: The
Float.parseFloat()
class method
could potentially throw an exception of the class
NumberFormatException
, which signi-
fies that the thread has been interrupted for some reason.
To handle this exception, the call to
parseFloat()
is placed inside a
try
block, and an
associated
catch
block has been set up. This
catch
block receives any
NumberFormatException
objects thrown within the
try
block.
The part of the
catch
clause inside the parentheses is similar to a method definition's
argument list. It contains the class of exception to be caught and a variable name. You
can use the variable to refer to that exception object inside the
catch
block.
One common use for this object is to call its
getMessage()
method. This method is pre-
sent in all exceptions, and it displays a detailed error message describing what happened.
Another useful method is
printStackTrace()
, which displays the sequence of method
calls that led to the statement that generated the exception.
The following example is a revised version of the
try
-
catch
block used on Day 6:
try {
float in = Float.parseFloat(input);
} catch (NumberFormatException nfe) {
System.out.println(“Oops: “ + nfe.getMessage());
}
The examples you have seen thus far catch a specific type of exception. Because excep-
tion classes are organized into a hierarchy and you can use a subclass anywhere that
a superclass is expected, you can catch groups of exceptions within the same
catch
statement.
7
As an example, when you start writing programs that handle input and output from files,
Internet servers, and other places, you deal with several different types of
IOException

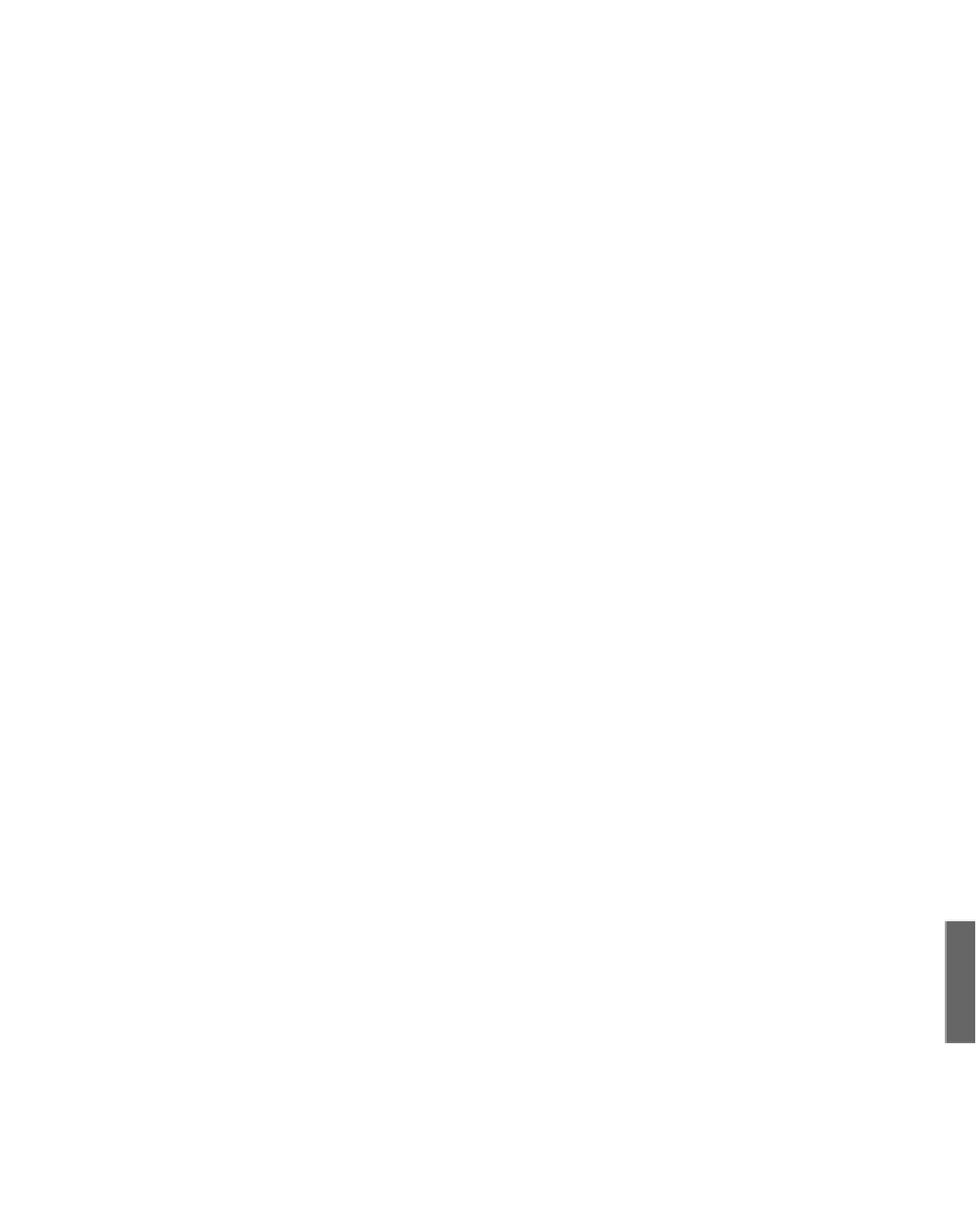