Java Reference
In-Depth Information
Implementing and Using Interfaces
You can do two things with interfaces: Use them in your own classes and define your
own. For now, start with using them in your own classes.
To use an interface, include the
implements
keyword as part of your class definition:
public class AnimatedSign extends javax.swing.JApplet
implements Runnable {
//...
}
In this example,
javax.swing.JApplet
is the superclass, but the
Runnable
interface
extends the behavior that it implements.
Because interfaces provide nothing but abstract method definitions, you then have to
implement those methods in your own classes using the same method signatures from the
interface.
To implement an interface, you must offer all the methods in that interface—you can't
pick and choose the methods you need. By implementing an interface, you're telling
users of your class that you support the entire interface.
After your class implements an interface, subclasses of your class inherit those new
methods and can override or overload them. If your class inherits from a superclass that
implements a given interface, you don't have to include the
implements
keyword in your
own class definition.
Implementing Multiple Interfaces
Unlike with the singly inherited class hierarchy, you can include as many interfaces as
you need in your own classes. Your class will implement the combined behavior of all
the included interfaces. To include multiple interfaces in a class, just separate their names
with commas:
public class AnimatedSign extends javax.swing.JApplet
implements Runnable, Observable {
6
// ...
}
Note that complications might arise from implementing multiple interfaces. What hap-
pens if two different interfaces both define the same method? You can solve this problem
in three ways:
If the methods in each of the interfaces have identical signatures, you implement
one method in your class, and that definition satisfies both interfaces.
n

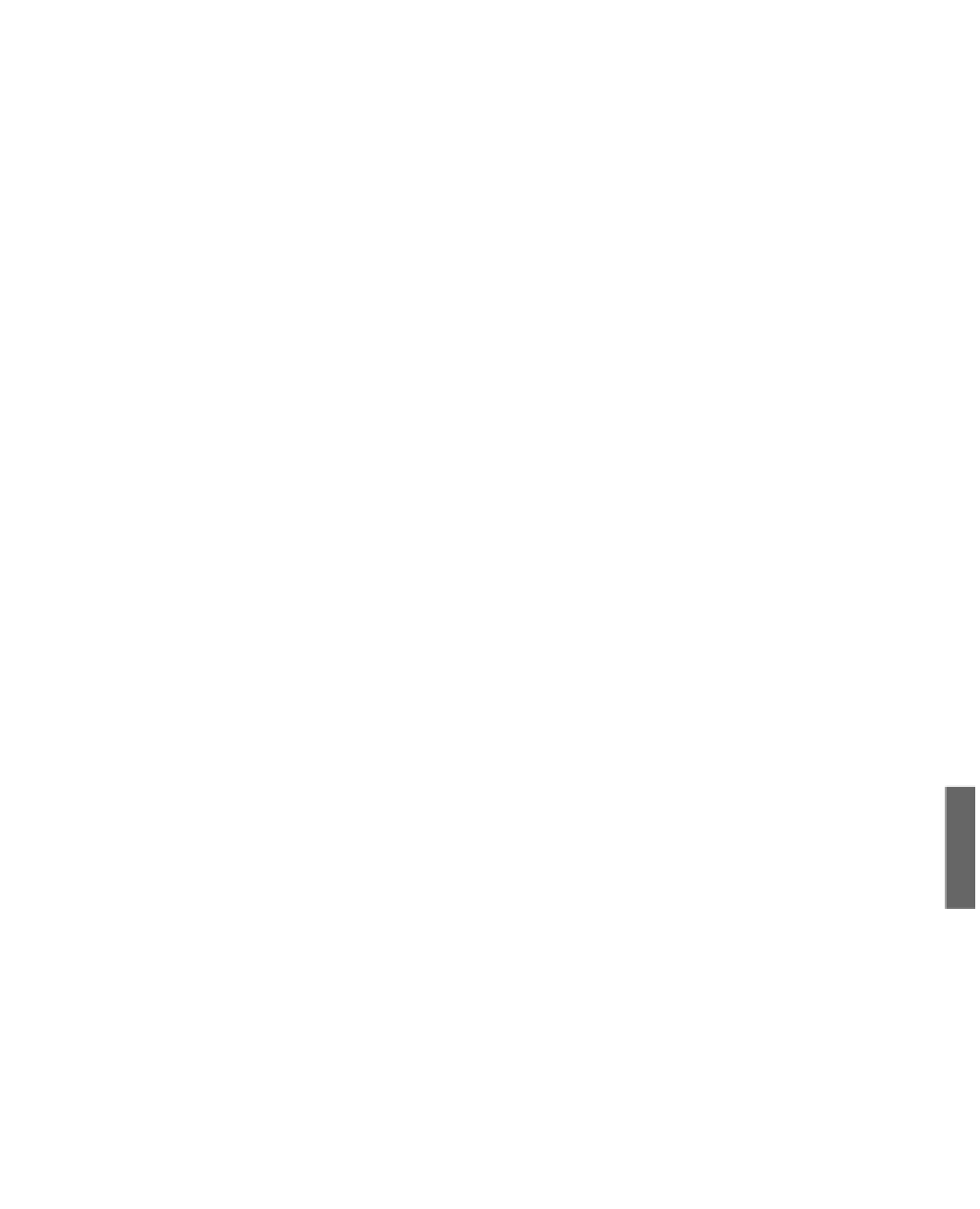